Any mathematician or physicist or statistician would be well-versed with running iterations. It is with these iterations that the desired result is obtained. When it comes to coding there are a handful of techniques through which iterations can be done.
In this article, we shall explore the workings of the for loop in Python through each of the below sections:
- Introduction to Python for loop
- Syntax of for loop
- Examples of using for loops
Python For Loop
A control flow statement that enables the iteration over a sequence until all items in the sequence have been processed is called as for loop. This allows you to execute a block of code for each item in the sequence. The iteration ends based on the completion of iterating through all the items in the sequence. The capabilities of the for loop extends towards iterating a large number of entities such as string or lists or tuples.
Syntax of For Loop
The for loop can be coded using a couple of syntaxes, each given below.
Syntax 1:
for val1 in val2:
<statement block>
Syntax 2:
for val in range (<startvalue>, <endvalue>, <jumps>):
<statement block>
The latter syntax harnesses the potential of the range function which makes it easier to specify the boundaries within which the for loop ought to operate. It also provides the flexibility for specifying the increments at which the iterations are to be executed.
Syntax 3:
for val1 in val2:
for val3 in val4:
<statement block>
<statement block>
Some might have guessed it by now. The above is the representation of the nested for loop. These are iterations within iterations such that the result obtained by ‘x’ iterations of the innermost condition would then be fed as an input for the ‘y’ iterations of the outermost condition.
Examples of using For Loop
This section shall detail the different variations in which the standard for loop could be utilised in Python. Let us get started with how to use it with a list.
Example 1:
Given below is a list, each of whose elements is to be incremented by one using the for loop.
ar1 = [20, 57, -10, 89]
for i in ar1:
print(i+1)
Output:
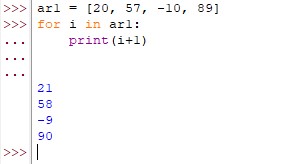
Example 2:
Not only numbers but also strings can be iterated through for loop. In the below code, let us have a look at how a list of string is iterated using a for loop.
snacks = ["Samosa", "Chips", "Murukku"]
for i in snacks:
print(i)
Output:
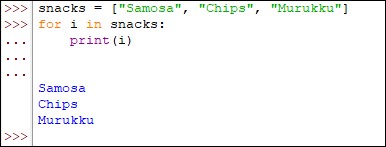
Example 3:
In both examples, we have mentioned ‘i’ after for, which represents each entity within the list that is to be put through the loop. Now let us have a look at how a for loop and the range function can be put to work together. The below code feeds the data within which the iteration is to be carried out using the range function. It states that the data shall start from ‘1’ and end at ‘90’ in increments of ‘10’.
for i in range(1,90,10):
print(i/2)
Output:
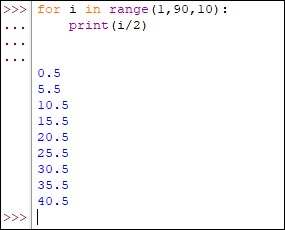
Example 4:
Finally, let us get on with the workings of a nested for loop through the below code. Here the numbers from the range specified in the first for loop will be printed the same number of counts they stand for (i.e.) the number ‘2’ gets printed 2 times & so on & so forth.
for i in range(2,9,2):
for j in range(i):
print(i, end = ' ')
print()
Output:
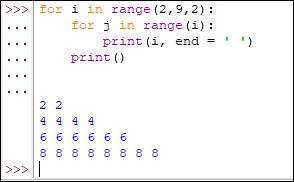
Conclusion
Now that we have reached the end of this article, hope it has elaborated on the different variations in which for loop can be put to use in Python. Here’s another article that can be our definitive guide to the variables in Python. There are numerous other enjoyable and equally informative articles in CodeforGeek that might be of great help for you to gain valuable insights. Until then, ciao!