Executing JavaScript Files in Node.js is the core and important part of utilising the strength and adaptability of JavaScript programming. Node.js is the open-source back-end runtime environment for JavaScript applications. It has an extensive ecosystem of libraries and tools accessible via the Node Package Manager (NPM) to provide different functionalities. With Node.js it is easy to create JavaScript executable code that runs outside of the web browsers on the server.
Running JS Scripts Through the Terminal
Below is the step-by-step process to run a JavaScript script through the terminal.
1. Install Node.js
You can execute JavaScript code outside of a web browser by using the Node.js. Firstly, you must download the installer from the official Node.js website (https://nodejs.org/) and install Node.js by following the operating system’s installation instructions.
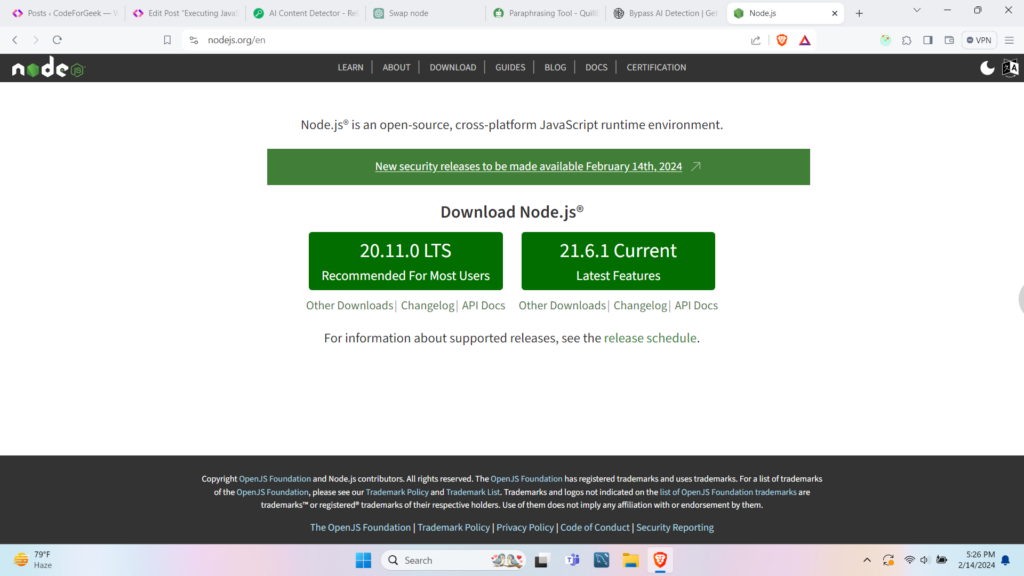
Verify Node.js Installation
To verify your Node.js is installed, open Terminal (or Command Prompt), then type “node -v” and press Enter. This command will display the version of Node.js installed on your system.
node -v
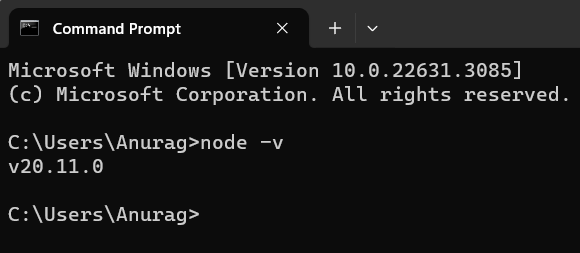
2. Create a JavaScript File
Using an integrated programming environment (IDE) or text editor, create a new file with a .js extension. The JavaScript code that you wish to execute using Node.js is contained in this file.
const add = (a, b) => {
return a + b
}
console.log(add(5, 5))
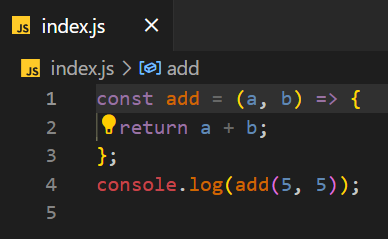
3. Open the Command Line Interface and Navigate to the Directory
You can open Command Prompt by searching for “cmd” in the start menu and then pressing Enter. Once the command prompt is open, browse to the directory containing your JavaScript file by using the “cd” command.
The command “cd”, which stands for “change directory”, is used to modify the current working directory in terminal shells and command-line interfaces (CLI).
cd [directory_path]
Here [directory path] is the path of the directory you want to navigate to.
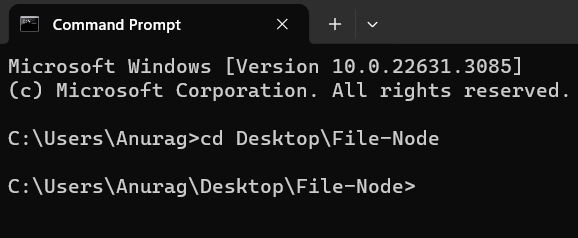
Step 4: Execute the JavaScript File
Once you have navigated to the desired directory in the command-line interface, you can execute a JavaScript file using Node.js. We will use the “node” command followed by the name of your JavaScript file.
Assuming your JavaScript file is named “index.js”, you would simply type the command “node index.js” and press Enter.
node index.js
Here
- node is the command used to execute JavaScript files with Node.js.
- index.js is the name of your JavaScript file. Replace it with the actual filename if it’s different.
This command runs the JavaScript file using Node.js, executing the code contained within it and displaying any output or errors in the command-line interface.
Output
The output of your script should appear in the CLI once the JavaScript file has been executed. Any messages or outcomes produced by the JavaScript code you developed for your file might go under this category. Any problems that you may have in your code will be also shown in the CLI along with details on where they happened.
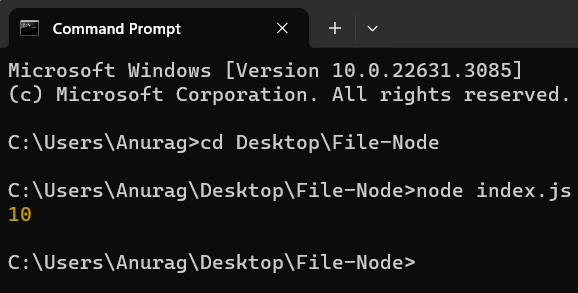
You may run a JavaScript file in a Node.js environment by following these instructions, and you can see the output or any problems that the script produces. This procedure makes use of the features of the Node.js runtime to enable you to execute JavaScript code outside of a web browser.
Conclusion
Developers may rapidly start scripts without the overhead of graphical interfaces by using the command-line interface to execute JavaScript files via the terminal. This simplifies the process for developers. This approach offers efficiency and simplicity and is especially beneficial for server-side scripting, batch processing, and automation jobs. Whereas Integrated development environments (IDE) boost productivity through the use of syntax highlighting, debugging tools, and an easier way to write and modify code. The decision between utilizing an IDE or the terminal ultimately comes down to the developer’s tastes and the particular requirements of the project.
Continue Reading:
- JSON.parse(): Convert JSON String To Object Using NodeJS
- Send a JSON response using Express Framework
Reference
https://stackoverflow.com/questions/8532960/how-do-you-run-javascript-script-through-the-terminal