In this article, we will explain what object prototypes are in JavaScript, their use, and various methods and concepts around it.
Before moving on with the topic, it is necessary to have a basic understanding of JavaScript objects. JavaScript object is a non-primitive data type in JavaScript that stores multiple collections of data, or properties.
Read more about JavaScript objects here.
JavaScript Object Prototypes
Try opening your Browser’s console window, type the following and press enter.
let website = {"javaScript": "CodeForGeek"}
Now console.log the object “website” we just created, using the below code.
console.log(website)

Now if we expand the object we find another object called prototype denoted by [[Prototype]] and on further expansion, we find various methods and properties attached to this prototype object.
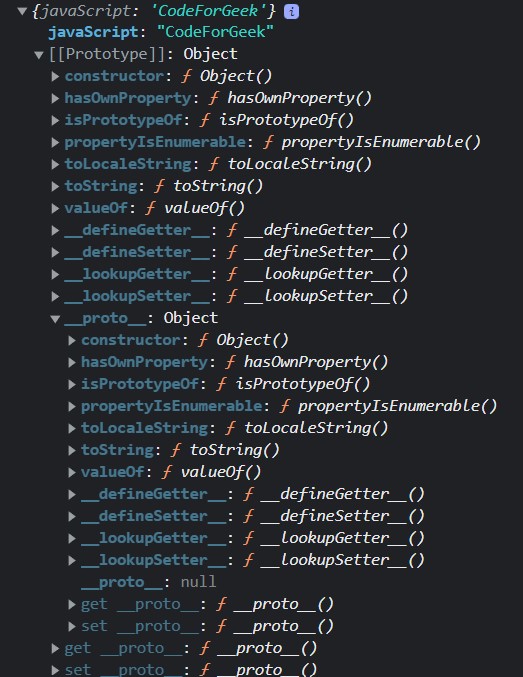
JavaScript objects inherit methods and properties from one another using prototypes. Every object has its own property called a prototype. As the prototype is also an object, it has its own prototype object. This is called prototype chaining and it ends when a prototype has null for its prototype.
JavaScript Prototype Chaining
If we access the “javascript” property of the object “website” we are returned with ‘CodeForGeek’. Now if we try to use any of the methods and properties defined inside the prototype object, we get the following result.
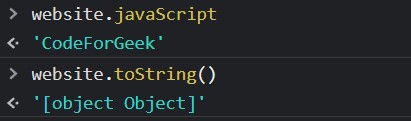
Here we used the toString() method. The JavaScript engine tries to provide us with a correct output even though the object “website” does not know about the toString() method.
So now we know if we call any methods or properties on any object, the JavaScript engine tries to find it inside the object first. If it is not able to find it inside the object it looks for it inside the prototype and then inside the prototype’s prototype until it reaches the end of the prototype chain.
JavaScript Prototype Linkage
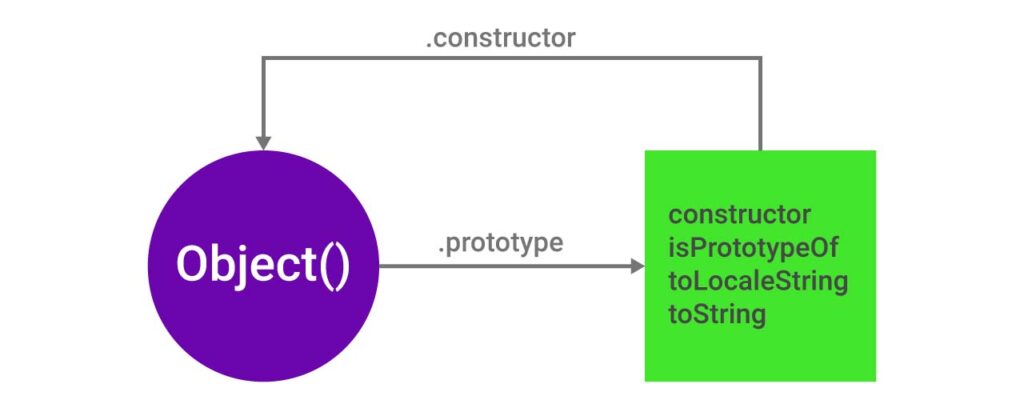
JavaScript provides us with a built-in Object() function which has some useful properties.

Learn more about how to get the type of object in JavaScript here.
console.log(Object.prototype)
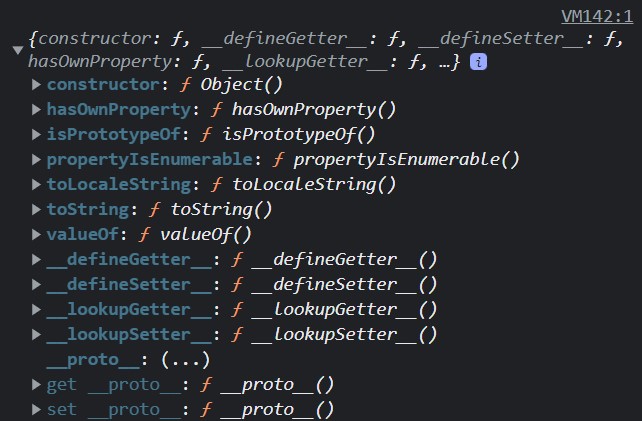
We can see that the Object.prototype.constructor property references the Object function.
console.log(Object.prototype.constructor === Object)
Output:
true
Now let’s create a constructor function called Website() that accepts an URL as a parameter. The function sets the URL property of this object to the input URL parameter.
function Website(url) {
this.url = url
}
JavaScript creates a new function Website() which has a property called prototype. The prototype object references an anonymous object which has a constructor property that references the Website() function.
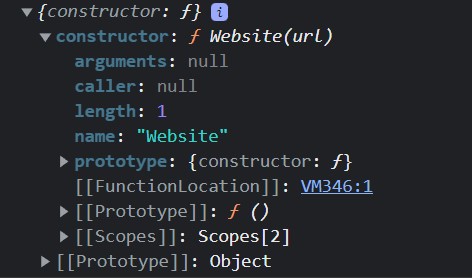
JavaScript links the Website.prototype object to the Object.prototype object with [[Prototype]]. This is called prototype linkage.
Defining Methods in Object Prototype
We can also define new methods and properties inside an object’s prototype. Try defining the following function
function Website(url) {
this.url = url
}
Now we will add a new method to the prototype of the above function and initialize an instance of the function in a constant called site and then invoke the new method.
Website.prototype.newUrl = function () {
console.log("New URL is", this.url)
}
const site = new Website("AskPython")
site.newUrl()
Output:
New URL is AskPython
JavaScript creates a new object site and links it with Website.prototype. Due to prototype linkage and chaining site object is able to call the newUrl method even when newUrl was not defined in the site object.
JavaScript Objects Shadowing Property
What would happen if we were to define the newUrl method inside the site object and then invoke the newUrl method in the site object?
site.newUrl = function () {
console.log("Shadowing took place")
}
site.newUrl()
Output:
Shadowing took place
The result is evident as we know about prototype chaining. The JavaScript engine first looks for the method in the site object and then looks for it in the object prototype, but in this case, the newUrl() method was defined in the site object so it shadows the method defined in Website().
This is called “shadowing” property.
Summary
In this article, we discussed JavaScript object prototypes.
- Every object has a prototype object
- Prototype chaining allows objects to inherit features from one another.
- JavaScript has a built-in Object() function
- The constructor property of Object.prototype object references the Object function.
- Shadowing property
References
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Basics
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Object_prototypes