ExpressJS is the most popular framework for creating web applications in less time with fewer lines of code. ExpressJS is built on top of NodeJS, it is minimal, flexible and enhanced NodeJS by providing more advanced features such as controllers, and routers, it also adds multiple middlewares, helping in making the application much more scalable. ExpressJS is also integrated body-parse to parse request body, which handles form or any other incoming data.
Express is best for creating fast and scalable servers, microservices and REST APIs. It is used by many big companies such as Accuweather, Yummly, MySpace, and Accenture.
Express can handle GET and POST requests without installing any third-party module.
GET and POST are the two common HTTP Requests used for building REST APIs. Both of these calls are meant for some special purpose. As per the documentation GET requests are meant to fetch data from specified resources and POST requests are meant to submit data to a specified resource.
This tutorial will teach you the way to handle GET and POST requests in Express.
Express GET Request
Handling GET requests in Express is pretty straightforward. You have to use the instance of Express to call the method Get. The Get method is used to handle the get requests in Express. It takes two arguments, the first one is the route(i.e. path), and the second is the callback. Inside the callback, we send the response when a user requests that path.
Below is the code snippet where we used the Get method to send a string “Hello World!” to respond to the “/” route.
const express = require('express')
const app = express()
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
Output:
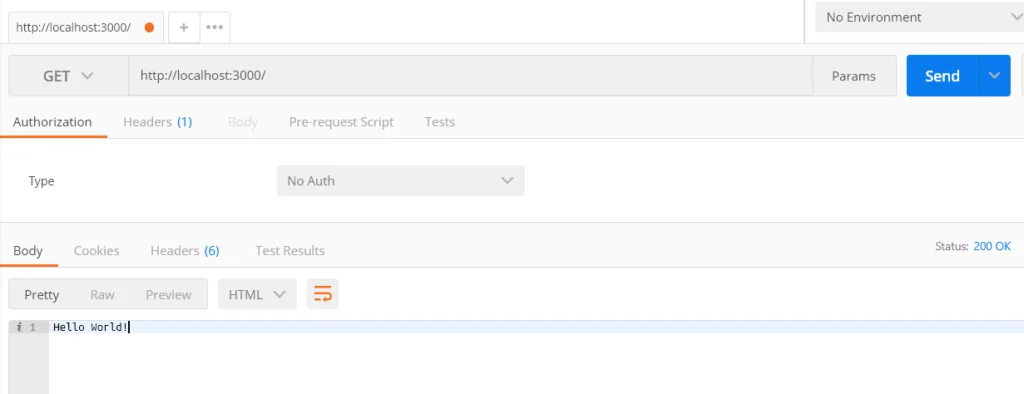
GET requests can be cached and remains in the browser history. This is why the GET method is not recommended to use for sensitive data (passwords, ATM pins, etc). You should GET requests to retrieve data from the server only.
Express POST Request
The POST request is handled in Express using the Post express method. This is used when there is a requirement of fetching data from the client such as form data. The data sent through the POST request is stored in the request object
Express requires an additional middleware module to extract incoming data of a POST request. This middleware is called the “body-parser”. We need to install it and configure it with Express instance.
You can install body-parser using the following command.
npm install body-parser
You need to import this package into your project and tell Express to use this as middleware.
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: false }))
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.post('/', (req, res) => {
let data = req.body;
res.send('Data Received: ' + JSON.stringify(data));
})
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
If you find the above code confusing we have a separate article on Getting Form Data in Node.js, consider reading it.
Output:
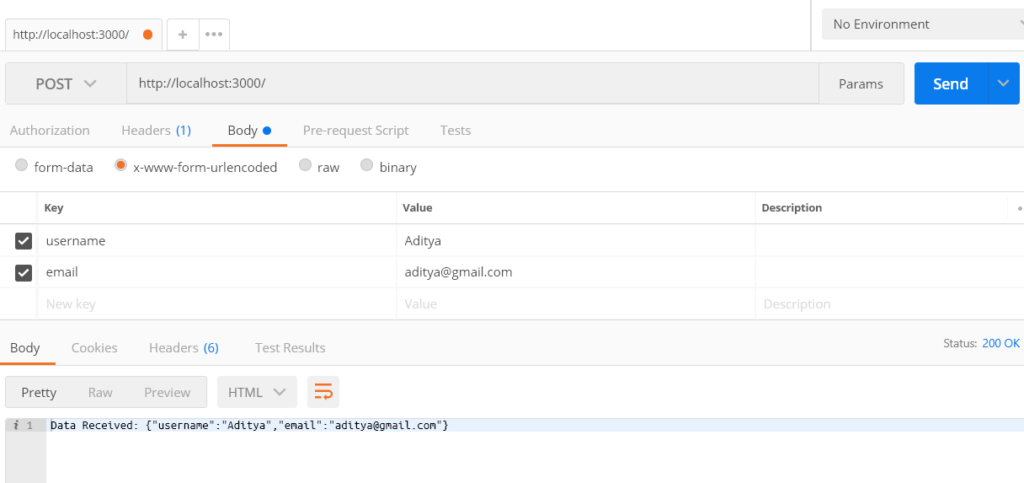
Demo App: Login System
To demonstrate this, let’s code one simple login system that uses a GET request to send a login form to the client and a POST request to fetch the username and password to the server from the client.
Let’s create a new Node.js project.
npm init -y
Let’s install the dependencies.
npm i express body-parser
Next, let’s create an Express web server.
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: false }))
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
Now use the Get method to send the HTML which contains a login form.
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html')
})
When the user clicks on the Login button on the HTML page we will POST the request to the Server and get the response.
app.post('/login', (req, res) => {
let username = req.body.username;
let password = req.body.password;
if (username === 'admin' && password === 'admin') {
res.send('Login successful');
}
else {
res.send('Login failed');
}
})
Here is the complete server.js file code.
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: false }))
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html')
})
app.post('/login', (req, res) => {
let username = req.body.username;
let password = req.body.password;
if (username === 'admin' && password === 'admin') {
res.send('Login successful');
}
else {
res.send('Login failed');
}
})
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
Here is the code of the index.html page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="/login" method="post">
<input type="text" name="username" placeholder="username">
<input type="password" name="password" placeholder="password">
<input type="submit" value="Login">
</form>
</body>
</html>
Run the Server using the command: node server.js
Output:
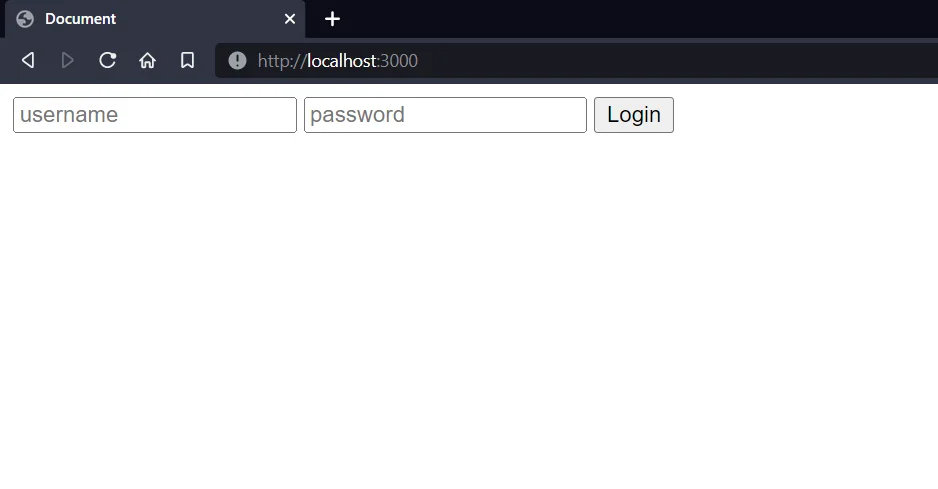
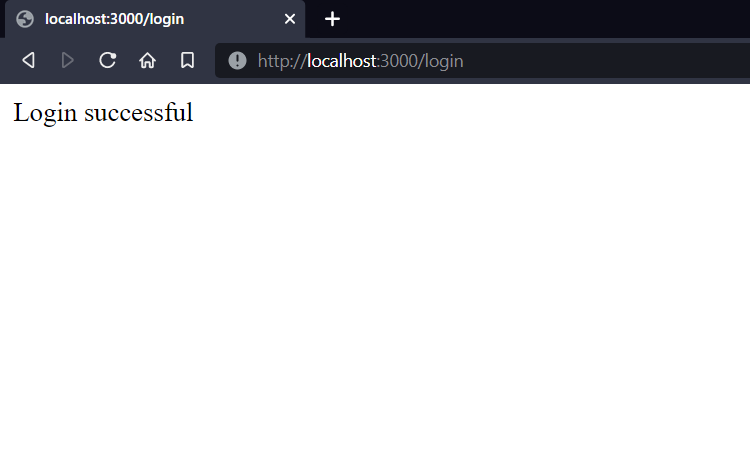
Summary
Express is the most popular framework for creating web applications in Node.js, it requires less time and fewer lines of code. It can able to handle GET and POST requests without installing any third-party module. The GET request can be handled using the Get method from the Express instance, and the POST request is used using the Post method. Hope this tutorial helps you understand how to handle GET and POST requests in Express.
Reference
https://expressjs.com/en/guide/routing.html