When we work on complex projects or tasks, it can be difficult to open up multiple terminals to run different scripts. Being able to run multiple npm scripts simultaneously can improve the efficiency of development. In this article, we’ll look at three ways in which we can run all our npm scripts in parallel with only a few commands and all in a single terminal!
Methods to Run Multiple NPM Scripts
NPM (Node Package Manager) may not provide us with a straightforward method to achieve this but there are a few workarounds we can use to go about doing this. Some of these methods are as follows:
- Using the concurrently package
- Using Bash’s & and && operators
- Using npm-run-all
Let’s look at each of these methods in detail with some examples.
1. Using the concurrently package
The concurrently package provided by npm allows us to run multiple npm scripts parallelly. This package can be installed globally or locally, depending on whether you want to access it throughout your system or locally if you only want to access it in a specific project.
Here, we’ll first look at both ways of installation:
- Install concurrently globally:
npm install -g concurrently
- Install concurrently locally:
npm i -D concurrently
We have used -D above to add the package to devDependencies instead of dependencies in the package.json file as it is recommended to do so.
Now, we add the scripts that we want to run parallelly into the scripts object in the package.json file. The scripts object contains commands that can be run in the terminal.
Example:
"scripts": {
"script1": "echo 'Running 1st command'",
"script2": "echo 'Running 2nd command'",
"script3": "echo 'Running 3rd command'",
"start": "concurrently \"npm run script1\" \"npm run script2\" \"npm run script3\""
}
Here, we use echo to print a string onto the terminal to indicate which script is running
Finally, we use npm start to run these scripts.
Output:
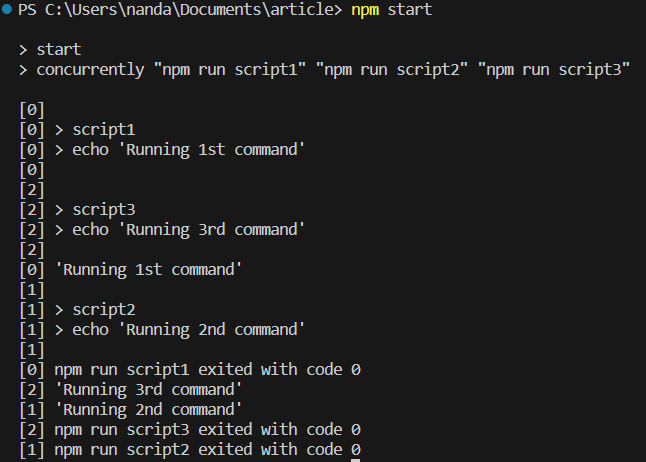
As we can see from the output, the scripts may run in any random order.
2. Using Bash’s & and && operators
The & and && operators are used to chain commands that can be run in Linux, macOS, or Bash terminals. However, there is a slight difference between & and &&, let’s look at what happens when either of these operators are used to chain commands.
Using the & operator to chain commands
In terms of shell scripting, the & operator is known as the background operator. When this operator is used between two commands, the first command is run, and simultaneously the second command is run without waiting for the first command to finish. In other words, it’s used to parallelly execute commands.
One thing to note about using & is, if the first command fails to finish execution, the second command still continues its execution, meaning, the commands are executed independently.
Example:
Let us add the following scripts to the package.json file, by changing only the start script from the previous example.
"scripts": {
"script1": "echo 'Running 1st command'",
"script2": "echo 'Running 2nd command'",
"script3": "echo 'Running 3rd command'",
"start": "npm run script1 & npm run script2 & npm run script3"
}
Output:
We use npm start to run these scripts
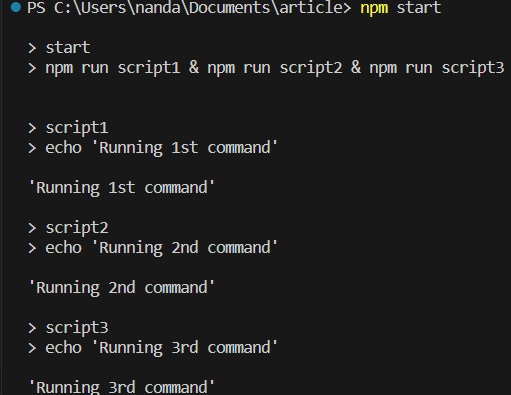
Here, we can see that the commands are run sequentially.
Using the && operator to chain commands
The && operators are referred to as AND in shell scripting and are another method used to chain commands and run multiple commands in a single terminal. When this operator is used between two commands, the first command is executed first and after it finishes execution, only then does the second command starts execution.
In the event that the first command doesn’t execute the succeeding commands are not executed as well.
Example:
Let us run the same scripts from the previous example, as all commands will execute without any error, we receive the same output as the previous example which uses the & operator.
"scripts": {
"script1": "echo 'Running 1st command'",
"script2": "echo 'Running 2nd command'",
"script3": "echo 'Running 3rd command'",
"start": "npm run script1 && npm run script2 && npm run script3"
}
Output:
The scripts can be run using the npm start command
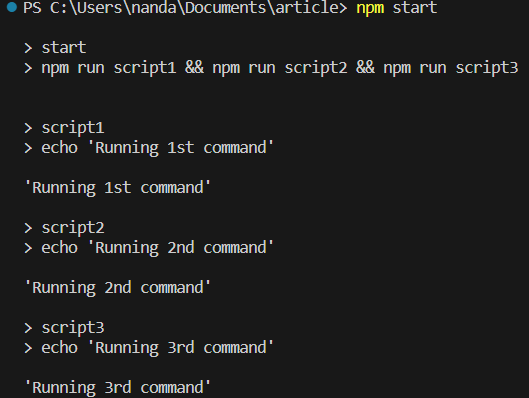
3. Using npm-run-all
The npm-run-all is a package that allows us to run multiple scripts in parallel in the terminal. This package also runs the commands sequentially. npm-run-all can be installed globally or locally depending on our requirements.
Installing npm-run-all globally:
npm install -g npm-run-all
Installing npm-run-all locally:
npm install --save-dev npm-run-all
we use –save-dev to install the package into devDependencies in the package.json file.
Now we add a script with all our commands into the package.json file which can be run using the command npm-run-all command.
Example:
"scripts": {
"script1": "echo 'Running 1st command'",
"script2": "echo 'Running 2nd command'",
"script3": "echo 'Running 3rd command'"
}
Output:
We can run the above script using the following command
npm-run-all script1 script2 script3
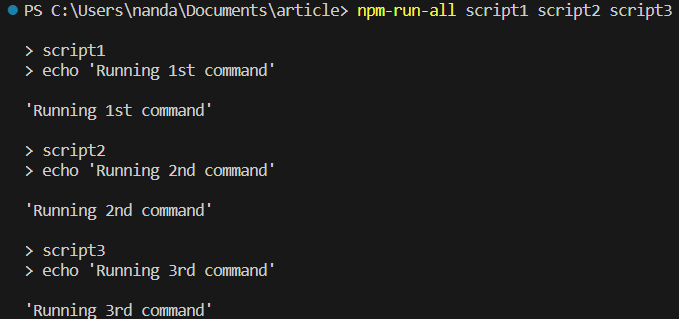
Conclusion
Running npm scripts in parallel can help us increase the efficiency and time of development. We have learned how we can use simple commands to run everything in a single terminal, without having to open up multiple terminals and run many different commands. In this article, we looked at 3 ways in which we can achieve parallel execution of npm scripts using the concurrently package, using the & and && operators, and using npm-run-all. These methods run all our scripts in the background without us having to worry about them.
Reference
https://stackoverflow.com/questions/30950032/how-can-i-run-multiple-npm-scripts-in-parallel