NPM (Node Package Manager) is the default package manager for installing and managing packages in Node.js. While installing a package using npm, we may require a certain version of that package to suit its compatibility with the remaining packages of our program. Let’s see two easy methods to do so.
How Do NPM Package Versions Work?
NPM packages have a specific standard for versioning packages called Semantic Versioning, or SemVer for short. When we install a package without specifying a version, NPM installs the latest version available in the registry, by default.
Version numbers are typically in the form Major.Minor.Patch:
- Major represents significant updates in the package, like changes in core functionality.
- Minor represent minor changes without change in functionality, like the addition of new features.
- Patch represents changes like bug fixes with no addition of new features or changes in functionality.
Keeping the Semantic Versioning system in mind we can better install the most apt versions of a package for our projects.
Why Install a Specific Version?
- To Avoid Compatibility Issues: A new version may not work with other previously installed dependencies, a specific compatible version solves this issue.
- To Maintain Project Stability: Specific versions prevent unexpected changes or bugs from new updates, making our project stable.
- For Fixing Bugs Easily: A particular versions make it easier to fix bugs, also if it is not so new, we can get help from the online docs for many errors.
- To Control Features: Fixed versions let us control the features and performance, avoiding unwanted changes.
- To Know How Code Works: Exact versions make our code behave as expected as we are already familiar with that version syntax.
Installing a Previous Exact Version of an NPM Package
Now that we know how versioning works in NPM, we can identify what version of a package we would like to install by going through the official documentation of that package and looking at the released updates. After we identified the package version it’s time to install it into our project.
We have two ways to install the exact version we want:
- Using the @ symbol in the npm install command
- Modifying the version in the package.json file
Let’s look at both of these methods with examples.
Using the @ symbol in the npm install command
The most common method to install a package is to use the npm install command. This command should be used after you have navigated to the root directory of your project.
The below command will install an exact version of a package:
npm install <package-name>@<version>
Example of npm install specific version:
Here, we will install an exact previous version of react (17.0.1):
npm install [email protected]
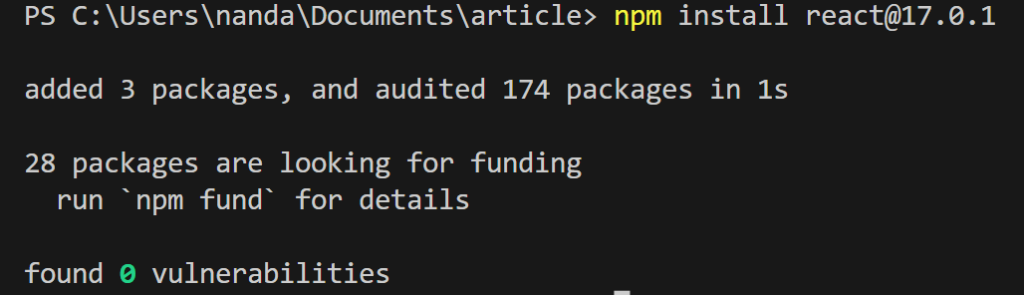
Also Read: What is the –save option for npm install?
Modifying the version in the package.json file
Another not so straightforward, yet simple method of getting an exact version of a package is to manually modify it to the version you want in the package.json project dependencies section. Let us see with an example how this can be done.
Example:
We have React installed with its latest stable version (18.2.0):
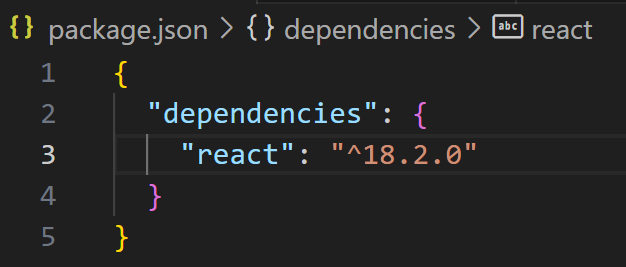
We will now manually modify this version to 17.0.1 in the package.json file:
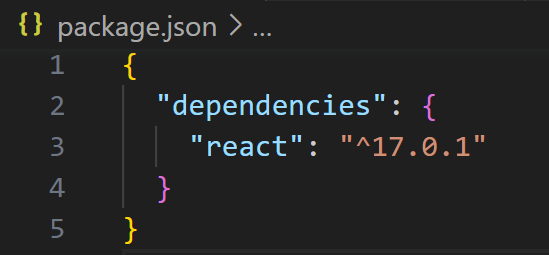
After updating the version in package.json we have to run the below command to make changes:
npm install
The version can be checked using the method given below.
Checking the Version of an Installed NPM Package
To verify whether the change in version has been reflected, we can check all the packages installed in our package.json file using the below command:
npm list
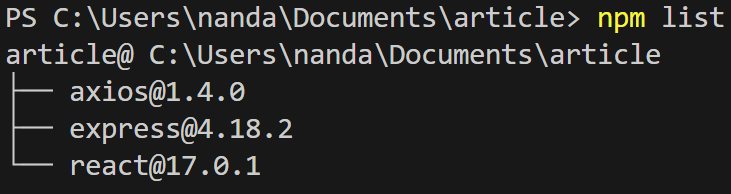
If we only want to check the version installed of a specific package, we use the command:
npm list <package-name>

Conclusion
Sometimes it is important to install a specific version of Node package to ensure compatibility with all other packages in a project. To get a compatible version for your needs, it’s crucial to understand how Semantic Versioning works in npm.
Once you decide, you can use the @ symbol in the npm install command to get that version. Using this knowledge, we can manage Node.js dependencies more effectively.