TypeScript, identified by the “.ts” file extension, enhances JavaScript by introducing static typing. But problems might occur if you use programs like “ts-node”, which are meant to run TypeScript files directly in a Node.js environment. The Unknown file extension “.ts” error occurs when a TypeScript file is not properly configured.
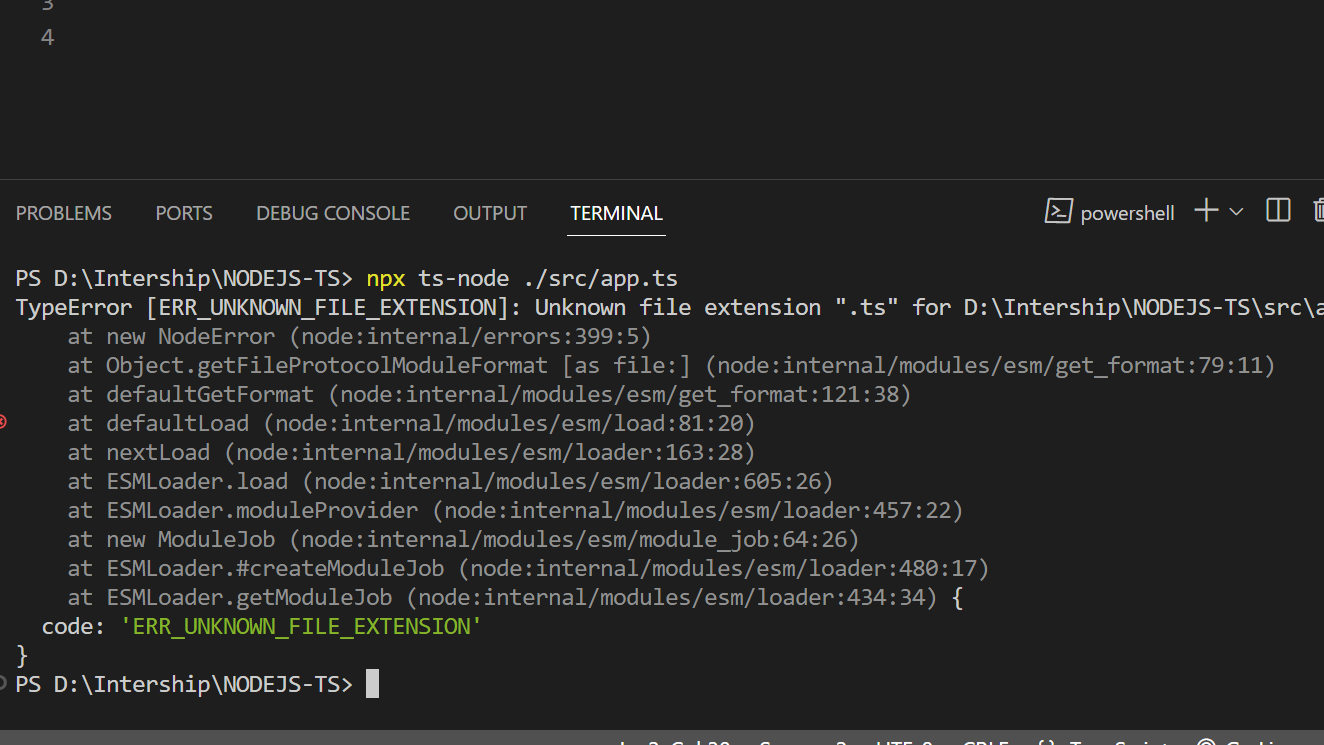
In this article, we will explore several common solutions to address this error.
Resolving Unknown file extension .ts Error
Let’s see the troubleshooting steps to resolve this error.
Method 1: Using “–esm” Option
In “ts-node”, the Unknown file extension “.ts” problem arises when your “package.json” file has “type”: “module” specified and by default, “ts-node” only recognizes the “commonjs” module.
To fix it, run the TypeScript file with “ts-node –esm my-file.ts”. Using the “–esm” flag is an easy way to fix the problem. When using the “–esm” flag, “ts-node” is directed to handle TypeScript files as an ECMAScript module.
ts-node --esm my-file.ts
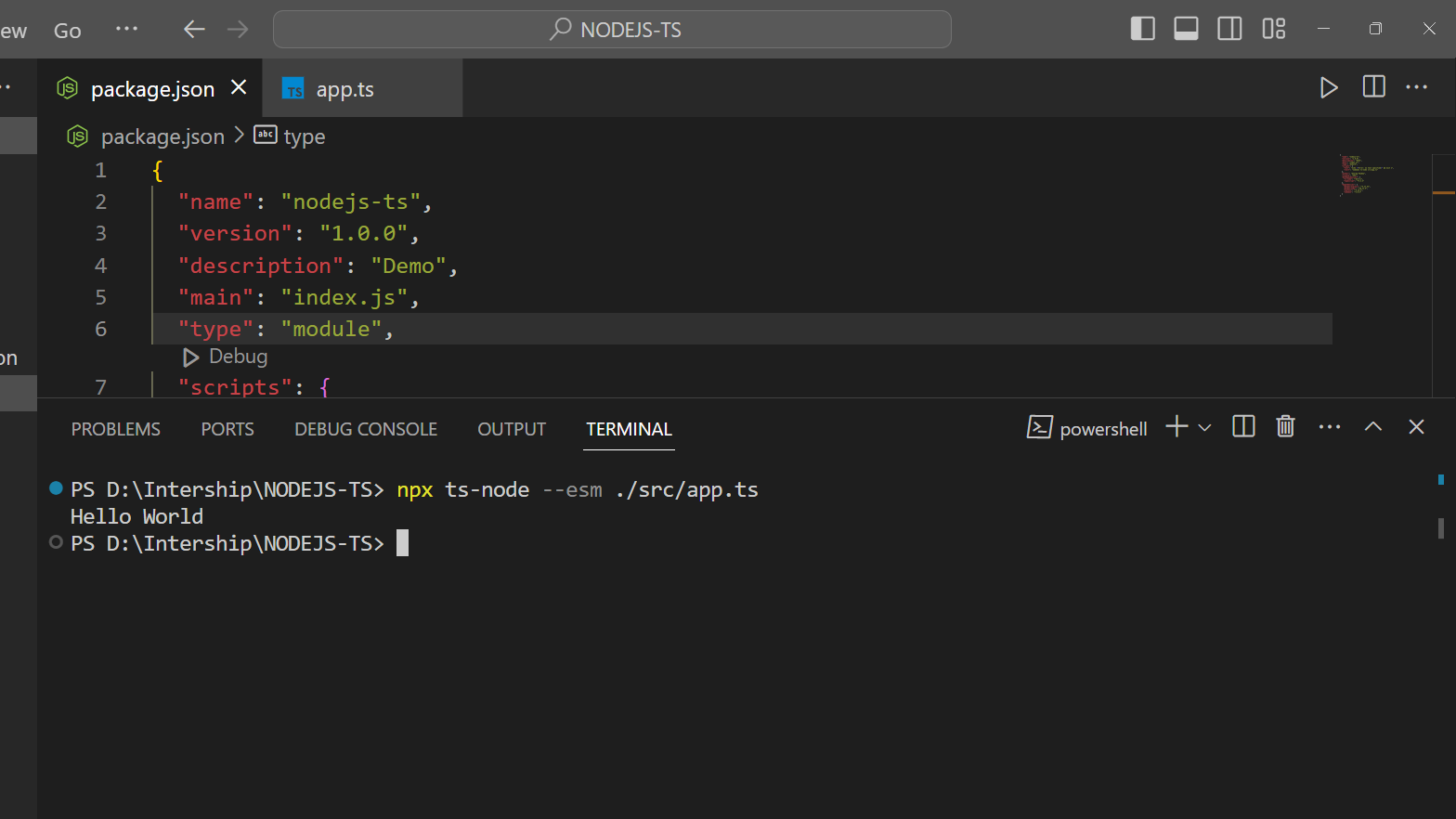
This command “npx ts-node –esm ./src/app.ts” makes it easier to run TypeScript files that use the syntax of ECMAScript. This allows you to use import and export statements to organize your modules. This command is very helpful for applications that use ECMAScript and TypeScript modules.
Note: You can define a dev script in your “package.json” file to not have to remember to set the “–esm” flag every time.
"scripts": {
"dev": "ts-node --esm my-file.ts"
},
By using this script object, you may avoid having to repeatedly memorize and type the flags and have a centralized, consistent manner to do all of your development-related tasks.
Method 2: Remove “type”: “module” Property
Changing the type attribute to” commonjs” or removing it from your “package.json” file is another way to fix the problem.
All “.js” files in the project are seen as ES modules when the “type” attribute is set to the “module”, which might cause an issue because “ts-node” could anticipate the “CommonJS” module by default.
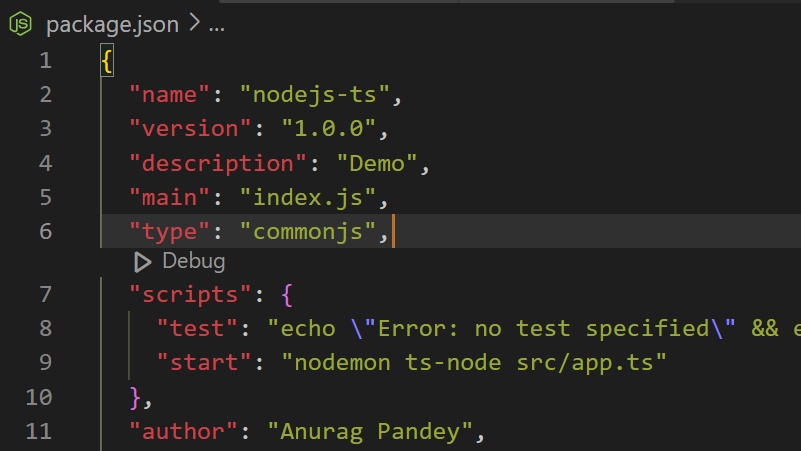
If “type”: “module” is changed, it means that CommonJS modules, not ECMAScript modules, are being used in your project. This change might be necessary if you encounter compatibility issues.
Method 3: Enable the ES Modules
Another useful way to fix issues and allow TypeScript files to be used with “ts-node” without using the “–esm” argument is to update the “tsconfig.json” file.
To update inside the “tsconfig.json” file, you may specifically modify the “ts-node” option:
"ts-node": {
"esm": true,
"experimentalSpecifierResolution": "node"
},
In this configuration:
- “esm”: true instructed ts-node to treat TypeScript files as ECMAScript modules.
- “experimentalSpecifierResolution”: “node” enables module resolution in TypeScript, which can help fix problems arising from module imports.
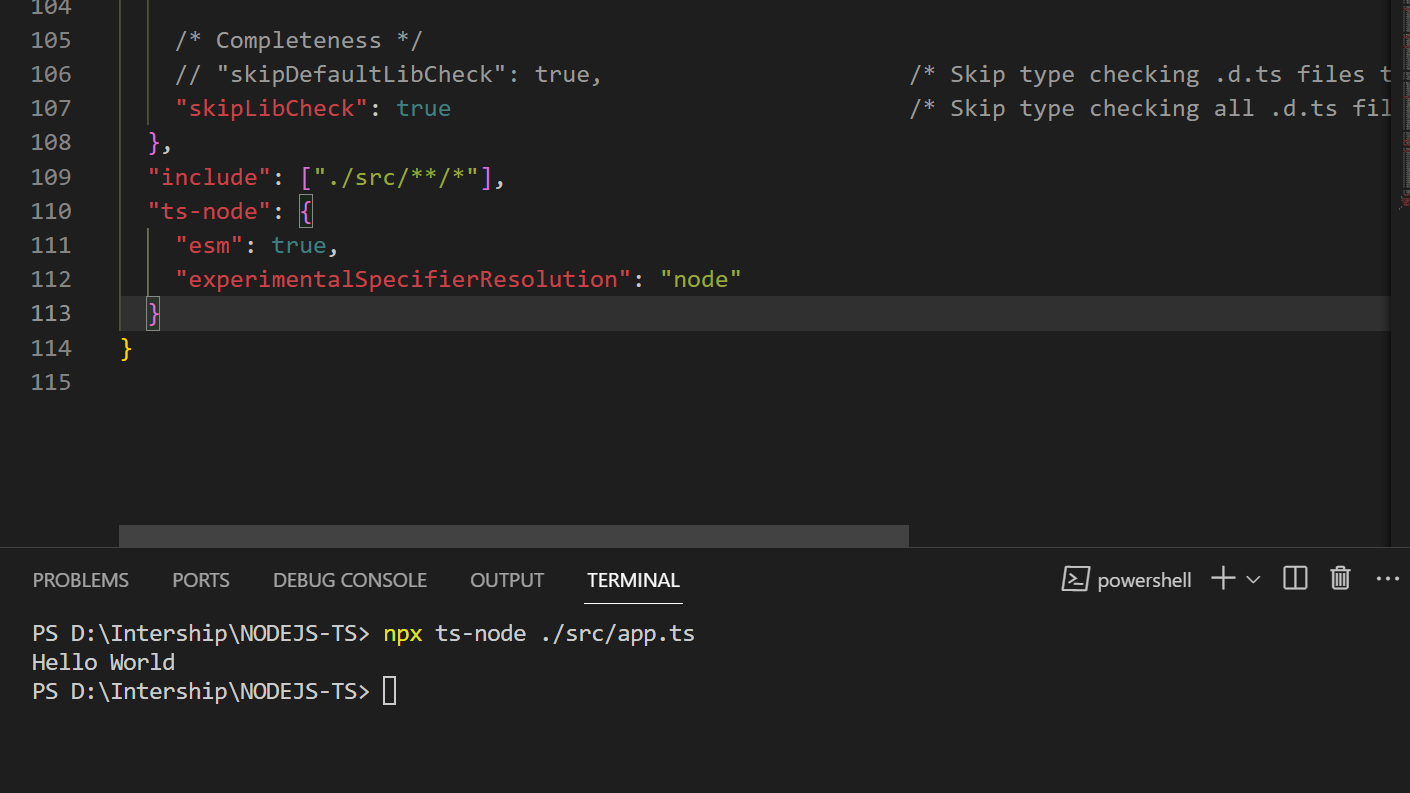
Making changes to the “tsconfig.json” file is a useful approach for allowing TypeScript files to function with “ts-node” without depending on the “–esm” parameter.
Method 4: Compile TypeScript Files into JavaScript
You can prefer not to use “ts-node” and would rather use “tsc“(TypeScript Compiler) to transpile TypeScript files and then “node” to run the generated JavaScript files.
The “tsc“ command is the shorthand for the TypeScript Compiler, which is the official compiler for TypeScript. Code written in TypeScript is translated into JavaScript using it. The “node” command is used to execute JavaScript code in a Node.js runtime environment.
You may follow these steps:
Step 1: Run TypeScript Compiler – To use the TypeScript Compiler to transpile your TypeScript files into JavaScript, run the following command in your terminal:
tsc
This will transpile the TypeScript files in the given include directory using the configurations from your “tsconfig.json” file.
Note: The “.js” file is located in the specified “–outDir”.
Step 2: Run Node – you can execute the generated JavaScript files using Node. For example:
node dist/your-file.js
Adjust the file path according to your project structure.
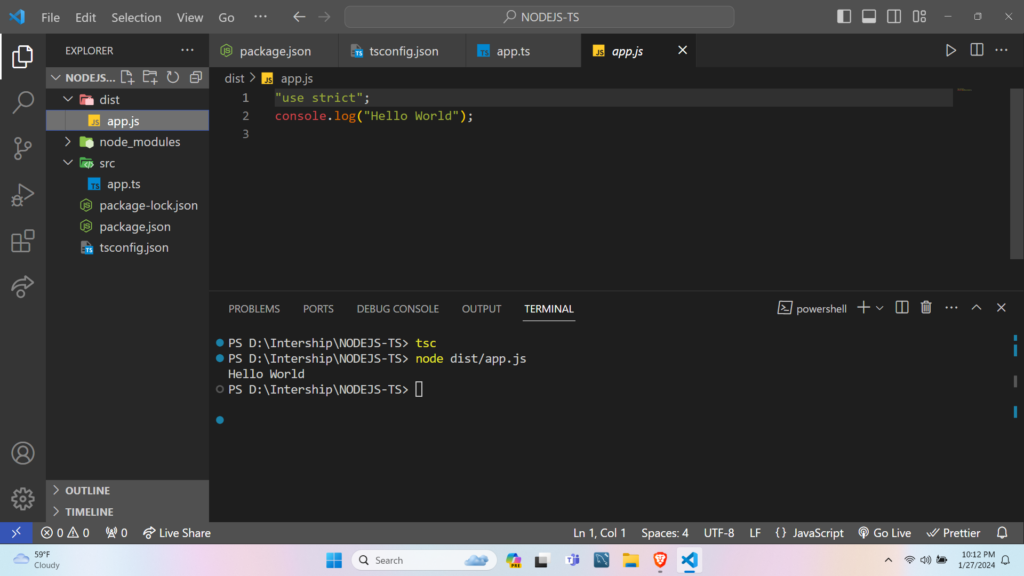
Lastly, we can also write a start NPM script that sequentially executes the “node” and “tsc” commands:
"scripts": {
"start": "tsc && node dist/app.js"
}
When you use “start”: “tsc && node dist/app.js”, it will run the TypeScript compiler (tsc) and then immediately run the generated JavaScript file (app.js).
If you want to dive deeper into TypeScript with Node.js and Express.js, we also recommend reading – How to use TypeScript in NodeJS and ExpressJS Project
Conclusion
In conclusion, dealing with TypeScript files in a Node.js project frequently results in the “Unknown file extension .ts” issue, particularly when utilizing tools like “ts-node”. This error in your Node.js and TypeScript project may be fixed by using the above solutions to make sure your TypeScript files are recognized and run properly. Select the strategy that best meets the needs of your project.
Continue Reading – Troubleshooting ReferenceError: fetch is not defined
Reference
https://github.com/TypeStrong/ts-node/issues/1997