Node.js is a JavaScript runtime that allows you to write JavaScript for creating back-end services. We can create services like APIs, Web App even Mobile App using it. Many big companies use Node.js to build their application such as Netflix, PayPal, Uber, etc.
Also read: NodeJS: Installation, Setup, and Creating a Hello World Application in NodeJS
What are loops in NodeJS?
Loops in Node.js are used to iterate through an expression for a particular condition. It makes it easy to write code without duplicating the expressions and provides a way to easily navigate through the element of the array, or properties of an object by using a few lines of code.
While writing Node.js code, it is recommended to not replicate the expressions
console.log(“Hello World!”);
console.log(“Hello World!”);
console.log(“Hello World!”);
console.log(“Hello World!”);
console.log(“Hello World!”);
Instead of writing the code again and again you can use loops to make your code non-replicate.
The above code can be written as
for(i=1, i<=5, i++){
console.log(“Hello World!”);
}
There are many loops that you can use in Node.js, let’s see them one by one.
Types of Loops in Node.js
- for
- while
- do/while
- for/in
- for/of
1. For Loop
For loop is used when there is a necessity to loop through a certain element on the basis of a condition.
Syntax
for (initialization; condition; evaluation ){
// expression
}
Example
Print the numbers from 1 to 10 using for loop.
We have a condition that we have to loop the expression until the value of i becomes 10, We have to initialize the i as 1 so that our loop starts from 1 and goes to 10, and at last, we want to increase the value of i each time the loop runs so in evaluation we will type i++.
for (var i=1; i<=10; i++){
console.log(i);
}
Output
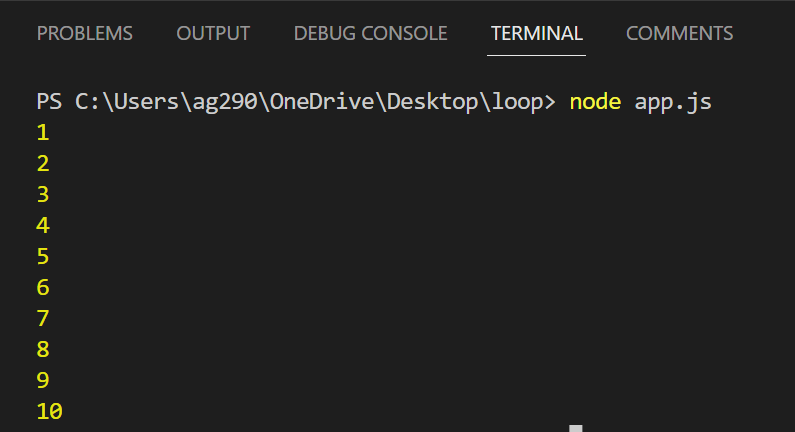
2. While Loop
While Loop is used to loop through an express till a particular condition is true.
Syntax
while ( condition ){
// expression
}
Example
Print the numbers from 1 to 10 using a while loop.
We have a condition that we have to loop the expression until the value of i is less than or equal to i.
i = 1;
while (i<=10){
console.log(i);
i = i + 1;
}
Output
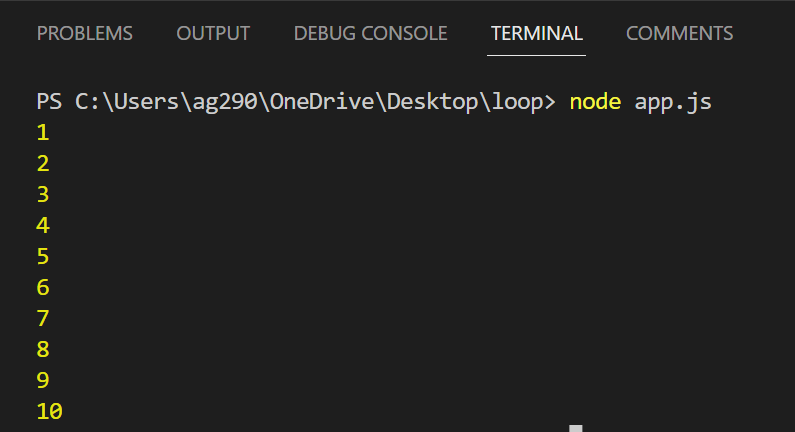
3. Do While Loop
Do while loop is different from for loop and a while loop, it first executes the expression then check for the condition and decides whether to execute the expression again or not.
Syntax
do {
// expression
}
while ( condition )
Example
Print the numbers from 1 to 10 using a do/while loop.
We have a condition that we have to loop the expression until the value of i is less than or equal to i.
i = 1;
do{
console.log(i);
i = i + 1;
}
while(i<=10)
Output
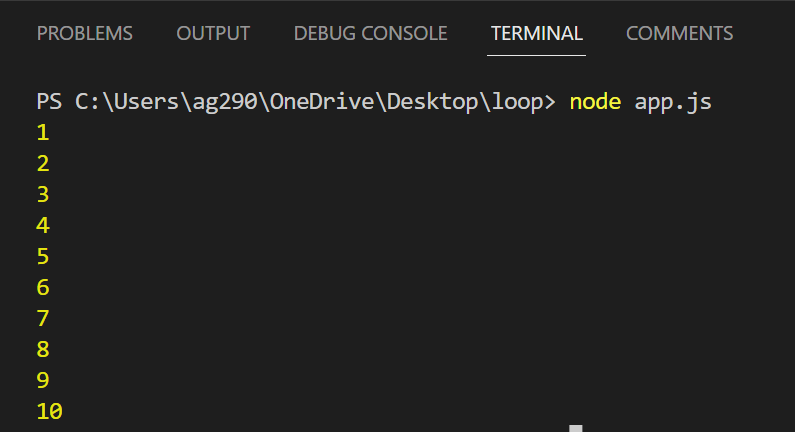
4. For In Loop
For In is the most commonly used loop in Node.js, it is used to loop through the key properties of objects.
Syntax
for ( key in object/array ) {
// expression
}
Example
var languages = { Good: 'JavaScript', Better: 'PHP', Best: 'Node.js' };
for ( languageKey in languages ) {
console.log(languageKey)
console.log(languages[languageKey])
}
Output
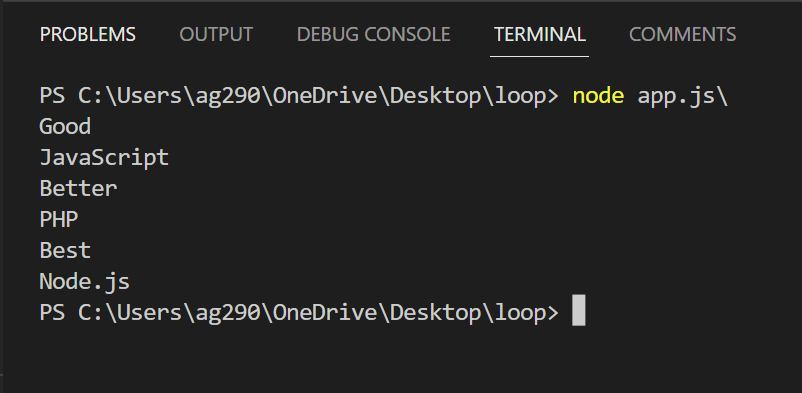
Example 2
Suppose we want to loop through an array without any key, so by default, the key is the position of an element inside the array like 0,1,2, etc.
var languages = ['JavaScript', 'PHP', 'Node.js' ];
for ( languageKey in languages ){
console.log(languageKey)
console.log(languages[languageKey])
}
Output
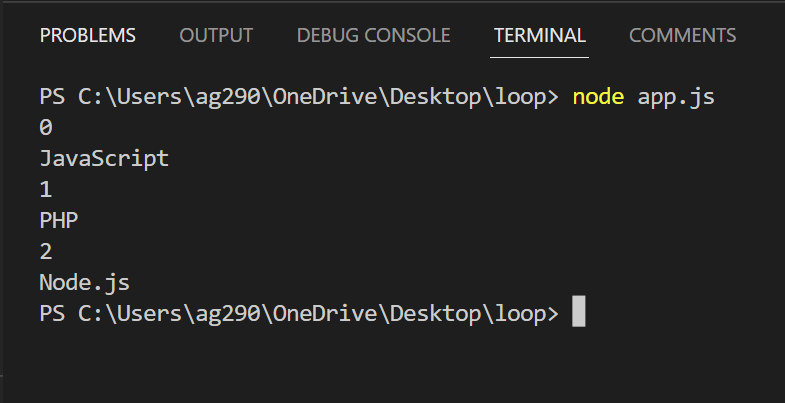
For Of Loop
This loop is used to loop through the actual element unlike for in loop which loops through the key property of elements.
Syntax
for ( element of object/array ) {
// expression
}
Example
var languages = ['JavaScript', 'PHP', 'Node.js' ];
for ( language of languages ) {
console.log(language)
}
We don’t have to manually grab the element using the key property.
Output
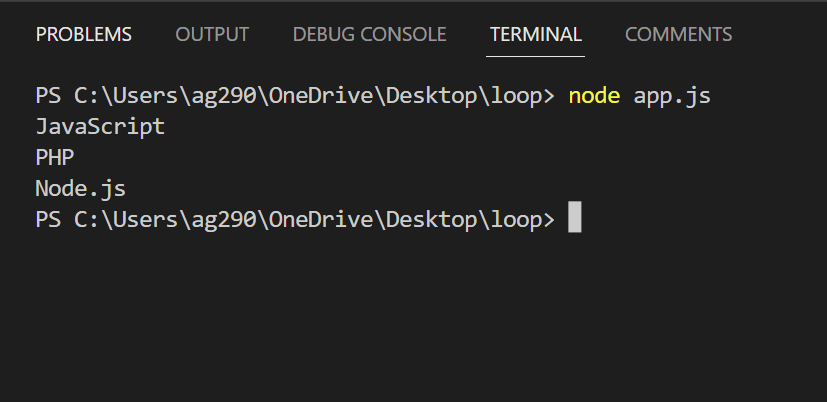
Summary
The loop makes it easy to write without duplicating the code. It can be used to literate an expression until a condition is true. It can also be used to navigate through the elements of an array/object. Using loops instead of replicating expressions will make your code cleaner and easy to debug. We hope this tutorial helped you understand the loop better.