Have you ever done form validation? Well with other languages this can be a complex process, one can do that by using loops to go through all the letters inside an expression to check for the required demands. Hey, but like all things, JavaScript made this process easier as well. How? Let’s see in this beginner’s friendly tutorial.
What Is a Regular Expression?
We all know how a string looks in JavaScript, right? It is a group of characters enclosed inside inverted commas(‘), but have you seen characters enclosed inside forward slashes(/)? Well, this is called a regular expression.
A regular expression works as a filter for strings. It is used to search for or validate a sequence of characters in a string. Regular expression can be very useful in systems like form validators, number checkers, etc. where we have to validate a string based on the presence of some substring.
Regular Expression Patterns and Flag
A basic regex syntax looks like this:
/pattern/flags
The pattern may have various elements:
- Literal: Matches itself in a string. Ex – “/abc/”, “/91/”, etc.
- Metacharacters: Special characters having special meaning. Ex – “.” (matches any single character), “^” (matches the beginning of a line), “$” (matches the end of a line), “*” (matches zero or more occurrences), “+” (matches one or more occurrences), etc.
- Character Classes: Allows us to match a single character from a set of characters. Ex – “[aeiou]” (matches any vowel), “[0-9]” (matches any digit), etc.
- Quantifiers: Define the number of times a character can appear. Ex – “+” (matches one or more occurrences), “?” (matches zero or one occurrence), “{n}” (matches exactly n occurrences), etc.
We can remove this ‘flags’ part if it is not necessary but they are very useful. Let’s take a look at some useful ones:
- i: For case-insensitive matching.
- g: For global matching.
- m: For multiline matching.
Example of Regular Expression
Let’s see an example of a regular expression and how it works with string.
var numberToCheck = "+911234567890";
var regex = /\+91/;
if (regex.test(numberToCheck)) {
console.log("Valid Indian phone number");
} else {
console.log("Invalid Indian phone number");
}
Here we have initialized two variables, the first is a random number to check, and the second is the regex, now we use the text() method to check for the result and based on that log different messages to the console.
In regex, we use backslash(\) as an escape character to use characters having special meaning as literals.
Let’s run the code.
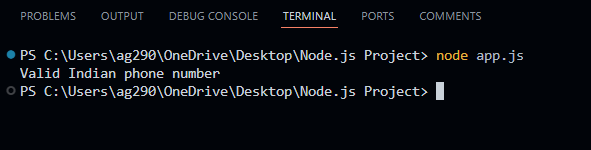
But if you notice, it validates a string if it has “+91” either in any possible but that is completely false. Suppose the user types the number “1234567890+91”:
var numberToCheck = "1234567890+91";
var regex = /\+91/;
if (regex.test(numberToCheck)) {
console.log("Valid Indian phone number");
} else {
console.log("Invalid Indian phone number");
}
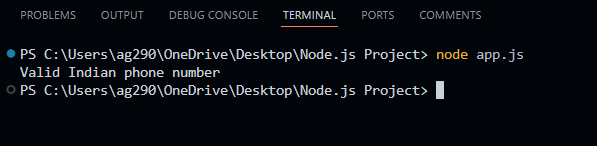
The program validates the number as a correct Indian number which is not true, so let’s modify the regex to make things work.
We can add metacharacters “^” to the regex at the beginning to match the result if the substring is present at the beginning of the string:
var numberToCheck = "1234567890+91";
var regex = /^\+91/;
if (regex.test(numberToCheck)) {
console.log("Valid Indian phone number");
} else {
console.log("Invalid Indian phone number");
}
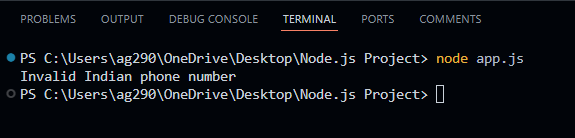
We can now modify the regex to match more precisely that the numbers after “+91” should be 10 digits.
var numberToCheck = "+911234567890";
var regex = /\+91\d{10}$/;
if (regex.test(numberToCheck)) {
console.log("Valid Indian phone number");
} else {
console.log("Invalid Indian phone number");
}
See how regular expressions are making everything easier. With just one line of expression, we can now validate any number. You can add multiple conditions using the different flags we mentioned above.
JavaScript Regular Expression Methods
Just like we have seen the regex test() methods, there are some more useful methods that we can use with regular expressions, let’s see them.
- test(): Returns true or false based on the match.
- exec(): Returns an array of information if the match is found otherwise null.
- match(): Returns an array containing all the matches if the match is found otherwise null.
- matchAll(): Returns an iterator containing all of the matches.
- search(): Returns the index of the match if the match is found otherwise -1.
- replace(): Replaces the matched substring with the given substring.
- split(): Split a string into an array of substrings using a regular expression.
Did You Know You Can Remove All Occurrences of a String in JavaScript Using RegEx? Click Here to Read!
Summary
In short, the regex stands for regular expression, a sequence of characters used to match substrings with strings to filter the result. You can use methods like test() to check the presence of a regex pattern in a string and if that’s a match it returns true or it returns false. Regex can be created using the various patterns we discussed above and you can also use flags to modify the filtration further.
That’s all for this article. If you like it, be sure to check out our main page, you will find a lot of interesting JS articles that make all the difficult concepts easy.
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_expressions