In our previous tutorial, we learned how to create different routes using App Router. But when it comes to dynamic routing, the question is how to implement it in file-based routing where file names are used to create routes and since it is fixed, how can we create dynamic routes? Well, don’t worry the solution is simple: dynamic segments, let’s see it in this tutorial.
Dynamic Segment
To create a dynamic segment in Next.js we just need to wrap the folder name in square brackets:
[folderName]
Dynamic segments are passed as a prop to the layout, page, route, and generateMetadata functions so we can easily access it anywhere we want.
So if you create a route app/users/[user], you can access the user parameter easily in app/[user]/page.tsx to show dynamic content based on that and also handle errors if the request data is invalid.
For example, if a user makes a request to the URL app/users/aditya you can access “aditya” and send relevant data based on that or an error message if the user data does not exist.
Creating Dynamic Routes in Next.js
No more theories, let’s jump into the coding part to implement it in an actually Next.js application.
Setup:
Run the below command in the terminal to create a new Next.js application:
npx create-next-app@latest
Check Yes for both TypeScript and App Router to follow along with us:
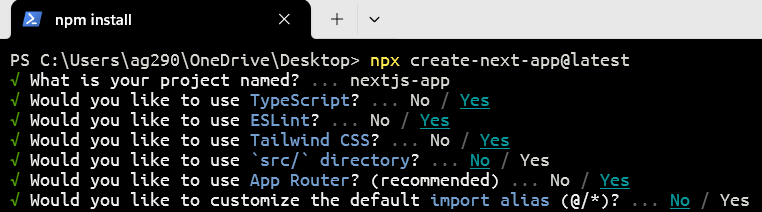
Now open the project inside a code editor and remove all the files inside the app directory.
Then only create a file page.tsx for our home page having the following code:
const HomePage = () => {
return <h1>Home Page</h1>;
};
export default HomePage;
To run the project, move on to the newly created project directory and execute the below command:
npm run dev
Now open the browser and paste http://localhost:3000 to see our home page:
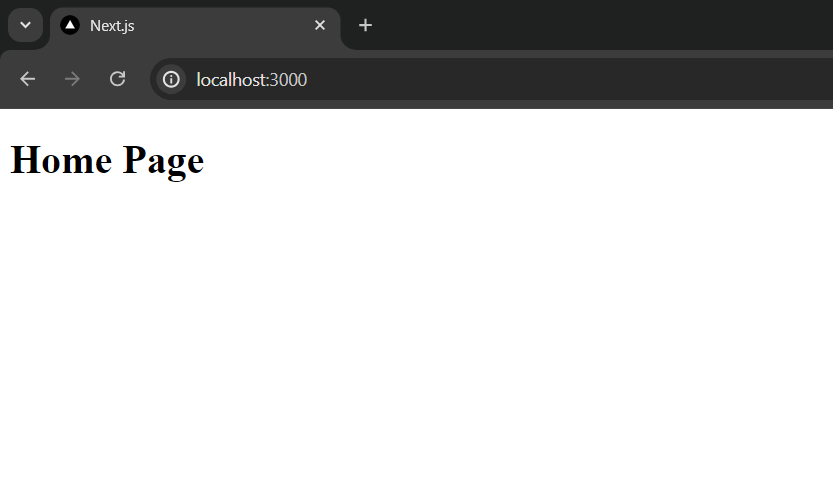
Everything works perfectly now!
Implementing Dynamic Route:
Now, let’s create a new folder inside the app directory named “user” with a file page.tsx and write the following code:
import React from 'react';
import { users } from '../../lib/userArray';
const UsersPage = () => {
return (
<div>
<h1>List of Users</h1>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
};
export default UsersPage;
This will show the list of users when a client tries to access the “/users” routes:
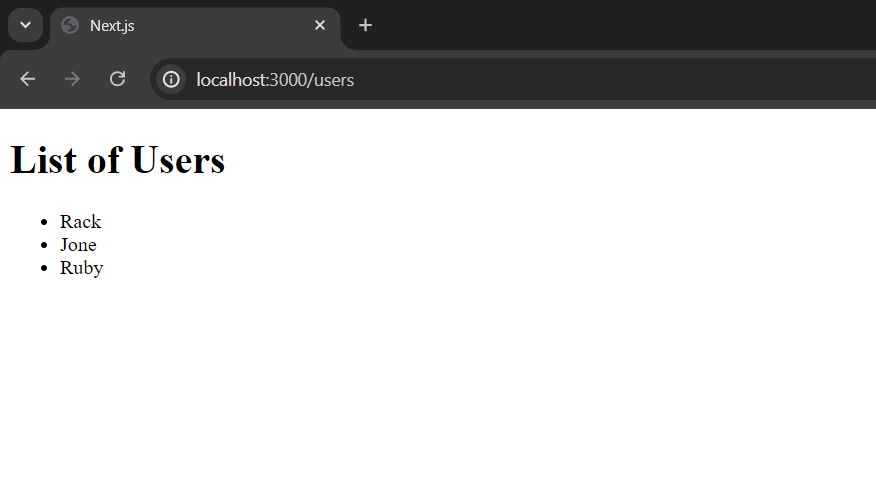
Now to create a dynamic route, create another folder named [user] with a file page.tsx having the following code:
import { users } from '../../../lib/userArray';
const User = ({ params }: { params: { user: string } }) => {
const userId = parseInt(params.user);
const user = users.find(user => user.id === userId);
return (
<div>
<h1>User Detail</h1>
<div>
<p>ID: {user?.id}</p>
<p>Name: {user?.name}</p>
<p>Age: {user?.age}</p>
<p>Email: {user?.email}</p>
</div>
</div>
);
};
export default User;
Now if a user makes a get request to the “/users/userId”, this userId will be captured inside the app/users/[user]/page.tsx and we can use it to find the relevant user information and send it back to the client.
Output:
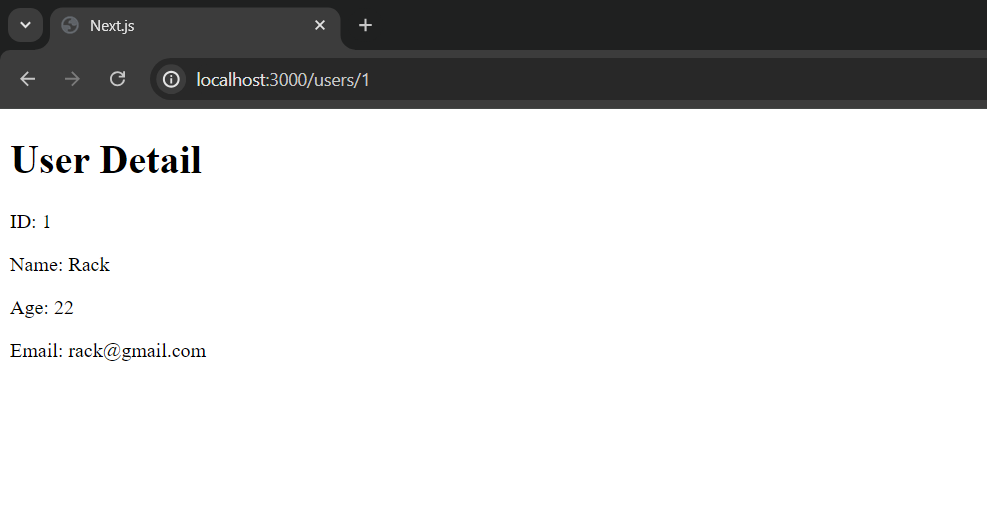
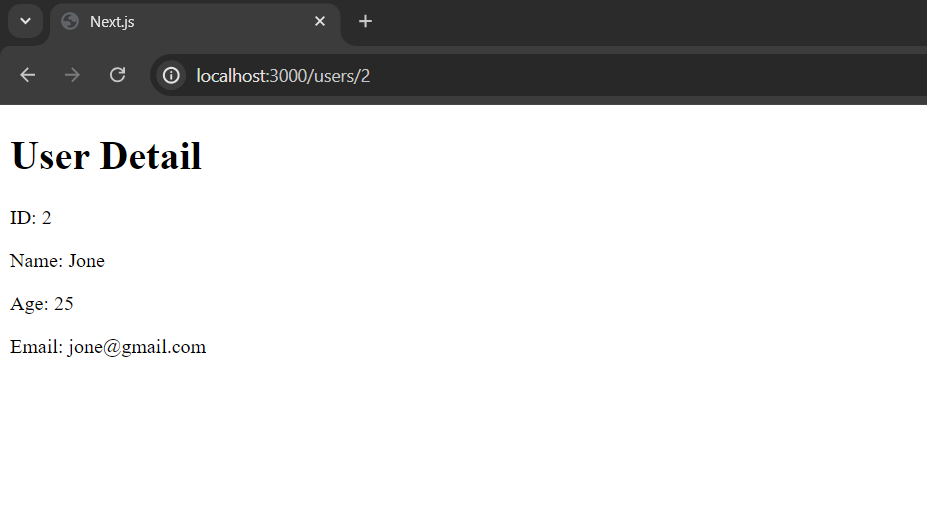
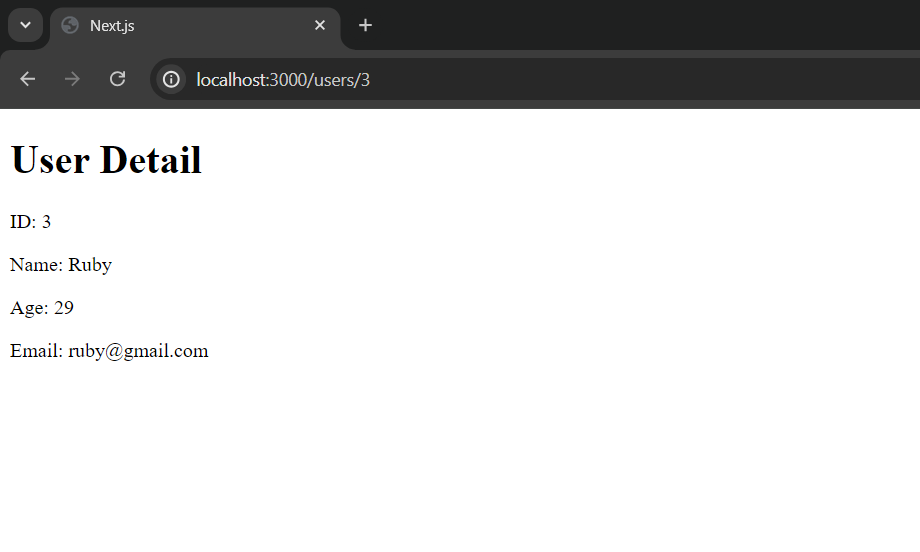
And, that’s it, our dynamic route is created!
Catch Nested Segments in Next.js
What if a user types a URL that has multiple segments like: /users/1/age, or even more nested, how can we get all those params?
Well, the answer is simple, all we have to do is put an ellipsis inside the square brackets that wrap a folder name:
[...folderName]
Example:
So now if we change our project directory from app/users/[user] to app/users/[…user], let’s see if we we able to catch all segments or not.
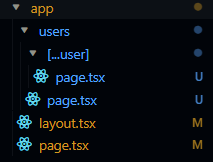
Let’s make app/users/[…user]/page.tsx a bit simple:
const User = ({ params }: { params: { user: string } }) => {
const user = params.user;
console.log(user);
};
export default User;
Open the browser and type multiple segments, like http://localhost:3000/users/1/age/params1/params2/etc:

Output:
Now, open the console and you will see that all segments got caught by our program:

That’s it, that’s how we catch all the segments in dynamic routes.
*What Next?
Summary
In Next.js, when we don’t know the exact segment name a user might request, we can create dynamic routes using dynamic segments.
To create a dynamic segment we just need to wrap the folder name in square brackets: [foldername]. Now we can access the segments inside /[foldername].page.tsx. Additionally, if we want to grab multiple segments, just put an ellipsis inside the brackets: […foldername]. That’s all about dynamic routes in Next.js.
Reference
https://nextjs.org/docs/app/building-your-application/routing/dynamic-routes