A controller is a function that defines the response sent to a client when making a request to the server.
This tutorial teaches you the concept of controllers in NodeJS. We highly recommend you read the previous tutorial Separating Routers in NodeJS to better understand the Controllers.
Controllers in NodeJS
Below is the code that contains a router for the home page of a website.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send("Home");
});
app.listen(3000, () => {
console.log("listening on http://localhost:3000");
});
This router uses the get() method which takes a path and a callback function as an argument. The path is a route to which a client can make a get request which activates the callback function and execute the code inside it.
Callback inside the get method can be used to define certain functionality and send some response in return to the request made by the client so here the callback passes inside these request methods called a controller.
Separating Controllers from Routers
The advantage of separating controllers from routers is that they can use again and again in multiple routers by just using their names. So instead of defining the whole callback function in separate routers we can define a single controller and use it inside multiple routers.
Below is the code where we have separated the controller from the router.
const express = require('express');
const app = express();
const home = (req, res) => {
res.send("Home")
};
const about = (req, res) => {
res.send("About")
};
const contact = (req, res) => {
res.send("Contact")
};
app.get('/', home);
app.get('/about', about);
app.get('/contact', contact);
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Run the Code:
To run the above code, create a project folder, and copy and paste the above code into a JavaScript file “app.js”.
Then locate the folder in the terminal and execute the below command to install the Express module.
npm install express
Then execute the below command to run the code.
node app.js
Open the Application:
Enter the below URL inside the search bar of a web browser to open the application.
http://localhost:3000/
Output:
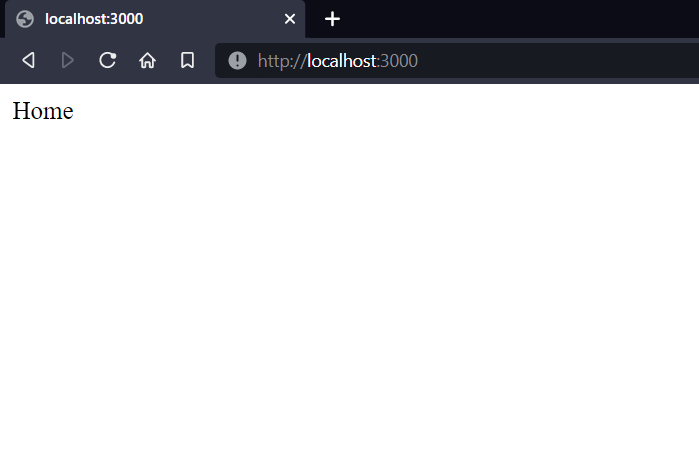
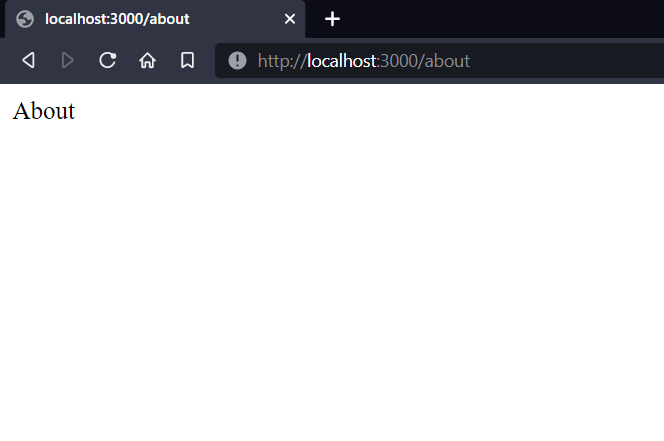
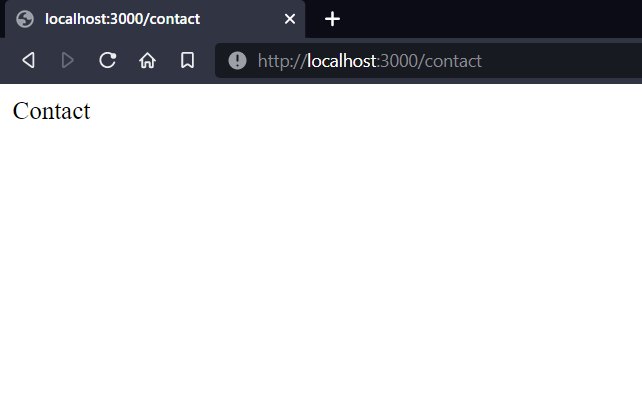
Separate Controllers in Individual Files
To ensure efficient routing and a clear, easy-to-debug code, website developers are separating controllers into individual files. This helps to avoid complex, cluttered code and makes it easier to understand and debug.
Steps to Separate Controllers
Below is the step-by-step guide to separate the controllers into individual files.
Step 1: To separate them into individual files, create multiple files for each of them.
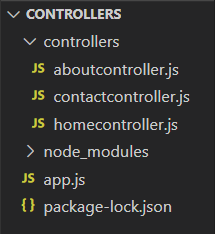
Step 2: Copy and paste the controller from “app.js” to their individual files and replace their names with “module.exports” to export them in order to import them in the “app.js” file.
homecontroller.js
module.exports = (req, res) => {
res.send("Home")
};
aboutcontroller.js
module.exports = (req, res) => {
res.send("About")
};
contactcontroller.js
module.exports = (req, res) => {
res.send("Contact")
};
Step 3: Import controllers and use them inside “app.js”
const express = require('express');
const home = require('./controllers/homecontroller')
const about = require('./controllers/aboutcontroller')
const contact = require('./controllers/contactcontroller')
const app = express();
app.get('/', home);
app.get('/about', about);
app.get('/contact', contact);
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Output:
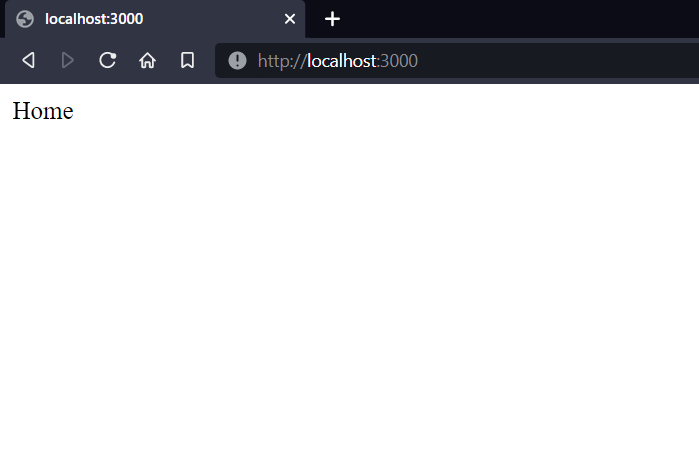
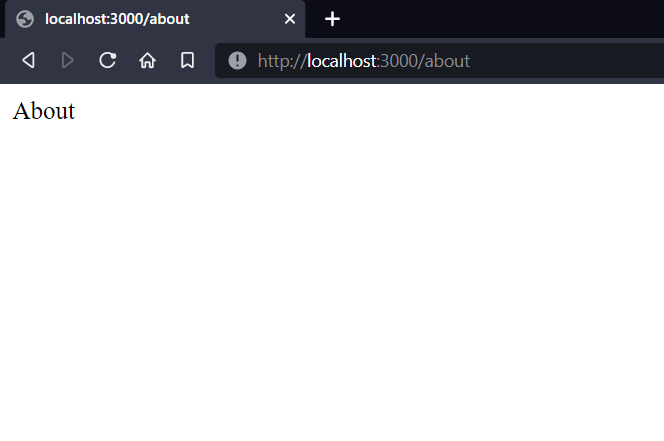
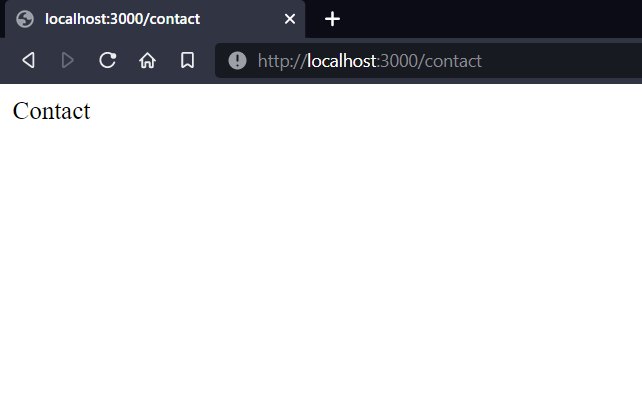
Here we get the same output as in the previous example, which means the controller works the same after separating into individual files.
Summary
Controllers are essential components of NodeJS applications, as they are responsible for defining the responses to requests from Routers. By separating Controllers from Routers, developers can keep their applications tidy and well-organized, which will make debugging and maintenance easier. With a better understanding of Controllers and how to use them, developers can create more powerful and efficient NodeJS applications.
Reference
https://developer.mozilla.org/en-US/docs/Learn/Server-side/Express_Nodejs/routes