DataFrames are a well-known data structure provided by Python‘s Pandas library. They can be used to store data in a two-dimensional tabular form, which is convenient for visualizing and manipulating various types of data. Since DataFrames are 2D, they contain rows and columns. In this article, we’re going to look at 3 distinct methods of counting rows and columns in a DataFrame along with some examples.
Also Read: 4 Ways to Add a Column to DataFrame in Pandas (With Examples)
Methods to Count Number of Rows and Columns in Pandas
The Pandas library provides us with various functions that can help in counting the number of rows and columns in a DataFrame. Here, we’ll look at some of the following methods in detail:
- Using len() function
- Using len(df.axes[]) function
- Using shape attribute
Let us understand these in detail backed up by examples for better understanding.
1. Using len() Function
The DataFrame.len() method is typically used to return the number of rows in a DataFrame. However, by using the columns attribute which returns the labels of columns in the DataFrame, which can be counted using len() again, to return the number of columns. Let us understand this better with an example.
Example:
We will start by importing the pandas library into our file and creating our DataFrame.
import pandas as pd
dataframe = {'Fruit' : ['🍎','🍋','🍊'],
'Name' : ['Apple', 'Lemon', 'Orange']}
df = pd.DataFrame(dataframe)
print(df, "\n")
print("number of rows:",len(df))
print("number of columns:",len(df.columns))
Here, we have a dictionary dataframe, containing two keys, Fruit and Name and a list of three corresponding values in each column. This dictionary can be converted to a DataFrame using the DataFrame() method, where the keys become the columns of DataFrame and list values become the rows. The returned DataFrame is stored in df and we can print df to better visualize the DataFrame.
We then pass in our DataFrame df to the len() function, which returns the number of rows. To get the column count we pass in df.columns into len().
Output:
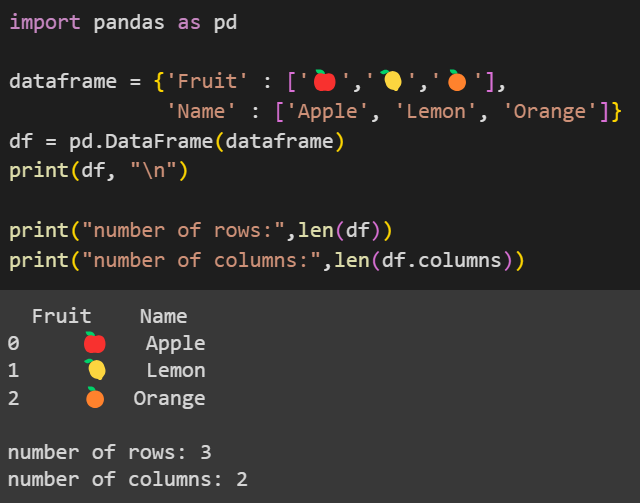
2. Using len(df.axes[]) Function
A simple variation to using len() separately on df and df.columns would be to use the df.axes attribute to specify which axis we’re taking the count of. While using axes, axes[0] represent the rows of the DataFrame and axes[1] represent columns of the DataFrame.
Example:
import pandas as pd
dataframe = {'Fruit' : ['🍎','🍋','🍊'],
'Name' : ['Apple', 'Lemon', 'Orange']}
df = pd.DataFrame(dataframe)
print(df, "\n")
print("number of rows (using axes) :",len(df.axes[0]))
print("number of columns (using axes):",len(df.axes[1]))
Here, we have used the same example as before but replaced df and df.columns passed into the len() function with their corresponding axes attributes. We start off by importing pandas and creating a dictionary dataframe. This dictionary is later converted to a DataFrame using the DataFrame() function. Then we pass in df.axes[0] into len() to compute the row count and df.axes[1] to compute the column count. The row and column count remains the same as in the previous example.
Output:
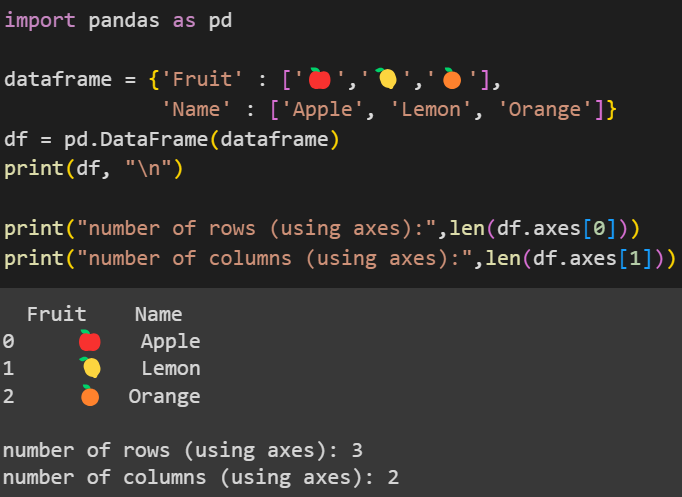
3. Using shape Attribute
The shape attribute in Datarame is used to return a tuple containing the row count and the column count in the form – (row count, column count). It can also be used to count the number of rows and columns separately by using the axes. Let us modify the last example to use shape.
Example:
import pandas as pd
dataframe = {'Fruit' : ['🍎','🍋','🍊'],
'Name' : ['Apple', 'Lemon', 'Orange']}
df = pd.DataFrame(dataframe)
print(df, "\n")
print("shape of DataFrame: ", df.shape)
print("number of rows:",df.shape[0])
print("number of columns:",df.shape[1])
Here, we have first imported the pandas library and initialised a dictionary, dataframe. The dictionary is then converted to a DataFrame using the DataFrame method which converts the keys of the dictionary to rows of the DataFrame and the list of values into rows. The DataFrame is stored in df.
We can now use df to access the shape attribute. We use this attribute to print the total shape using df.shape, which is returned in the form of a tuple containing both rows and columns. Then, we use it along the axes to separately print out rows using df.axes[0] and columns using df.axes[1].
Output:
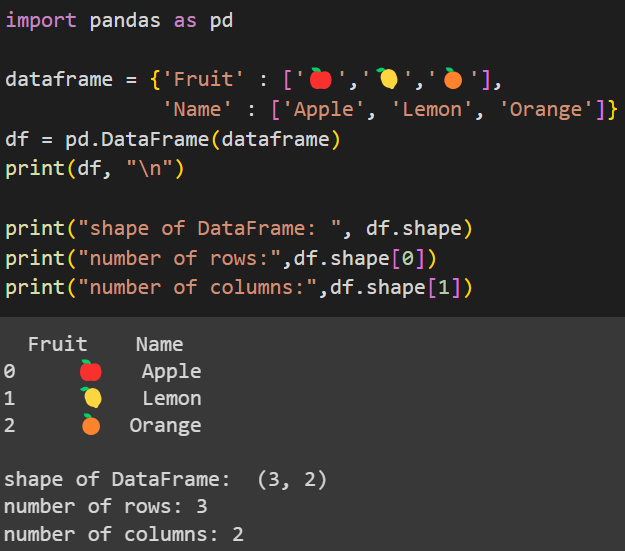
Conclusion
DataFrames form an integral part of Pandas that helps structure data cleanly and in a way that can easily be understood. Hence, it is essential to know how to perform basic functions on DataFrames such as returning the row count and column count of a given DataFrame. In this article, we have looked at 3 simple ways in which we can count the number of rows and columns using the len() function, using the axes attribute and passing it into len() and finally using the shape attribute.