Writing files is a fundamental operation in the development of full-fledged web applications. For instance, applications like WhatsApp, write user data, such as chats, in a file that can be downloaded upon request. In Node.js, this operation can be easily done in a few lines of code using the FS module.
In this tutorial, we will learn to write to a file using FS module methods fs.writeFile() and fs.writeFileSync(), one is asynchronous whereas the other is synchronous in nature.
FS Module to Writing Files in Node.js
Writing files in Node.js can be done using a built-in core module called the FS module. The FS module stands for file system module which comes with many synchronous and asynchronous methods used to read and write files.
To use the file system module’s methods to write to a file, we must first import it in Node.js.
Syntax:
Below is the syntax to import the file system module in Node.js.
const fs = required('fs')
In Node, it usually recommends using Asynchronous methods. Asynchronous means non-blocking i.e. the method does not block the execution of other methods or operations. But sometimes we want a Synchronous approach such as we want to block other methods or operations until a file is written so in this case it is recommended to use a Synchronous method. That’s why in this tutorial we will have a look at both ways.
Methods to Write Files in Node.js
- fs.writeFile(): This method is used to write the data of a file asynchronously.
- fs.writeFileSync(): This method is used to write the data of a file synchronously.
Now let’s have a look at both methods one by one.
Also Read: Node FS Module: Working With Files on NodeJS
Writing a File Asynchronously in Node
You can write to files asynchronously without blocking the other part by using the fs.writeFile() method. Since this is an asynchronous method, it takes a callback as an argument in which we can handle if an error occurs, print a success message, or perform any other operation after the file writing is complete.
Syntax:
fs.writeFile(file, data, [options], [callback])
Parameters:
- filename – (string or Buffer): The path of the file to be written.
- data – (string or Buffer): The content to be written to the file.
- options – (object):
- encoding – (string, optional): The encoding of the file. Default is ‘utf8’.
- flag – (string, optional): The file system flag. Default is ‘w’.
- callback – (function, optional): The callback function is called after the file is written or an error occurs.
Return: none
Example:
const fs = require('fs');
const data = 'This content is to be written to the file.';
// Write data to the 'sample.txt' file
fs.writeFile('sample.txt', data, (err) => {
// callback body
if (err) {
console.error(err);
return;
}
console.log('File written successfully.');
});
If the file already exists in which you want to write, this method simply writes data to it, but if the file does not exist, it automatically creates a new file with the specified file name.
Output:
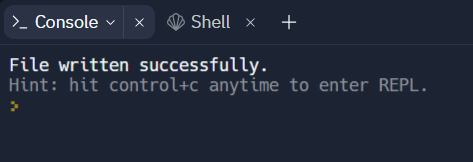
Here, the file exists at the specified file path, so it simply rewrites the data passed as an argument to the file.
Note: If you don’t want to remove the old content, you can use the fs.appendFile() method, which appends the new content at the end of the file.
Writing a File Synchronously in Node
The writeFileSync() method comes into action whenever there is a need to block the execution of the rest of the program until the file writing is complete.
Syntax:
fs.writeFileSync(file, data, [options])
Parameters:
- filename – (string or Buffer): The path of the file to be written.
- data – (string or Buffer): The content to be written to the file.
- options – (object):
- encoding – (string, optional): The encoding of the file. Default is ‘utf8’.
- flag – (string, optional): The file system flag. Default is ‘w’.
Return: none
Example:
const fs = require('fs');
const data = 'This content is to be written to the file.';
try {
// Write data to the 'sample.txt' file synchronously
fs.writeFileSync('sample.txt', data);
console.log('File written successfully.');
} catch (err) {
console.error(err);
}
To validate the output, you can read the file manually using the readFile() or readFileSync() method.
Output:
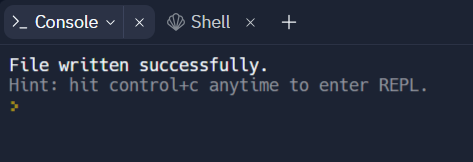
Here, the new file is created using the fs.writeFileSync() method as it does not pre-exist in the specified directory. Also, the file content is the same as passed as an argument to this method.
Conclusion
In this tutorial, we have explored different ways to write data to a file using both async and sync methods. If you are doing an operation that needs to block the execution of other blocks of code and let them execute only after the file is written then you should use the writeFileSync() method.
On the other hand, if you don’t want to block the execution of other programs while the file is being written, you can of course go with the writeFile() method. We hope you have gained enough knowledge to write the data to the file using the Node.js File System module.
Reference
https://nodejs.org/api/fs.html