NodeJS StringDecoder is used to decode the buffer data into a string. Buffer is used to storing the streams of binary data, since this binary data can only understandable by a computer, it is required to convert it into a string so that humans can read it.
There is a method buffer.toString() provided by the buffer class which is also used to convert the buffer data into a string, the difference is that StringDecoder provides extra support for UTF encoding. We have a separate article on Node.js Buffers, consider it if you want to read more about the buffer and its method including the buffer.toString() method.
Node.JS StringDecoder Module
StringDecoder is a built-in Node.js module, so it is not required to install it, we just have to import it using the below statement.
Syntax:
const { StringDecoder } = require("string_decoder");
Methods of Node.JS StringDecoder
There are two methods, the write() method and the end() method provided by StringDecoder to decode a buffer into a string having a slite different functionality.
1. write()
This method decodes the data of the buffer passed as an argument and returns the decoded string. This method omits the incompleted byte characters at the end of the buffer and then stored them in the internal buffer for the next call to the StringDecoder methods.
Syntax:
write(buffer)
Where the buffer can be a standard JavaScript buffer, TypedArray or Data.
Example:
Let’s start with importing the StringDecoder, then create an object passing the ‘utf-8’ encoding to the argument for StringDecoder class. This object is used to call the write() method.
const { StringDecoder } = require("string_decoder");
const decoder = new StringDecoder("utf-8");
Create a new buffer using the Buffer.from method. We have covered this method in another article Node.js Buffer.
const buffer = Buffer.from("This is a buffer");
Here we pass a string as an argument to this method that initialized the new buffer with that string.
Then use the write() method passing the buffer as an argument and setting it to a variable.
const buffer_text = decoder.write(buffer);
Finally, print the result.
console.log(buffer_text);
Complete Code:
const { StringDecoder } = require("string_decoder");
const decoder = new StringDecoder("utf-8");
const buffer = Buffer.from("This is a buffer");
const buffer_text = decoder.write(buffer);
console.log(buffer_text);
Output:
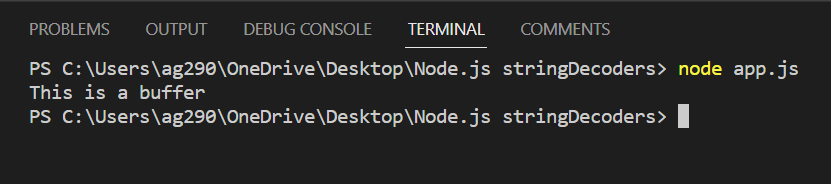
2. end()
This method decodes all the remaining input stored in the internal buffer to a string and returns it. It also uses some alternative characters to replace the incomplete UTF-8 and UTF-16 characters to make them suitable for character encoding.
Syntax:
end(buffer)
Where the buffer can be a standard JavaScript buffer, TypedArray or Data.
Example:
The importing statement is the same as in the previous example. We first import the StringDecode class and then create an instance of that class used to call its methods.
const { StringDecoder } = require("string_decoder");
const decoder = new StringDecoder("utf-8");
Then use the write method passing a buffer that uses the [0xE2, 0x82, 0xAC] as an argument that represents the euro symbol.
const buffer = decoder.write(Buffer.from( [0xE2, 0x82, 0xAC]));
Then use the end() method, which returns the data stored in the internal buffer by decoding it into a string, which is the euro symbol.
console.log(decoder.end(buffer));
Complete Code:
const { StringDecoder } = require("string_decoder");
const decoder = new StringDecoder("utf-8");
const buffer = decoder.write(Buffer.from( [0xE2, 0x82, 0xAC]));
console.log(decoder.end(buffer));
Output:

Summary
Node.js uses buffers to store the streams of binary data, since the binary data is only understandable by computers we have to decode it into a string in order to make it readable by humans. Hopefully, we can decode buffer data into the string using a Node.js build-in module called Node.js StringDecoder which provides two methods write() and end() which return decoded string of the buffer pass as an argument to these methods. Hope this article helps you to understand the use of Node.js StringDecoder.
Reference
https://nodejs.org/api/string_decoder.html