We all know JavaScript and how good it is for both client-side and server-side. But then why are we writing this tutorial on TypeScript? Why is TypeScript gaining popularity even when JavaScript is here and constantly updating?
What is TypeScript, why do we need it, how does it differ from JavaScript, and how to get started using it? We will explain all of these in this article. Let’s get started.
Introduction to TypeScript
TypeScript is a programming language created by Microsoft as a superset of JavaScript. It introduced static typing allowing us to add types which is lacking in JavaScript. Beyond several other features that it adds, this is one of the most important ones that make error handling easier than ever.
Static typing means the variable types are defined at compile-time instead of runtime to make sure that the type errors are caught during the coding not during execution for error-free execution.
Why Not Just JavaScript?
JavaScript is a loosely typed language which means here we do not define the data type during variable initializing or for parameters, that’s why the type mismatch can lead to errors. For example, someone creates a function sum() which can only take numbers but since JavaScript is not statically typed, it allows you to call that function by passing any data type, even strings which results in wrong output and error as well.
If the function is an external function imported from a module or third-party libraries, it can be even difficult to know what parameters type it takes and we have to go through the official documentation or get help online for that.
So here comes TypeScript by which we can specify the types of data and it also gives proper errors when the types do not match.
// JavaScript Code
function addNumbers(a, b) {
return a + b;
}
console.log(addNumbers(5, "10")); // This will return 510 (but no error)
// TypeScript Code
function addNumbers(a: number, b: number): number {
return a + b;
}
console.log(addNumbers(5, "10")); // TypeScript Error: Argument of type 'string' is not assignable to parameter of type 'number'.
Apart from this, JavaScript also lacks modern programming language features that TypeScript has, let’s look at them next.
Key Features of TypeScript
TypeScript is specially designed to provide the following features:
- Static Typing: By introducing static types, TypeScript helps us to catch errors that occur due to type mismatch.
- Class-Based Objects: TypeScript provides true support for classes, not like JavaScript which uses a prototype-based approach. TypeScript lets us create classes, constructors, implement inheritance and even let us access modifiers like public, private, and protected.
- Modularity: TypeScript supports modularity so that we can divide our code into reusable components to increase reusability.
- ES6 Syntax: TypeScript uses the same ES6 concept as in JavaScript so if you are familiar with JS syntax like arrow functions, template literals, etc, you can do all that too in this.
- Compatibility with JS Environments: TypeScript code compiles into plain JavaScript, making it compatible with any JavaScript environment.
- Other Features: TypeScript provides modern language features like interfaces, enums and generics, making it a better option for creating large-scale applications.
How to Use TypeScript?
To use TypeScript, we can use NPM. NPM is a package manager that comes with Node.js. Whenever you install Node.js, it automatically gets installed as well. NPM is used to install external packages from the npm registry.
Step 1: Check for Node.js and NPM
Make sure you have Node.js installed as well as NPM by running the below commands:
node -v
npm -v
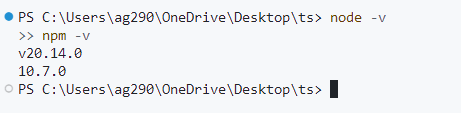
If you get their respective version numbers, it is installed. If not, click here to learn how to install them.
Step 2: Install TypeScript
Now, execute the below command to install TypeScript globally.
npm install -g typescript

Globally, it means it can be accessed from anywhere.
Step 3: Verify TypeScript Installation
Now you can run the below command to check if TypeScript is installed:
tsc -v

If this gives a version, it means it is installed successfully.
Step 4: Create a Project Folder
Now create a new project folder and run the below command to initialize npm:
npm init -y
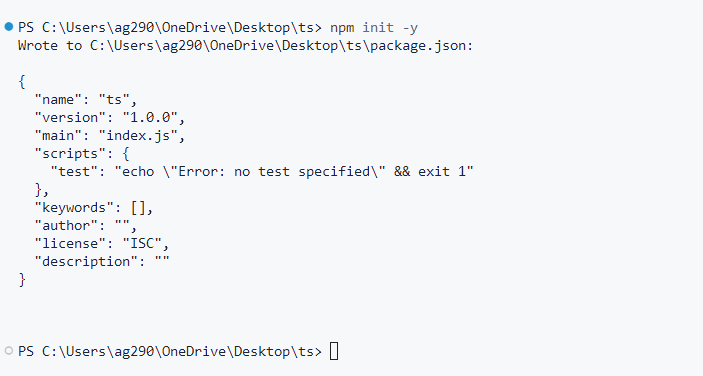
Step 5: Create a TypeScript File
Create a new file with the extension .ts (e.g., hello.ts) and write the below code:
let message: string = "Hello, World!";
console.log(message);
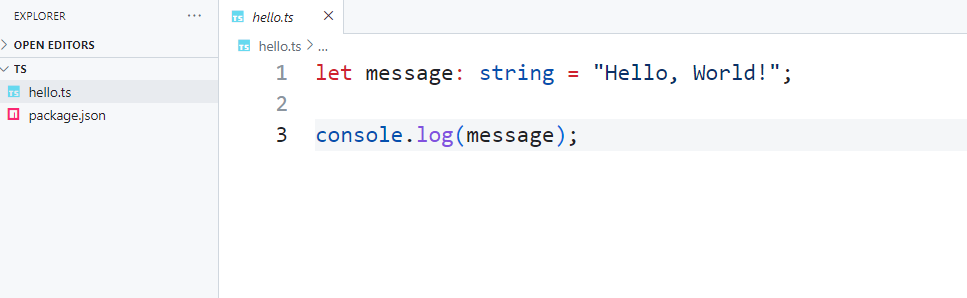
Step 6: Compile TypeScript to JavaScript
Now to compile this code, open the terminal, navigate to the project folder, and run the below command:
tsc hello.ts
This will generate a hello.js file.
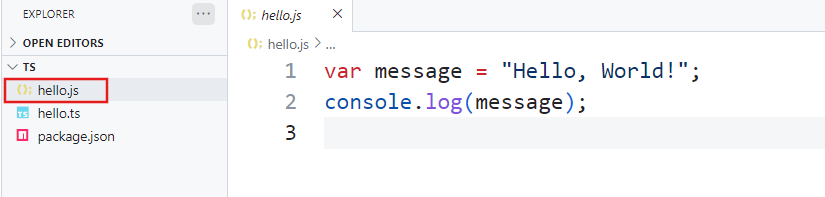
Step 7: Run the JavaScript File
Now we have to run the generated JavaScript file with:
node hello.js

Yes, we can’t directly compile TypeScript code, it first compiles into JavaScript. With third-party libraries like Next.js, there is no need for any additional compilation as it configures it on its own.
Summary
In short, TypeScript is a strict syntactical superset of JavaScript which adds new features and static typing. Static typing means the variable types are defined at compile-time rather than runtime to catch errors while coding.
Using TS is quite easy, all you have to do is have NPM and you can install it directly from the NPM registry by running the command “npm install -g typescript”.
Read this to avoid TypeScript-related errors in the future: How to Fix “Unknown file extension .ts” Error in ts-node
Reference
https://www.typescriptlang.org