Recently I was working on a large Node.js project having a huge number of libraries installed and since the project is on a production server, it is quite difficult for me to see the libraries my team installed.
Due to reasons like errors or compatibility issues, we also want to see the version of a specific library. So I have come up with some simple commands as solutions that help to get the list of npm packages installed in Node.js. Let’s begin with an introduction to npm before moving on to the commands for listing libraries.
NPM (Node Package Manager)
NPM (Node Package Manager) is a powerful tool to install, manage, and share packages. It has an online repository of thousands of Node.js packages that can be used for many purposes. These packages are free to install and can be used to create different types of applications. For example, Express is a package used to write Node.js efficiently, Mongoose is used to connect with the MongoDB database with few configurations, and so on.
While working with NPM it is important to keep track of the modules we have installed in our project. Listing out these modules can be a good way to keep track of them. Let us now see how.
List NPM Installed Packages Using Command Line
NPM packages can be installed both globally (meaning packages can be accessed throughout all projects on our system) and locally (packages can only be accessed in the project directory where they have been downloaded) on our system. How we can list locally and globally installed npm packages is quite similar.
List Locally Installed Packages
NPM packages that have been locally for a specific project are present inside the node_modules folder under the project’s root directory.
To list locally installed packages we first navigate to our root directory and use the command:
npm list
NPM modules and dependencies are installed in a tree structure. The depth=0 flag is used to signify that only packages at the top level of the tree should be listed. If the depth=1 flag is used it lists all packages at the top level of the tree and their immediate dependencies.
Command to list surface-level packages:
npm list --depth=0
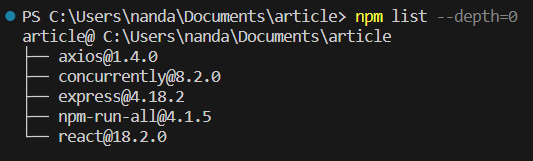
Command to list top-level packages along with their immediate dependencies:
npm list --depth=1
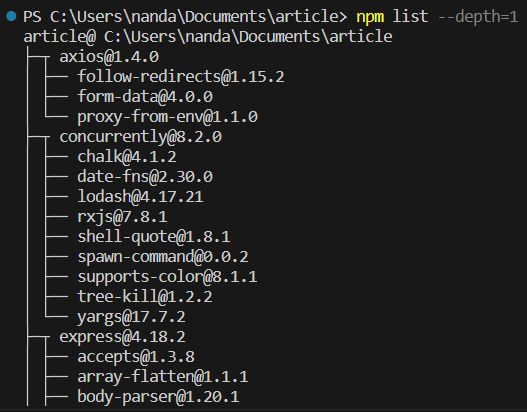
List Globally Installed Packages
NPM packages that are installed globally throughout our system can be listed by using the -g (which denotes global packages) flag with the npm list command.
Command to get a list of globally installed packages:
npm install -g
The depth=0 and depth=1 flags can also be used here as with local packages.
List Installed NPM Packages Using Code
The above method uses the command line to get a list of globally and locally installed packages, but what if you need to get the list programmatically? Well, a not-so-straightforward approach that involves programming is using the child_process module.
We can install the child_process module using the following command:
npm install child_process
After installation, the execSync method from the child_process module can be imported into our file using require. This method is used to synchronously run shell commands from the Node.js script.
const { execSync } = require('child_process');
const cmd = 'npm list --depth=0 --json';
const output = execSync(cmd);
const data = JSON.parse(output);
const packages = Object.keys(data.dependencies);
console.log(packages);
In the above code, the constant cmd is used to set the command that we want to run on our terminal. ‘npm list –depth=0 –json’ indicates that we want to list all packages at the top level of the package tree and return this data in JSON format using the –json flag. We use the execSync method to execute the command stored in cmd on the shell and then the output is stored in the output constant.
JSON.parse() is used to convert the output from a JSON string to a JavaScript object and is stored in the data constant. The data returned contains a property called dependencies which contain the name of the packages installed, which can be accessed using Object.keys() and stored in the packages variable.
At last, we use console.log() to print the list of packages on the console.
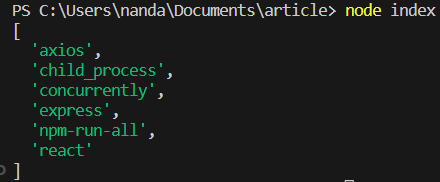
Want to learn more about the Child Process module? Click here to read.
Frequently Asked Questions (FAQs)
What Is an NPM Package?
NPM package is a third-party module present in the NPM online repository. This package can be installed to import various functionalities written by someone else in our code. An NPM package can be installed both locally and globally.
What Is a Global Install?
If you install a package using the “npm install” command with the “-g” flag, it is a global install, meaning the package can be accessed from anywhere in the system and you can use it in any directory directly from the command line.
What Is a Local Install?
If you install a package using the “npm install” command without the “-g” flag, it is a local install, this is done when the package is not needed in the future or in any other program.
Where Do Global NPM Packages Install?
The global npm packages are installed inside a global node_modules folder that can be accessed from everywhere, no matter the location in the system. The path of the global node_modules folder depends on the operation system. For example in Windows you would find it at “C:\Users\<username>\AppData\Roaming\npm\node_modules”, and in macOS/Linux it is at “/usr/local/lib/node_modules”.
How Do I List Global Packages in NPM?
To list all NPM packages installed globally in your system, you can run the command “npm list -g”.
Conclusion
In this article, we have seen two different ways of listing locally and globally installed NPM packages. If you are allowed to use the command line you should use the direct approach of running the “npm list” command.
On the other hand, if you need to list the libraries using code, you can use the second approach which is using the child_process module.
You can use these methods to maintain an efficient development environment by removing unnecessary dependencies if you find any in the output list.
If you want to know how to do this, check out – Removing NPM Packages and Dependencies in Node.js (npm uninstall)
Reference
https://stackoverflow.com/questions/17937960/how-to-list-npm-user-installed-packages