The pandas.melt() function in the Pandas library is used for reshaping or transforming DataFrames. It is particularly useful for converting wide-format DataFrames into long-format ones. The pandas.melt() function essentially “unpivots” a DataFrame, making it more suitable for certain types of analyses and visualizations
In this article, we will first go over the syntax and parameters of this method. After that, we’ll use a few examples along with the implementation to explain each of the parameters for the pandas.melt() function.
Also Read: Getting Number of Rows and Columns in Pandas DataFrame (3 Methods)
Syntax of pandas.melt() Function
The wide format is where each column represents a variable, and each row contains a unique observation, while the long format involves melting or unpivoting the DataFrame to have a more tabular structure with identifier variables and values.
Syntax:
pandas.melt(frame, id_vars=None, value_vars=None, var_name=None, value_name='value', col_level=None)
Parameters:
- frame: The DataFrame to be melted.
- id_vars: Columns to be retained as identifier variables (not melted).
- value_vars: Columns to be melted. If not specified, uses all columns not set in id_vars.
- var_name: Name to be used for the variable/column name. If not specified, uses the existing column name.
- value_name: Name to be used for the value/column name. The default is ‘value’.
- col_level: If columns are MultiIndex, use this level to melt.
Implementing pandas.melt() Function in Python
After knowing the syntax and the parameters of the pandas.melt() function let’s move on to implementing it.
For implementing pandas.melt() function let’s first create a sample DataFrame.
import pandas as pd
df = pd.read_csv("weather.csv")
df
Here we first imported the pandas as pd, then created the DatFrame using a CSV file, the CSV file name is weather.csv, and then printed the DataFrame.
Output:
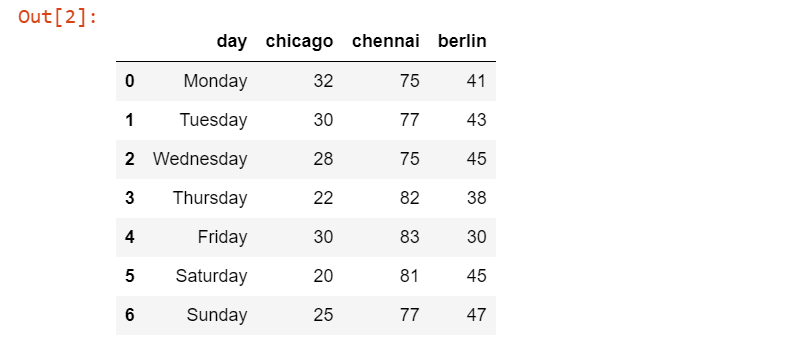
Let’s now implement pandas.melt() function in different scenarios by passing different parameters into it for the above Pandas DataFrame.
Example 1: Applying pandas.melt() Function Directly on the DataFrame
In this example, we will directly pass the above-created DataFrame df into the pandas.melt() function and save the result into the variable df1.
df1 = pd.melt(df)
df1
Output:
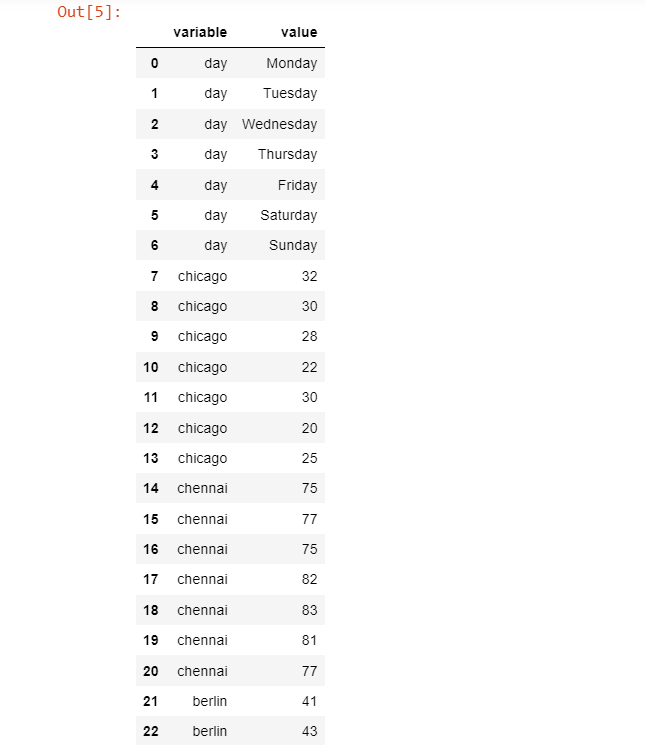
We can see that our DataFrame df got changed from a wide format to a long format.
Example 2: Passing Parameter ‘id_vars’
In this section, we are going to use pandas.melt() function to melt our DataFrame df from wide to long by passing parameters frame and id_vars.
df1 = pd.melt(df, id_vars=["day"])
df1
Here we have called the pandas.melt() function using the Pandas library which we imported as pd. This function takes two arguments, the first argument that it takes is the DataFrame df and the second argument is id_vars as day, the id_vars are the things that we want in the x-axis means the day column will be intact and it will not be transformed.
Output:
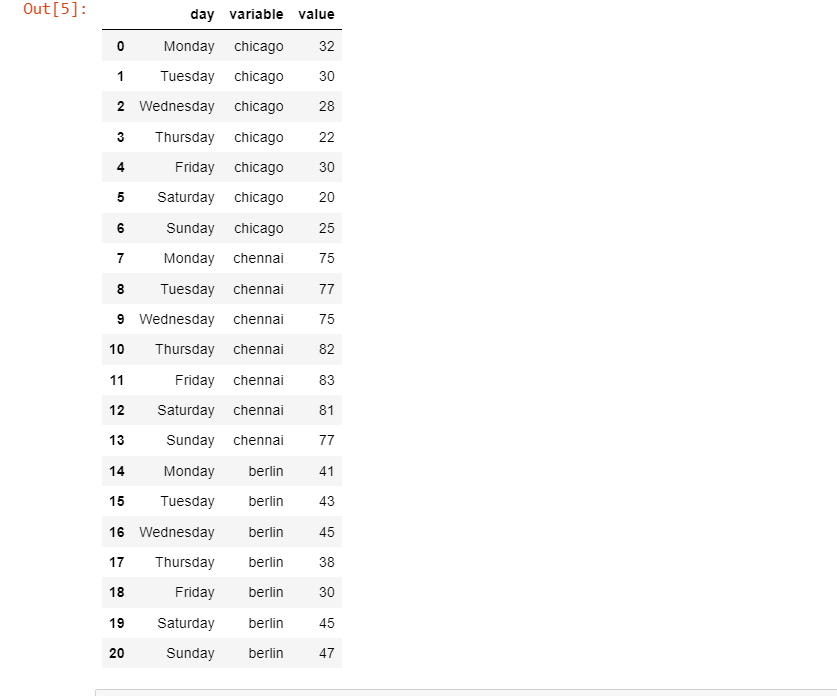
In the above output, we can see the DataFrame df got transformed into a format where the cities are now actually inside the rows.
Example 3: Changing the Variable and Value Names
In the above examples, we can see that we got all the cities in the column whose name is variable and all the temperatures in the column whose name is value. So these are pretty boring column names and they should be changed to something like a city for the column name variable and the column name value should be replaced with the name temperature. So we can do this by using other arguments of pandas.melt() function which are var_name and value_name.
df1 = pd.melt(df, id_vars=["day"], var_name="city",value_name="temperature")
df1
Here we have passed the parameter var_name means the variable name as the city and parameter value_name means the value name as the temperature.
Output:
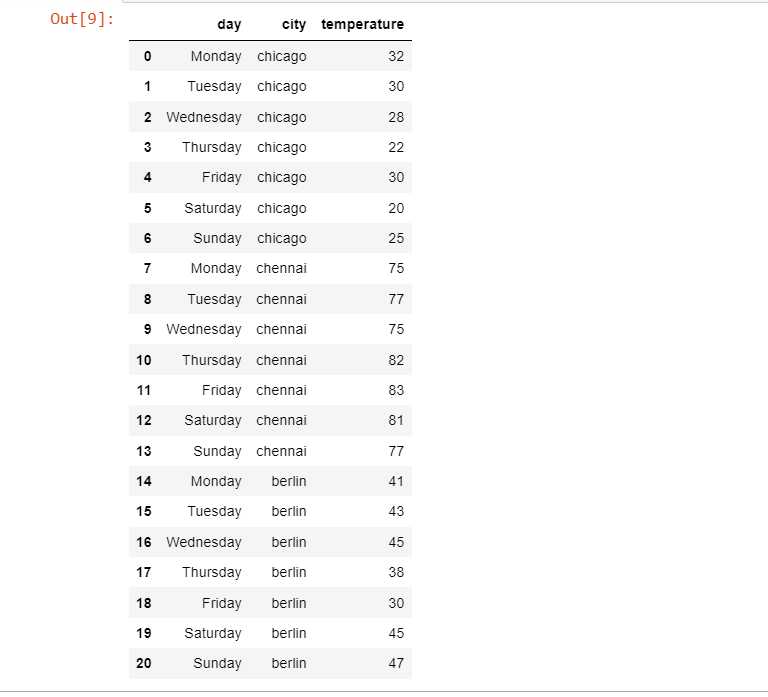
In the above output, it clearly can be seen city is written in place of the variable and place of the value now the temperature is written.
Summary
The pandas.melt() function is a versatile tool for reshaping DataFrames and is commonly used in data cleaning and preparation workflows, especially when dealing with datasets in wide format. It helps make data more accessible for analysis and visualization tasks. We hope this article has elaborated on how to use the pandas.melt() function from the Pandas library. Here’s another article that details how to get column names in Pandas DataFrame.
Reference
https://pandas.pydata.org/docs/reference/api/pandas.melt.html