A website has multiple pages which can be accessed using endpoints, a endpoint has two parts the URI and the HTTP request method such as get, post, etc. For example, for opening a website home page clients have to make a get request to the URI “https://domain/home” or “https://domain/”. The server sends the response page according to the endpoints. To define the response for different endpoints routers are used. There are many routers in an application which makes the overall application complex and hard to debug, it is a good approach to separate them into individual files to make the overall code organized.
In this tutorial, you will learn about the process to separate routers into multiple files.
Introduction to Routers
Routers are used to define the functionalities for multiple types of requests a client can make on different routes to send the response accordingly.
There are many types of request client can create for an endpoint such as a get, post, delete, etc. In routers, we have to use different methods which specify the request type for example when a client does a “get” request on the URI “https://domain/login” then we have to send a login form but if the request type is “post” rather than “get” for the same URI means the client this time sending the response after filling the form, so we have to handle that separately in another router.
It might sound confusing for beginners, but as you see an example it will become clear, it’s a very easy concept.
Example:
Below is an example where we have set up a route for a get request to the home page and send a message “Home” as a response.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send("Home");
});
app.listen(3000, () => {
console.log("listening on http://localhost:3000");
});
Output:
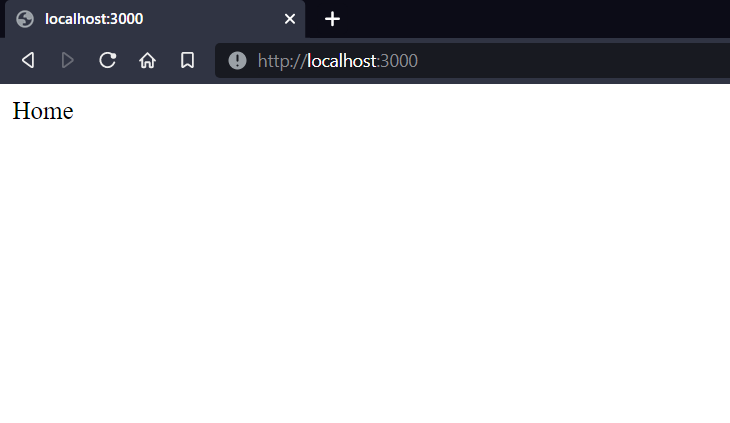
We also have a separate tutorial on Routers if you want to read it.
Create Different Routers
Different Routers are created for distinct endpoints. The instance of express has methods for handling different types of HTTP requests like get(), post(), etc. These methods take a path, and a callback function to send the response.
Example:
In the below example, we have set up multiple routers which send different responses depending upon the endpoint.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send("This is a get request");
});
app.post('/', (req, res) => {
res.send("This is a post request");
});
app.get('/about', (req, res) => {
res.send("This is a get request for the about page");
});
app.post('/about', (req, res) => {
res.send("This is a post request for the about page");
});
app.listen(3000, () => {
console.log("listening on http://localhost:3000");
});
Run the Code:
To run the above code, create a project folder, and copy and paste the above code into a JavaScript file “app.js”.
Then locate the folder in the terminal and execute the below command to install the Express module.
npm install express
Then execute the below command to run the code.
node app.js
Test the Application:
Open postman and enter the below URL inside the search bar.
http://localhost:3000
Perform different types of requests to the different paths to see the distinct response.
Output:
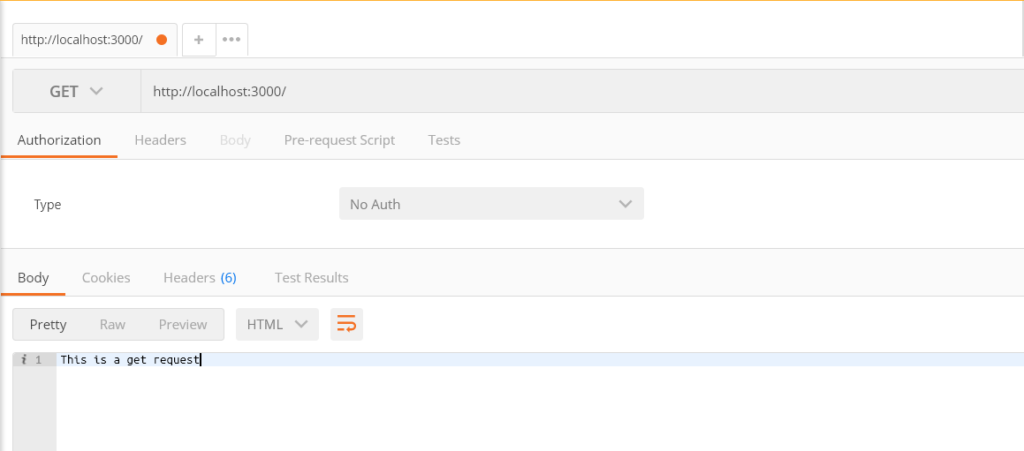
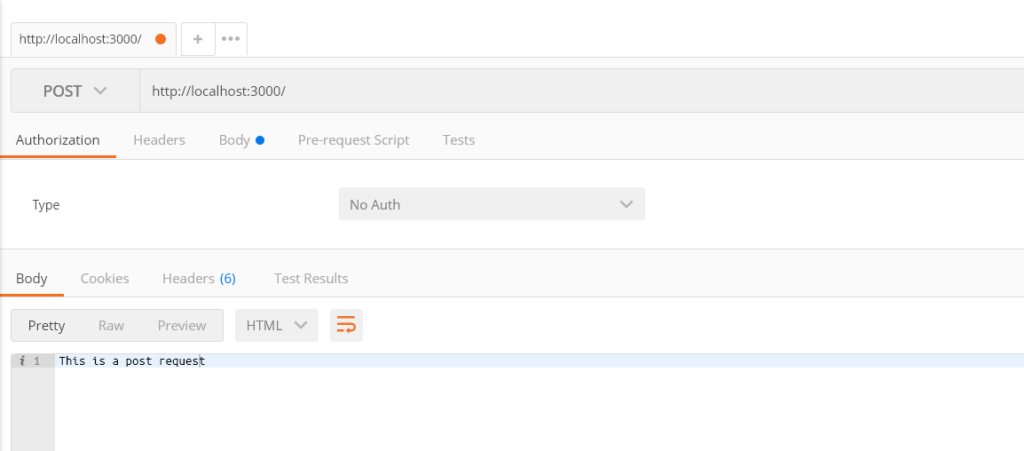
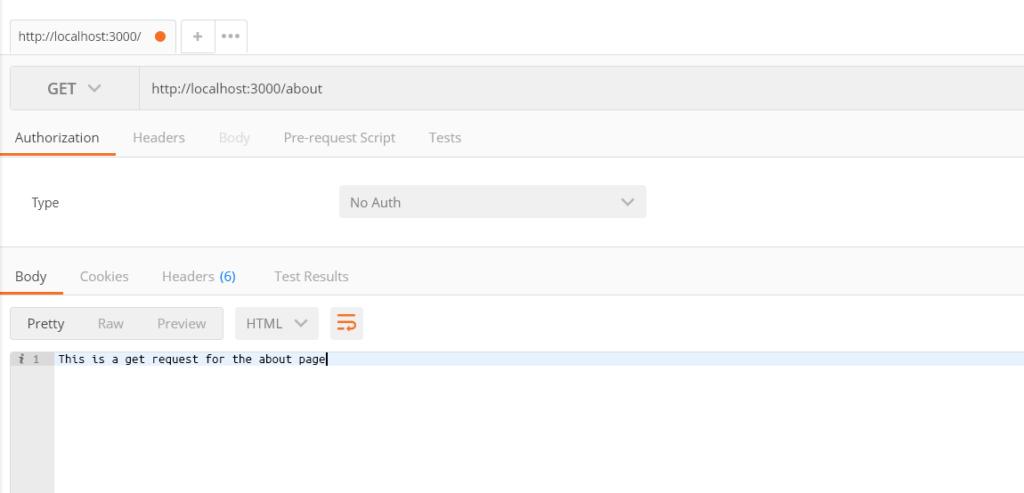
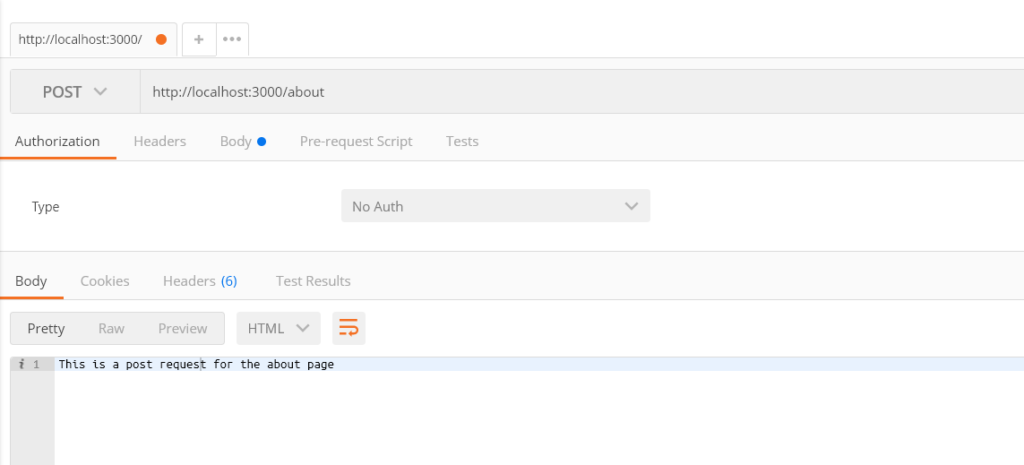
Separating Routers into Individual Files
Suppose a website has thousands of endpoints, and it is complicated if write them in a single file, so we can separate them into individual files and then use them inside the main server file.
Steps to Separate Routers in NodeJS
Step 1: Create a project folder with a file “app.js” and type the below command to install Express Module.
npm i express
Step 2: Create a folder “routers” inside the project folder, and create three files homerouter.js, aboutrouter.js, and contactrouter.js.
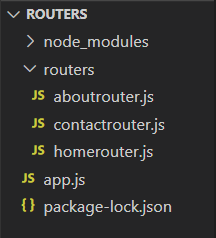
Step 3: Inside each file, import the Router from the express, create an instance “router”, create Routers using that instance, and export it.
homerouter.js
const { Router } = require('express');
const router = Router();
router.get('/', (req, res) => {
res.send("Hello From Home Router");
});
module.exports = router;
aboutrouter.js
const { Router } = require('express');
const router = Router();
router.get('/about', (req, res) => {
res.send("Hello From About Router");
});
module.exports = router;
contactrouter.js
const { Router } = require('express');
const router = Router();
router.get('/contact', (req, res) => {
res.send("Hello From Contact Router");
});
module.exports = router;
Step 4: Inside the “app.js” file import the Express module and routers from their separate file, create an instance of Express then use the use() method from that instance for each router to activate them then use the listen() method that takes a PORT as an argument to run the application.
const express = require('express');
const home = require('./routers/homerouter');
const about = require('./routers/aboutrouter');
const contact = require('./routers/contactrouter');
const app = express();
app.use(home);
app.use(about);
app.use(contact);
app.listen(3000, () => {
console.log("listening on http://localhost:3000");
});
Run The Application:
Type the below command on the terminal to run the application’s server.
node app.js
Open the Application:
Enter the below URL inside the search bar of a web browser to open the application.
http://localhost:3000/
Output:
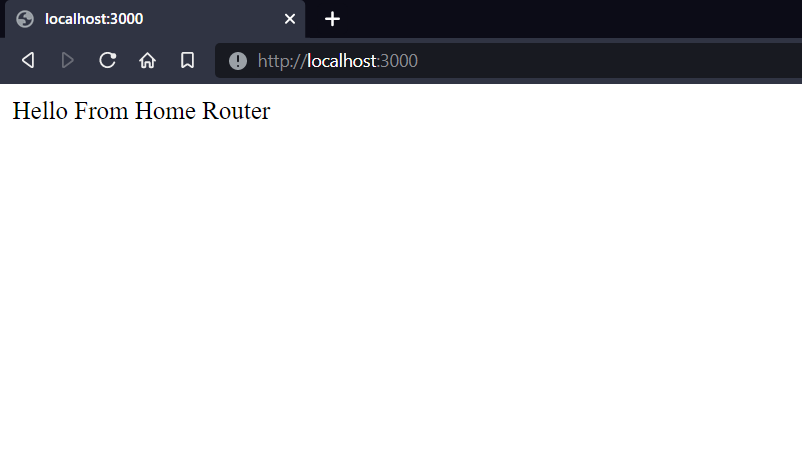
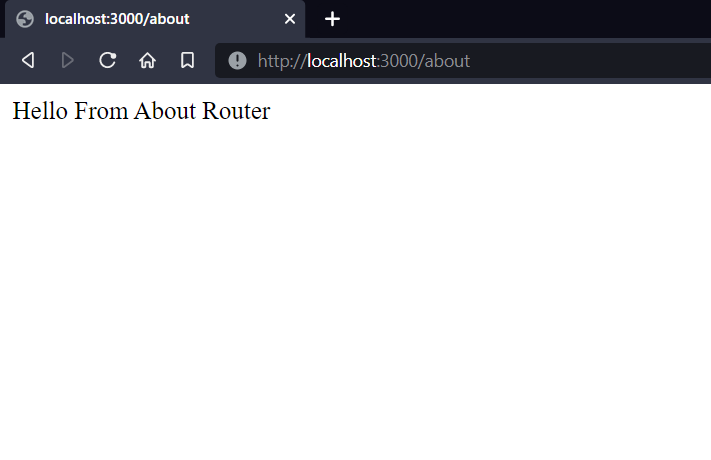
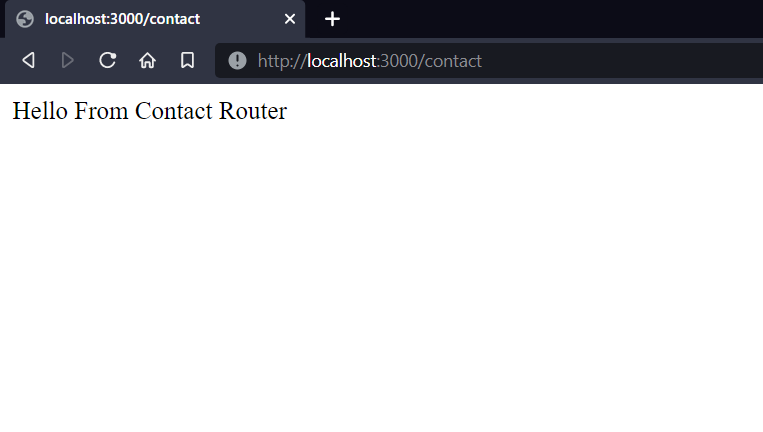
Summary
Routers are a way to handle different types of requests for different routes. There are many routers used in an application so it is a good approach to write them separately to organize the code. Hope this tutorial helps you to learn about the routers in NodeJS.
Reference
https://stackoverflow.com/questions/34174115/express-separate-route-and-controller-files
https://developer.mozilla.org/en-US/docs/Learn/Server-side/Express_Nodejs/routes