Python provides many libraries that can perform various functions in different fields. One such famous library is NumPy. NumPy provides various mathematical functions that help us perform computations on arrays, scalars and matrices. In this article, we’ll explore the numpy.cumprod() function which helps compute cumulative product along with some examples.
Also Read: numpy.square() in Python
Introducing numpy.cumprod() Function
numpy.cumprod() is a function provided by NumPy that calculates the cumulative product of elements in an array.
The cumulative product of the ith element in the input array returns the product of all the elements until the ith element in the output array (including the ith element).
Syntax:
numpy.cumprod(arr, axis=None, dtype=None, out=None)
- arr: Input array whose cumulative product is to be calculated.
- axis: Specify the axis along which cumulative product should be computed. It is mainly used for computations involving 2D arrays (matrices), where it takes a value of 0 indicating column-wise product is returned and 1 indicating row-wise product is returned.
- out: Name of the output array. If set to None or not given a value, a new array will be created to store the resultant array containing cumulative products.
- dtype: Used to specify the data type of the output array. Here, None indicates that the data type of the output array must be inferred according to that of the input array.
Let us now understand how we can use numpy.cumprod() with some examples.
Calculating Cumulative Product Using numpy.cumprod() Function
In this section, we’ll understand how to use numpy.cumprod() to find the cumulative product of elements in an array. We’ll also look at some examples with matrices and other examples where we change the various parameters (like axis) to make changes in the output array.
Finding Cumulative Product in an Array
The most basic example is using numpy.cumprod() to find the cumulative product of elements in an array. Here, we’ll take a NumPy array of numbers and find its cumulative product.
import numpy as np
array = [1, -2, 3, -4]
cumulative_product = np.cumprod(array)
print(cumulative_product)
In order to use the cumprod() function we have to first import the NumPy library as np. The output array containing cumulative products is stored in cumulative_product.
Output:
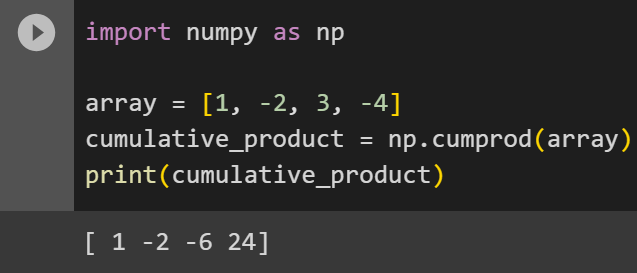
- Here, the 0th index in the output array contains 1, the first element of the input array.
- The 1st index of the output array contains the -2, which is the product of the first two elements in the input array (1 x -2 = -2).
- The 2nd index of the output array contains -6, which is the product of the first 3 elements in the input array (1 x -2 x 3 = -6) and so on for the rest of the elements in the output array.
Calculating Cumulative Product in Matrices Using the Axis Parameter
Similar to how we use numpy.cumprod() for the cumulative product in an array, we can use it to find the cumulative product of elements in a matrix as well. Here, since we’re using a matrix, we’ll also use the axis parameter to indicate whether we want to perform row-wise or column-wise multiplication.
Setting axis = 0 for Column-Wise Computation of Cumulative Product
import numpy as np
matrix = np.array([[1, -2, 3],
[-4, 5, -6]])
cumulative_product = np.cumprod(matrix,axis=0)
print(cumulative_product)
The product will be calculated column-wise.
Output:
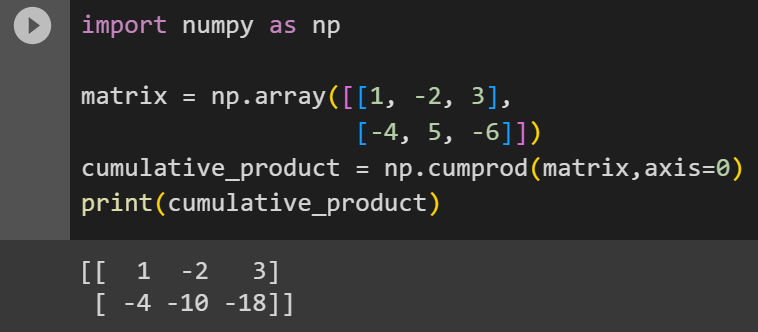
- Here, the cumulative product of the first three elements in the output array are the same as the first three elements of the input array.
- The 4th element of the output array is -4, which is the product of the elements at i[0][0] and i[1][0] (1 x -4 = -4). This process is repeated for the rest of the elements in the output array as well.
Setting axis = 1 for Row-Wise Computation of Cumulative Product
import numpy as np
matrix = np.array([[1, -2, 3],
[-4, 5, -6]])
cumulative_product = np.cumprod(matrix,axis=1)
print(cumulative_product)
The product will be calculated row-wise.
Output:
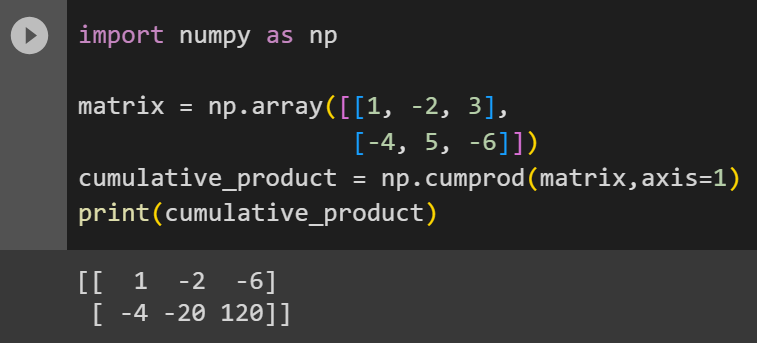
- Here, the first element of every row in the output and input array are the same.
- The element at i[0][1] is -2, which is the product of the first two elements of the first row in the input matrix (1 x -2 = -2). The same process is repeated for elements of that row.
- Again, the element at i[1][0] is the -4, which is the same as the input array.
- The same process that was performed in the first row is then repeated for all other rows.
Conclusion
Calculating the cumulative product of an array may come in handy in many situations. In this article, we’ve explored the numpy.cumprod() function provided by Python’s NumPy library which does this task for us in just a single line of code. We have seen with various examples, how we can efficiently use this function on arrays and matrices while exploring parameters like axis, which lets you customize the output according to your requirements.