A collection with respect to MongoDB is used to store documents. It is similar to a table in a relational database.
A relational database such as MySQL database holds multiple tables to store different records similarly a MongoDB database holds multiple collections to group different documents together but unlike the relational database, it doesn’t store data in rows and columns, it uses a key-value like structure for storing data inside documents where the key acts like a column header with the value init.
If these details excite you, let’s see how we can create a new collection in MongoDB using Node.js. Don’t worry if you are new to Node.js, I will bring some very basic code for this.
Prerequisites – Node.js MongoDB Create Database
Creating Collection in MongoDB with Node.js
MongoClient has a method connect() which takes a URI and a callback as an argument. URI is a MongoDB connection string used to connect to the MongoDB database and a callback is a function that returns the error or the client object.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
// callback body
});
If you find the above code confusing, we recommend reading our previous tutorial where we have covered the entire process of creating a database.
This client object has a method db() that takes the name of a database as an argument to create a database object.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db(databaseName);
});
This database object can use the method createCollection() to create a new collection.
database.createCollection(collectionName, function (err, client) {
// callback body
});
The complete code snippet for creating a collection:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db(databaseName);
database.createCollection(collectionName, function (err, client) {
// callback body
});
});
where,
- databaseName is the name of a database in which you want to create a collection,
- database is a database object,
- collectionName is the name of the collection you want to create
Alternate Method for Creating a MongoDB Collection
The above way might be complex for a new developer so here is an easy way of creating a collection.
You can pass the database name in which you want to create a collection in the connection string(URI) at the end after a slash(“/”). Then inside the callback, you can directly call the createCollection() method using the database object.
An easy code snippet for creating a collection:
var MongoClient = require('mongodb').MongoClient;
MongoClient.connect("mongodb://localhost:27017/databaseName", function(err, db) {
if (err) throw err;
db.createCollection(collectionName, function(err, res) {
// callback body
});
});
where,
- databaseName is the name of a database in which you want to create a collection,
- db is a database object,
- collectionName is the name of the collection you want to create
Example of Creating a MongoDB Collection
Let’s now move to the practical demonstration and since we are going to create the collection using Node, make sure that the Node.js driver exists in your system.
Step 1: We need to create a folder containing a JavaScript file “app.js” where we will write our code.
Step 2: Locate the project folder in the terminal and execute the below command to initiate a Node.js project.
npm init -y
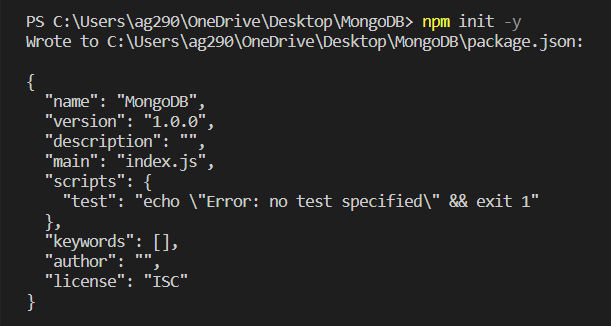
Here we have used NPM (Node Package Manager) which allows us to install any Node.js package from the online NPM repository. I have a dedicated NPM guide if you want to read it: A Beginner’s Guide to Node Package Manager
Step 3: Now let’s use NPM to install the MongoDB module.
npm i mongodb
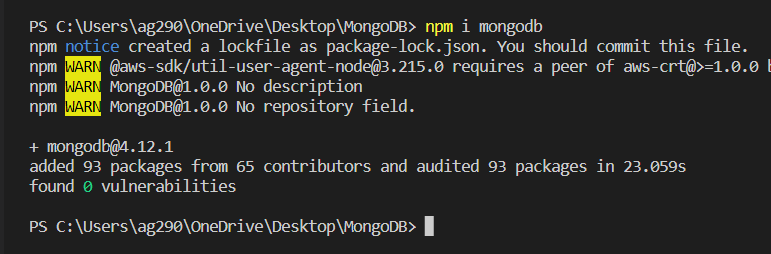
Step 4: Open the “app.js” file and import the MongoClient from the MongoDB module.
const { MongoClient } = require('mongodb');
Step 5: Use the connect() method to pass a URI and then inside the callback use the client object to use the db() method and pass “newdatabase” as a database name which we have created in our previous tutorial.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
});
Step 6: Finally, use the createCollection() method to pass a collection name to create it, and inside the callback check for the error, throw the error if occurs, then log the successful message in the console and close the client.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.createCollection("newcollection", function (err, res) {
if (err) throw err;
console.log("Collection created!");
db.close();
});
});
Running Node.js Script
The application code is written in a single file “app.js” that does the operation on executing the below command in the terminal.
You can now execute the query to insert a document or multiple documents into your collection, I have a detailed guide on that, click here to read it.
Using MongoDB Compass to Verify Collection Creation
After inserting the data, you can verify that the specified collection is successfully created by using MongoDB Compass which provides a graphical way to interact with the MongoDB database. All you need is to open the MongoDB compass, enter the MongoDB URL and press enter.
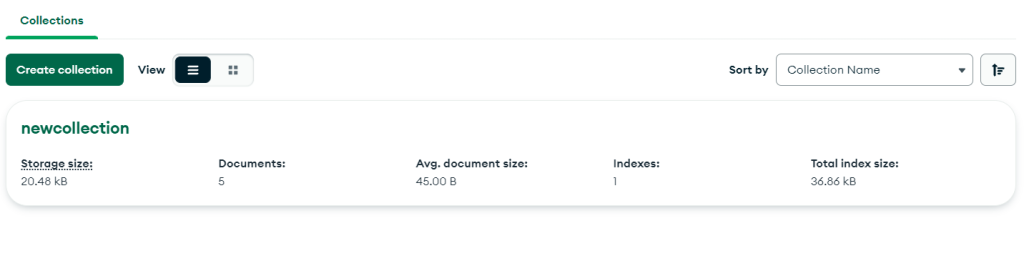
Here you can see that the collection name “newcollection ” has been created inside the database name “newdatabase”.
If you find that the collection does not exist, try looking at the MongoDB connection URL and make sure you entered the correct URL.
Summary
A relational database like MySQL uses tables to store data in the form of rows and columns whereas a MongoDB database uses collection to store documents in the form of key-value pairs where keys act as fields. We can create a collection inside a database using the createCollection() method in two ways, either by using Mongo Shell or by using the killer combination Mongo + Node.js that we have seen in this tutorial.
If you are new to MongoDB & Node.js:
- What is Node.js?
- Asynchronous Programming in Node.js – Callback, Promises & Async Await
- $ (update) Operator in MongoDB
Reference
https://www.mongodb.com/docs/manual/reference/method/db.createCollection/