Sorting Pandas DataFrame involves arranging its rows based on one or more column values. Sorting can be done when we need to identify the top or the bottom values which can be helpful in selecting the most deserving data points. Sorting can also be proved useful in the time-based data where we can sort timestamps which gives us the analysis for changes over time. Also, we can obtain more efficient and accurate operations when we sort data before merging DataFrame. Basically, Soring has lots of use cases.
In this article, we’ll look at 6 ways to Sort Pandas DataFrame in Python.
Also Read: How to Drop One or Multiple Columns in Pandas DataFrame (5 Ways)
Methods for Sorting Pandas DataFrame
For Sorting Pandas DataFrame let’s first create a DataFrame using a CSV file.
Example:
import pandas as pd
df = pd.read_csv('nba_player_stats.csv')
df.head()
Here we first imported pandas as pd, then created the DataFrame using a CSV file, the name of the CSV file is nba_player_stats.csv and after that, we printed the head of the DataFrame df.
Output:
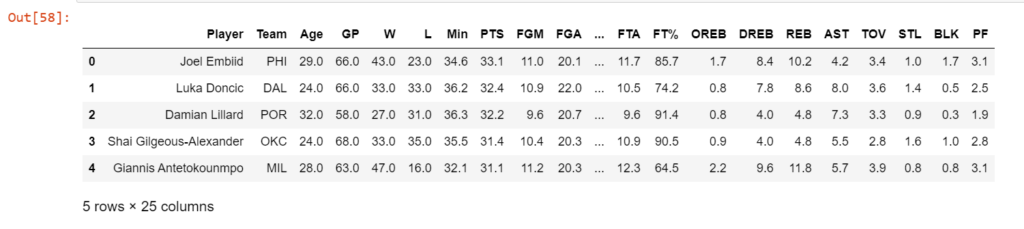
We will use the sort_values() method for sorting DataFrame and there are six ways through which we can sort the above Pandas DataFrame which are as follows:
- Sorting DataFrame on a Single Column
- Permanently Sorting a DataFrame on a Single Column
- Sorting DataFrame on Multiple Columns
- Sorting DataFrame in Descending Order
- Sorting DataFrame by Positioning Null Values
- Sorting DataFrame on Key
1. Sorting DataFrame on a Single Column
We can sort the DataFrame on a Single Column by using the sort_values() method.
Example:
df.sort_values(by='Player').head()
Here we have written df.sort_values(by=’Player’).head() means we have specified the Player column and sorted the DataFrame df by the Player column. After running we have seen that we have alphabetically sorted DataFrame df on the Player column.
Output:
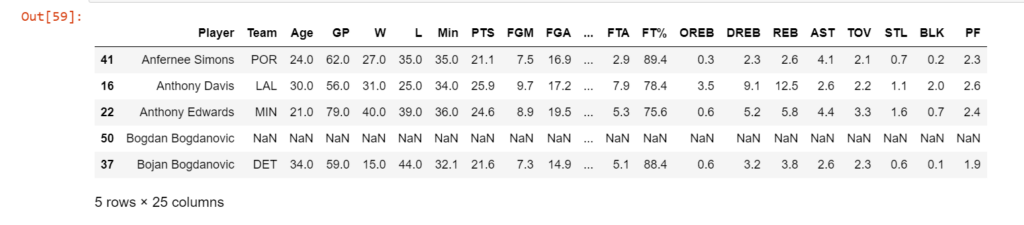
2. Permanently Sorting a DataFrame on a Single Column
We can sort the DataFrame on a Single Column Permanently by using the same sort_values() method by passing inplace=True as an argument.
Example:
df.sort_values(by='Player', inplace = True, ignore_index=True)
df.head()
Here we have written df.sort_values(by=’Player’, inplace = True, ignore_index=True) means we have called the sort_values method in which we specified Player Column means we have sorted the DataFrame based on Player column and then we have mentioned inplace = True which means we sorted the DataFrame df permanently and we have also mentioned ignore_index=True for re-indexing as it was out of order.
After running we have seen that the layout got permanently changed and DataFrame got sorted based on the players going alphabetically from A to Z and we have also seen that we were able to re-index.
Output:
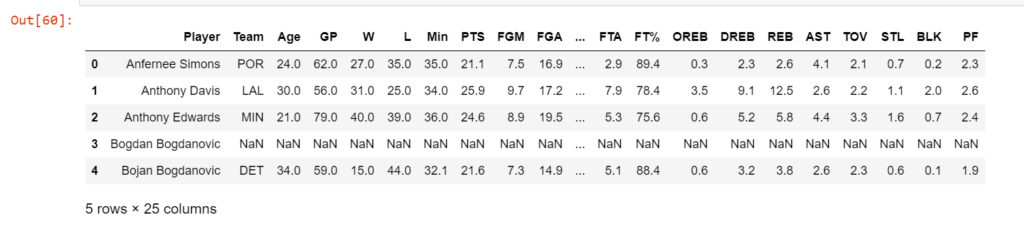
3. Sorting DataFrame on Multiple Columns
We can also sort the DataFrame on Multiple Columns by using the sort_values() method.
Example:
df.sort_values(by=['Team','Player']).head()
Here we have written df.sort_values(by=[‘Team’, ‘Player’]).head() means we have called the sort_values method in which we have passed a list of Team and Player columns which gives us the result such that our DataFrame got sorted First by Team column then by Player column.
Output:
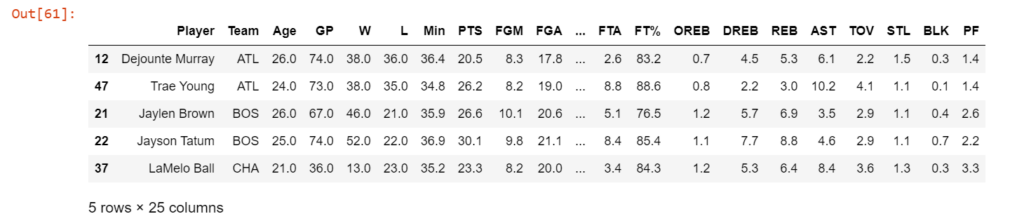
4. Sorting DataFrame in Descending Order
We can sort DataFrame in Descending Order by specifying ascending=False in the sort_values() method.
Example:
df.sort_values(by='Player', ascending=False).head()
Here we have written df.sort_values(by=’Player’, ascending=False).head() means we have called the sort_values method in which we specified to sort the DataFrame by Player and we have also specified ascending=False which sorted our DataFrame in Descending order.
Output:
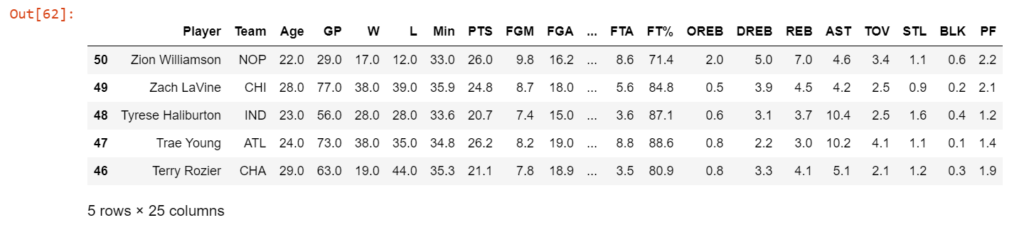
5. Sorting DataFrame by Positioning Null Values
We can also sort DataFrame on the basis of positioning the null values by specifying na_position in the sort_values method.
Example:
df.sort_values(by='Team', na_position='first').head()
Here we have written df.sort_values(by=’Team’, na_position=’first’).head() which sorted the DataFrame df on the basis of the Team column and we have also specified na_position=’first’ which listed the rows with null values first.
Output:
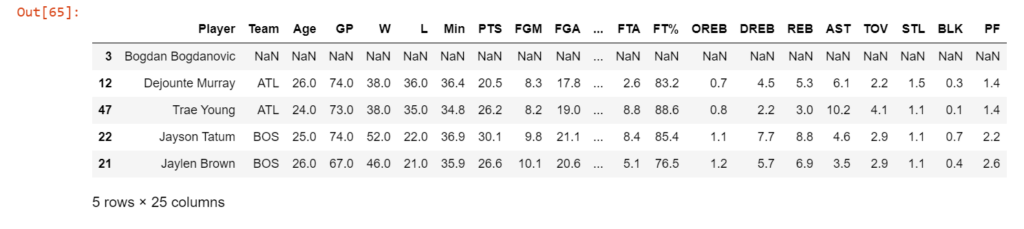
6. Sorting DataFrame on Key
We can sort the DataFrame on a specified key.
Example:
df.sort_values(by='Player',key=lambda name: name.str.len()).head()
Here we have written df.sort_values(by=’Player’, key=lambda name: name.str.len()).head() means we have taken the Player column for sorting and then sorted them based on the length of their characters.
Output:
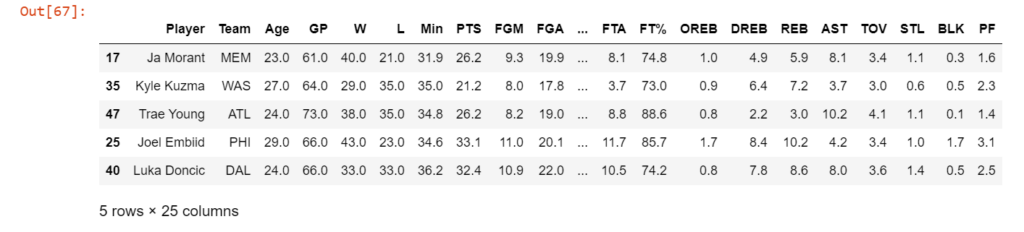
Summary
Sorting is crucial for organizing data, identifying patterns, and preparing data for analysis or visualization. Whenever we plot data in ascending or descending order, sorting a DataFrame can enable a clearer visualization. In this article, we have discussed six ways of sorting the Pandas DataFrame with examples. After reading this tutorial, we hope you can easily Sort Pandas DataFrame in Python.
Reference
https://stackoverflow.com/questions/37787698/how-to-sort-pandas-dataframe-from-one-column