In JavaScript, a multiline string allows us to write text in multiple lines and display it. This makes our code easy to read and avoids unnecessary repetition of display function code. It is quite useful when there is a need to handle vast sections of text or documentation and to reduce the need to break the text into separate lines.
Before we get into multiline strings, let’s first quickly revise strings in JavaScript.
Strings in JavaScript
A string is a sequential collection of characters. It can be any text item, like a word, phrase, sentence, or paragraph. Let’s discuss how we can input, store, and display strings in Javascript.
Writing Single-Line Strings in JavaScript
When a text is processed by a single line of code, then it is a single-line string. There are three ways in which we can represent a string in Javascript code. We usually enclose the text inside a single quote, a double quote, or a backtick to specify that it is a string.
Single Quotes:
One way to specify a string in Javascript is to keep the text inside a single quotation mark (‘ ‘).
let sentence = 'This is a string enclosed in single quotation mark.';
console.log(sentence);

Double Quotes:
Similarly, we can represent a string inside double quotation marks(” “). It works in the same way as single quotes.
let sentence = "This is a string enclosed in double quotation mark.";
console.log(sentence);

Backticks:
The best way to define a string is to write the text inside the backticks in this way:
let sentence = `This is a string enclosed in backticks.`;
console.log(sentence);

This technique of specifying a string is also known as a template literal. It is the most common method used in the manipulation of strings.
Problem with Writing Multiline Strings
Let’s say we want to list the steps for baking a cake, with each step on a different line. Based on what we have learned so far, you might do it like this:
let title = 'Steps to bake a Cake!';
let step1 = 'Melt butter and mix it with sugar and vanilla essence.';
let step2 = 'Add flour,baking soda and cocoa powder with milk.';
let step3 = 'Mix the ingredients together and form a thick batter with a flowing consistency.';
let step4 = 'Transfer it to a baking can and bake.';
console.log(title);
console.log(step1);
console.log(step2);
console.log(step3);
console.log(step4);

But look closely at the code. Do you think it’s professional to write code this way? Don’t you think it has too many variables and console statements? This code can be made cleaner, and more organized, let’s see how.
Also Read: How to Convert Object into String in JavaScript?
Best Method to Create Multiline Strings in JavaScript
There are three best possible ways in which you can join multiple single-line strings into one multi-line string.
1. Creating Multiline Strings Using +
The ‘+’ operator helps us to add or concatenate two or more single-line strings into one multiline string. Here are a few demonstrations of how you would do this using the ‘+’ operator.
First, let’s directly use the ‘+’ operator:
We can enclose every string line inside the quotation (may it be single or double) and then join all of them using the ‘+’ operator by inserting it in between the joining of two lines, like the following:
let title = 'Steps to bake a Cake!';
let steps = 'Melt butter and mix it with sugar and vanilla essence.' +
'Add flour,baking soda and cocoa powder with milk.' +
'Mix the ingredients together and form a thick batter with a flowing consistency.' +
'Transfer it to a baking can and bake.';
console.log(title);
console.log(steps);

This code joins all the lines into one multiline string, but it looks a bit odd because they’re all on a single line. We can place each line on a new line using the newline (\n) operator.
Adding New line Character (\n):
The new line character helps us change lines and makes the string appear on a new line. Here is how we place it:
let title = 'Steps to bake a Cake!';
let steps = 'Melt butter and mix it with sugar and vanilla essence.\n' +
'Add flour,baking soda and cocoa powder with milk.\n' +
'Mix the ingredients together and form a thick batter with a flowing consistency.\n' +
'Transfer it to a baking can and bake.';
console.log(title);
console.log(steps);
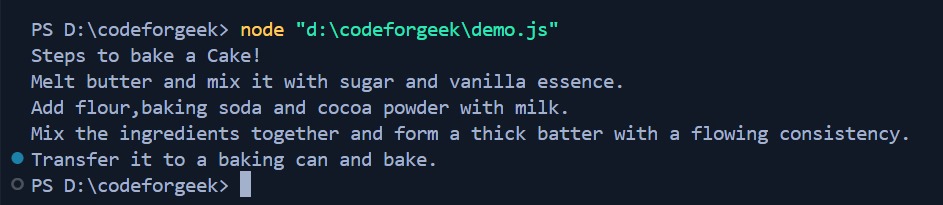
Implementing Escape Character (\):
An escape character is a backslash (\) used in strings to allow special characters. It helps us to include apostrophes (’) and quotation marks in our strings.
Suppose we want to have an apostrophe inside a string, like – Is’nt it tasty? then we would write:
let title = 'Steps to bake a Cake!';
let steps = 'Melt butter and mix it with sugar and vanilla essence.\n' +
'Add flour,baking soda and cocoa powder with milk.\n' +
'Mix the ingredients together and form a thick batter with a flowing consistency.\n' +
'Transfer it to a baking can and bake.\n' +
'Voila we are done! Is'nt it tasty?';
console.log(title);
console.log(steps);
This causes errors:
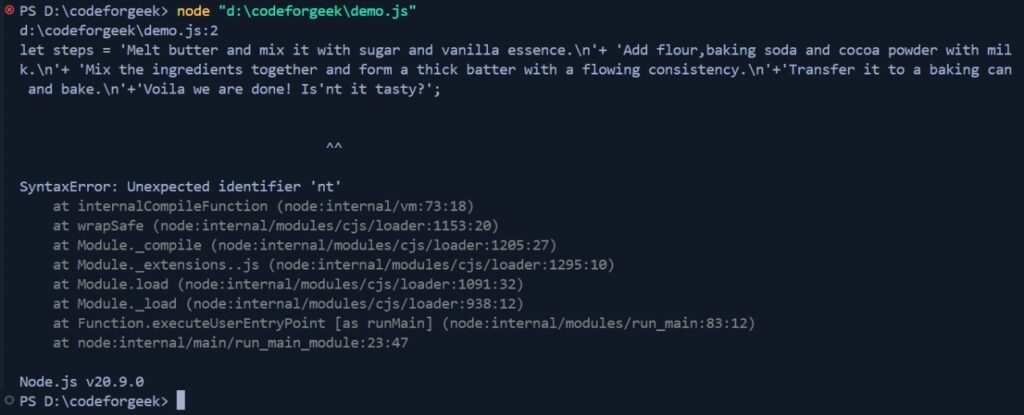
To resolve this, we can add an escape character just before the apostrophe like this:
let title = 'Steps to bake a Cake!';
let steps = 'Melt butter and mix it with sugar and vanilla essence.\n' +
'Add flour,baking soda and cocoa powder with milk.\n' +
'Mix the ingredients together and form a thick batter with a flowing consistency.\n' +
'Transfer it to a baking can and bake.\n' +
'Voila we are done! Is\'nt it tasty?';
console.log(title);
console.log(steps);
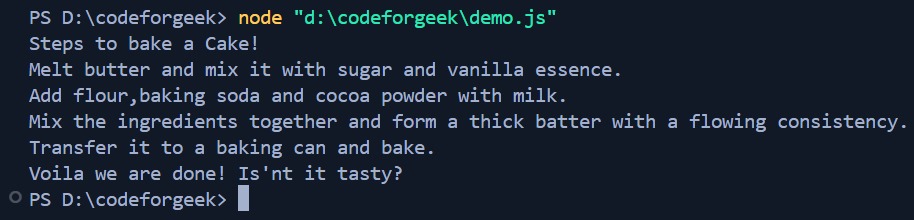
2. Creating Multiline Strings Using \
Using the \ operator is also a way to create multiline strings without using the ‘+’ operator.
let title = 'Steps to bake a Cake!';
let steps = 'Melt butter and mix it with sugar and vanilla essence.\n\
Add flour, baking soda, and cocoa powder with milk.\n\
Mix the ingredients together and form a thick batter with a flowing consistency.\n\
Transfer it to a baking pan and bake. Voila, we are done! Isn\'t it tasty?';
console.log(title);
console.log(steps);
The backslash (\) is used at the end of each line to indicate that the string continues on the next line.
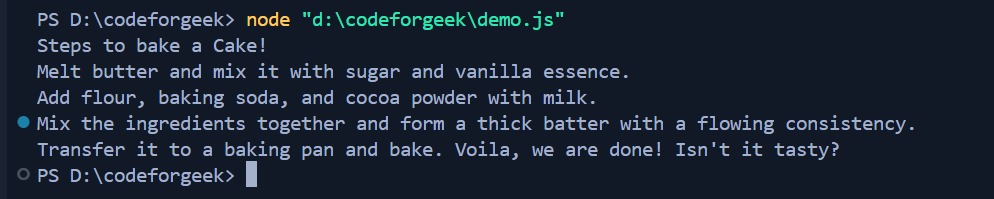
3. Creating Multiline Strings Using Template Literals
Template literals are the easiest and simplest way to create a multiline string. All you have to do is enclose the complete text inside one backtick enclosure.
let title = 'Steps to bake a Cake!';
let steps = `Melt butter and mix it with sugar and vanilla essence.
Add flour, baking soda, and cocoa powder with milk.
Mix the ingredients together and form a thick batter with a flowing consistency.
Transfer it to a baking pan and bake.
'Voila', we are done! Isn't it tasty?`;
console.log(title);
console.log(steps);
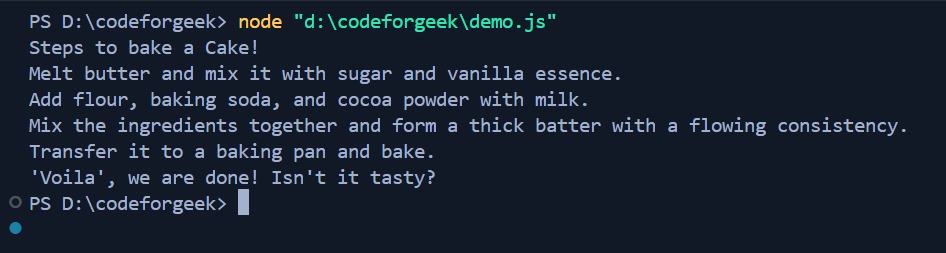
No \n for new lines, no \ before any special character.
Conclusion
Multiline strings are good because they make long strings more readable when we break them into more than one line. It helps when the strings are long such as in SQL queries, JSON data, etc. HTML content can also be easily created by inserting expressions and variables directly into the string using backticks (`) without concatenation.
To learn about string interpolation in JavaScript, click here.
Reference
https://stackoverflow.com/questions/64347350/how-to-create-multiline-string-in-js