We would like to run codes & get the results swiftly but there should be some means to store the data such as input or deduced results right? Wonder where they go? Enter the Variables. These are destined to serve as a depository of the umpteen values that would be used in Python programming.
In this article, we shall deep dive to explore Python variables through each of the following sections.
- Variables definition
- Rules for Python variables
- Assigning values to variables
- Declaring variables
- Re-declaring variables
- Memory allocation
- Concatenating variables
- Local & Global variables
- Deleting variables
Variable Definition
An exclusive entity that reserves a memory location in order to store the values assigned throughout a set of codes to run a program is called a Variable. At times, it comes around with an alias – bucket; might be due to the practice of using it as a storage dump for values!
Therefore we can store the value, fetch the value and if necessary, we can also make changes to the value and store it again. By this, we can repeat to retrieve and maintain information that serves as input data to be processed by the Python programming.
Rules for Python Variables
However, there are a set of rules that ought to be followed when putting these variables into use such as those listed below.
- Variables cannot be a keyword.
- Variables can contain only upper & lower case letters, numbers, and underscore.
- Variables are case sensitive – Variable and variable is not the same when declared in Python programming.
Note:
We don’t need to initialize any variable manually in Python & can declare it at once.
Assigning Values to Variables
The equals sign “=” is an operator that is used to assign any value to the variables. This assignment works from right to left manner, meaning that those to the right of “=” are assigned to those which are to the left. Given below are some examples to understand how this operator works.
Example 1:
a = 5
b = 5
c = a + b
print(c)
Output:
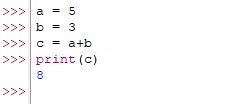
Example 2:
d = 3
e = 5
d += e
print(d)
Output:
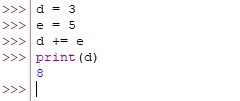
Declaring Variables
Python has no specific command to declare variables as shown in the below code where we assign some string to a variable and print it.
Example:
ip = "Samosa"
print(ip)
Output:
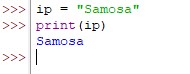
Re-declaring Variables
In Python, if you are using a variable to declare a specific value and then re-declare another value in the same variable which is used previously, it gets allotted automatically in a jiffy.
Example:
y = "Python is a good skill to learn"
print (y)
y = 20
print (y)
Output:
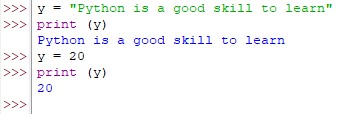
Memory Allocation in Variables
Though one can store umpteen values in the same variable, Python will finally store only the recent value in the memory allotted. It is then processed by the program to which it is assigned. There are two types of memory allocation in Python viz.
- Static memory allocation – The memory allocation that will happen during the time of compilation and is not a reusable memory.
- Dynamic memory allocation – The memory allocation that will happen during the runtime. This is a reusable memory.
Concatenating Variables
Combining some variables together by using “+” operator is known as concatenating. Let us have a look at how it works.
Example:
a = "Good "
b = "Morning"
c = a + b
print(c)
Output:
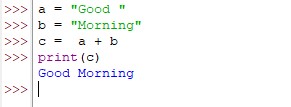
Local and Global Variables
Local variables are those which are defined within a function and can only be modified within that function. So, if one plans on doing any changes to a local variable, it shall reflect only within the function.
Example:
def func():
s = "Good Morning"
print(s)
func()
print(s)
Output:
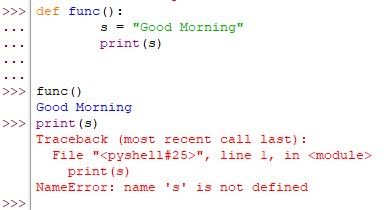
Global variables are those that are initialized outside a function. They are not bounded by a function & any changes done in a global variable will reflect everywhere in the program.
Example:
def func():
print(x, “Would you like some samosa?”)
x = "Good Morning"
func()
print(x)
Output:
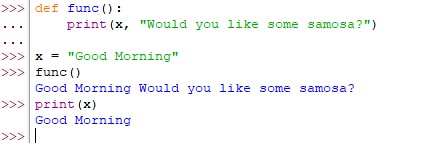
Deleting Variable
The easiest way to delete any variable is by using the keyword del. In the following example, we shall try deleting the first element from the list created.
Example:
a = ["Names", "Place", "Things"]
del a[0]
print(a)
Output:
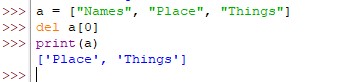
Conclusion
Now that we have reached the end of this article, hope it has elaborated on the variables used in Python. Here’s another article that can be our definitive guide to the if else statement in Python. There are numerous other enjoyable and equally informative articles in CodeforGeek that might be of great help for you to gain valuable insights. Until then, ciao!