There are several reasons why we might want to display file names from a folder in Node.js. Here are a few common use cases:
- Debugging: Printing file names can help us troubleshoot issues related to file system operations or verify if the expected files are present in a directory.
- Directory listing: We might need to generate a list of files in a directory for further processing, such as performing batch operations or generating a report.
In this tutorial, we will see how to display a list of file names from a folder in Node.js using the fs module (file system module) which is one of its internal packages.
Displaying File List from a Folder in Node.js
For displaying the file list from a folder in Node.js we will first start with importing a file system module which has lots of methods such as writing files, removing files, etc then we will see how to write on a file then we will use the path module for writing files in a folder inside the root folder, and then in last, we will display all the files from a folder. Let’s get started.
Step 1: Importing File System Module
The file system module which is also called the fs module is one of the internal packages of Node.js.
Now the question is why we will be importing a file system module. The answer is that we will be utilizing all the methods inside the fs module such as writeFileSync(), readdir(), etc.
Syntax for importing the FS module:
const fs = require('fs');
We have used require for importing the fs module and after importing we are storing it into a constant called fs.
Step 2: Writing Files using FS Module
For writing a file we will use the fs.writeFileSync() method which is used to write the data in a file synchronously.
Syntax of fs.writeFileSync() method:
fs.writeFileSync(filename.extension,data);
where:
- filename.extension is the file name with its extension which we want to write for example apple.txt
- data is the content that is to be present inside the file
Example 1:
In this example, we are writing a single file called apple.txt.
const fs = require('fs');
fs.writeFileSync('apple.txt','a simple file')
We have first created a constant with the name of the fs so that we can import the file system module that means fs.
Then we used the fs.writeFileSync() function for writing a file, there will be two parameters in the fs.writeFileSync() function, the first is the file name apple.txt, and the second parameter is the text inside the file apple.txt which in our case is “a simple file”.
Output:
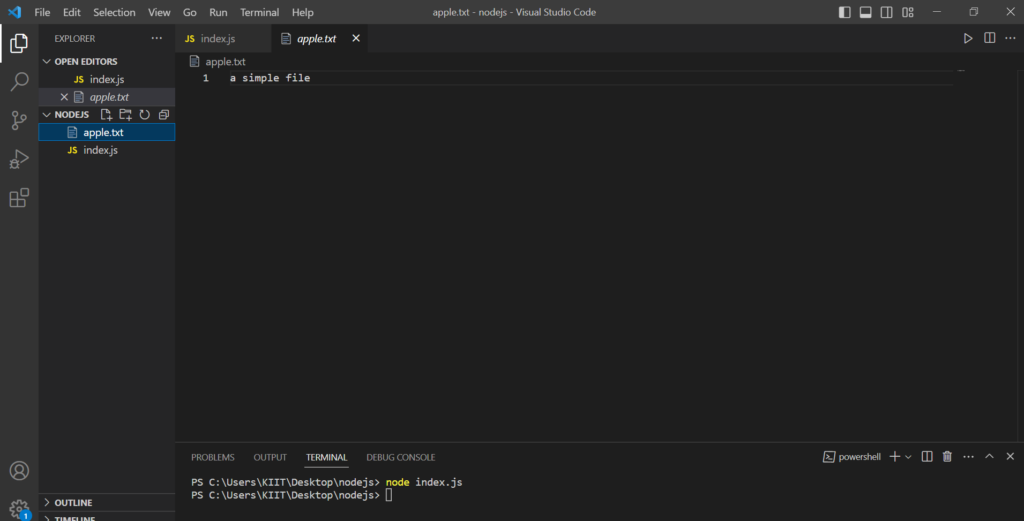
Example 2:
In this example, we are writing five files within the folder NODEJS.
const fs = require('fs');
for(i=0;i<5;i++){
fs.writeFileSync(`hello${i}.txt`,'some simple text in file');
}
We have used for loop for writing more than one file in a folder, the loop starts from i=0 and ends on i<5.
The first parameter is hello${i}, where we have used the $ for creating the dynamic file name. For example hello0.txt, hello1.txt,etc.
The second parameter is some simple text in the file which will be the content for all the files.
Output:
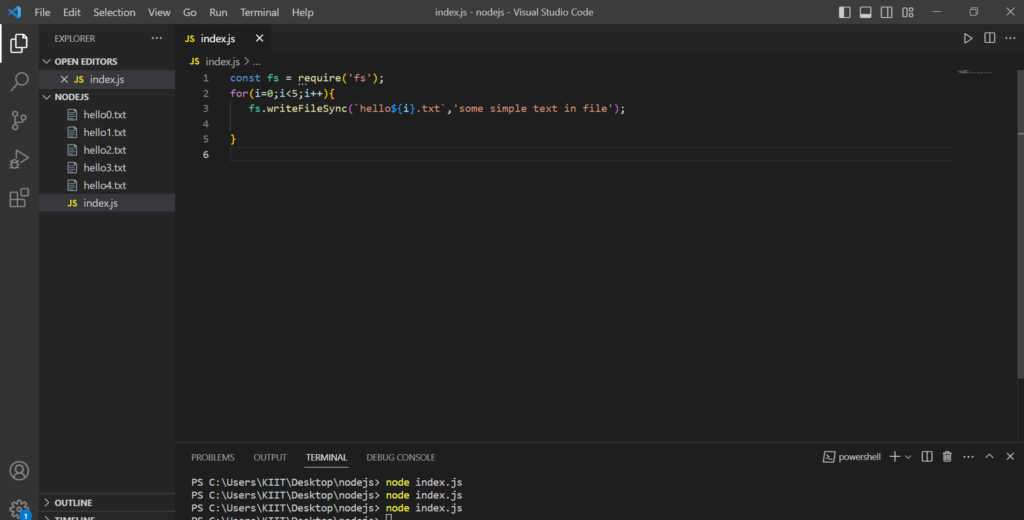
Step 3: Getting the Folder Path
What if we want to create all those files in another folder? Let’s say the files folder which is inside the root folder NODEJS So for that, we will require a path module.
The syntax for importing the Path module:
const path = require('path');
We are using require keyword for importing the path module and then we are storing it into a constant called path.
Example:
In this example, we are creating all the files inside the folder called files which is inside the root folder NODEJS.
const fs = require('fs');
const path = require('path');
const dirPath = path.join(__dirname,'files');
for(i=0;i<5;i++){
fs.writeFileSync(`${dirPath}/hello${i}.txt`,'some simple text in file');
}
We have created a constant with the name of the fs so that we can import the file system module that means fs. Then after that, we created a constant path to require the path module.
We will get the path of our current root directory, NODEJS, with the help of __dirname.
After that, we used the path.join() function for joining the path of the current directory and the path of a folder called files by writing path. join(__dirname, ‘files’). Then store the path of the files folder in the constant dirPath.
Then used the writeFileSync() function for writing dynamic file names with the help of $ in the files folder.
Output:
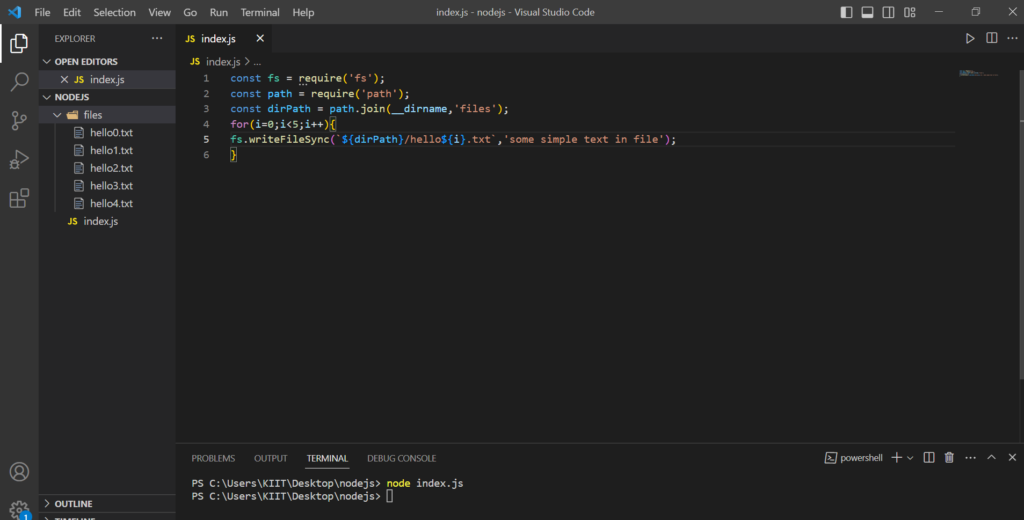
Step 4: Displaying File Names
We will display the file names in two steps, first, we will read the directory of the files and then will print the file names.
So why are we reading the directory? It is because we have to print files which is present inside the particular directory.
Syntax for displaying file names:
fs.readdir(dirPath,(err,files)=>{
console.log(files);
})
readdir() is a function used for reading a directory. and by using console.log(files) we are printing the files or we are displaying the name of the files in the console.
Example:
In this example, we are displaying the files from a folder called files.
const fs = require('fs');
const path = require('path');
const dirPath = path.join(__dirname,'files');
fs.readdir(dirPath,(err,files)=>{
console.log(files);
})
Output:
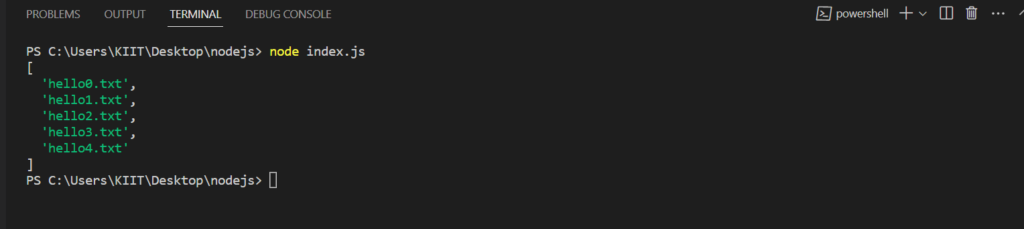
Summary
In this tutorial, we have learned how we can import the file system module and then we have explored how to write a single file and multiple files with data inside a folder using a loop. We also explored the path module and its syntax to get the folder path, then finally we came to know about display file names by reading a directory of files and then printing it. After reading this tutorial, we hope you can easily display the file list from a folder in Node.js.