Even a movie is not worthy of being watched when the logic is tossed off the roof. The same goes for the coding too. One of the important entities to be understood before one is ready to unleash the all-powerful Python engine is its logical operators. Yep! The same oldies that go synonymous with the name Boolean. These operators are as far as logic can get in programming.
Any decision-making can be carried out by coding a condition using any of the below listed logical operators:
- Logical AND
- Logical OR
- Logical NOT
What are Logical Operators?
The operators that aid the decision-making process by factoring in the results of a single or multiple conditions, only to return a result in True or False are called the logical operators. It might seem very simple, but these operators lay the very foundations for many decision matrices to operate upon. This very True or False will determine the difference between success and failure while developing machine learning models.
Logical AND Operator
This guy is pretty much a very strict chap. Mostly, like the faculty who threatens you during your viva voce examination. It returns a result as True only when both conditions are met. Else, it shall throw False in every other instance.
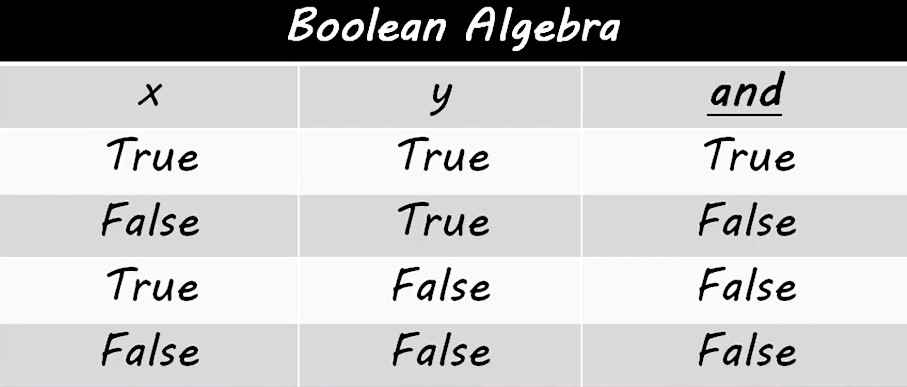
Example of Logical AND Operator
Have a look at the below example in which the logical AND is put to use.
ip = int(input("Enter your marks:"))
if ip>80 and ip<100:
print("Congratulations!")
Output:
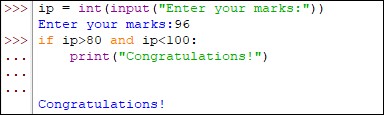
However, when one enters an input that defies the conditions included on either side of the logical AND operator, the result is simply empty. (That’s because there is nothing alternate that can be done in the given code).
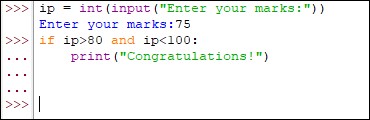
It is to be noted that the logical AND operator in Python works by verifying initially the condition to the left of it & only if it is met, it shall move on to verify the other condition specified to its right. Else, it shall return False right away.
Logical OR Operator
This chap is more lenient when compared to the previous. He is that faculty who lets you play games during the class hours. As stated in the tabulation below, the logical OR returns the result as True if any of the conditions given are met & yes it also returns True if both conditions are met, duh!
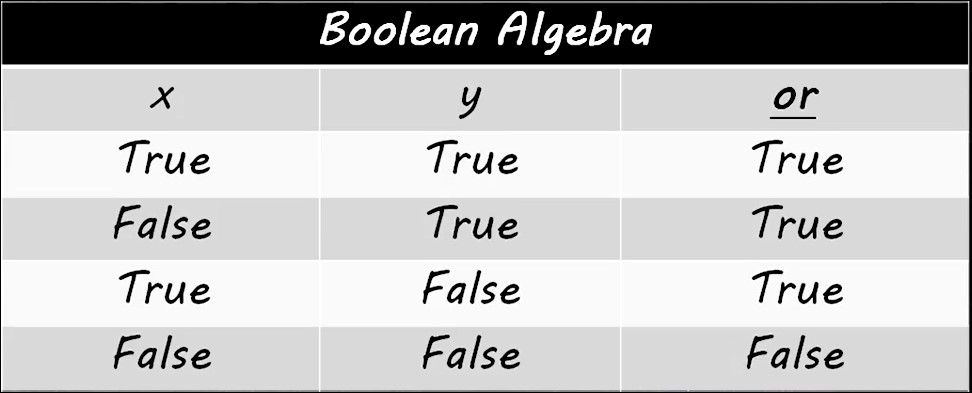
Example of Logical OR Operator
Given below is an example to understand Logical OR better.
ip = int(input("Enter your speed:"))
if ip>30 or ip<60:
print("Correct speed!")
Output:
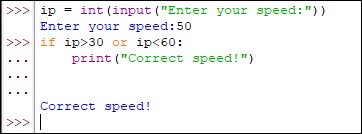
Logical NOT Operator
Unlike the above two logical operators, the logical NOT works only with one input. It is that friend of yours who does exactly the opposite of what you tell them to do. The logical NOT return the opposite of the result (i.e.) returns False when the result is True and vice versa. In a geeky context, the logical NOT negate the result deduced from a given condition.
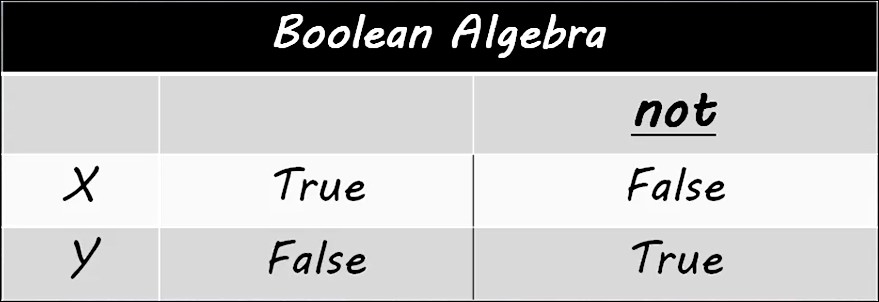
Example of Logical NOT Operator
In the following example, we can attempt to restrict user access based on gender using logical NOT.
ip = int(input("Enter '0' if male & '1' if female:"))
if not(ip==0):
print("Please ask a male to use this")
Output:
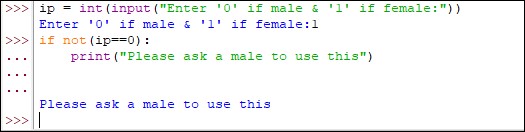
It can be observed in the above example that the code is using the logical NOT to print a message when the opposite of the condition given within the parenthesis occurs.
Conclusion
Now that we have reached the end of this article, hope it has elaborated on the different logical operators that are available in Python. Here’s another article that can be our definitive guide to the keywords in Python. There are numerous other enjoyable and equally informative articles in CodeforGeek that might be of great help for you to gain valuable insights. Until then, ciao!