Util Module provides many utility methods used for scaling the NodeJS application and in the development of modules.
It is a built-in NodeJS module, so it is not required to install it using NPM or Yarn.
Syntax:
The syntax for accessing the Util Module in a NodeJS is given below.
const util = require('util')
Methods of Util Module in NodeJS
Below are the methods of the Util Module that we can implement in our application to make it to the next level.
Example of Util.deprecate() Method
This method is used to send a deprecation warning. This is useful when the developers deprecated a method from a package.
Syntax:
util.deprecate(fn, msg)
where
- fn is a JavaScript function,
- msg is the warning in a string
Example:
Suppose, You have created a package “helloModule” which has a method “hello” to return the “Hello World!” string.
const util = require('util');
function hello() {
return "Hello World!";
}
module.exports = hello;
Someone uses this package to print the “Hello World!” string on the console.
const helloModule = require('./helloModule');
console.log(helloModule());
Output:
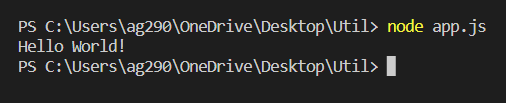
At some point in time, you decide to deprecate this hello method in the future, so you can use util.deprecate() method to export the “hello” method with a deprecated warning which shows to the client who implements this method.
const util = require('util');
function hello() {
return "Hello World!";
}
module.exports = util.deprecate(
hello,
'This function will be deprecated. Use something else instead.'
);
Output:

You have seen this type of deprecation warning when implementing some old method of NodeJS built-in or other NPM modules. They also used util.deprecate() method for creating this warning for the method which will deprecate.
Example of Util.formate() Method
This method is similar to the printf method in C, PHP, and other programming languages that provide string formatting utilities.
This method takes a string as an argument that can contain specifiers like %s for string, %d for number, %o or %O for the object, etc, with their values separated by command and return a formatted string.
Syntax:
Util.formate(format[, ...args])
where
- format is a format string,
- args is the specifiers’ value
Example:
const util = require('util');
const output = util.format('Hello %s!', 'World');
console.log(output);
Output:

Example of Util.callbackify() Method
This method takes an async function or a function that returns a promise and returns a function having an error-first style callback as an argument. This callback has two arguments, the first is an error that occurs when the promise is rejected and the second is the resolved value.
Syntax:
util.callbackify(functionName)
where
- functionName is the name of a JavaScript async function
Example:
const util = require('util');
async function fun() {
return 'This is a result';
}
const callback = util.callbackify(fun);
callback((err, result) => {
if (err) throw err;
console.log(result);
});
Output:
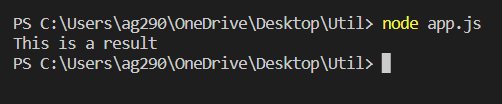
Example of Util.inspect() Method
This method works like the console.log() method but gives more control over the output. It can take multiple options as an optional argument containing various configurations regarding the output.
Syntax:
util.inspect(object[, options])
where
- object is a JavaScript primitive or object,
- options are the optional argument such as colors, depth, etc
Example:
const util = require('util');
const inspectOpts = {
colors: true, // Display output in color
};
console.log(util.inspect("Hello World!", inspectOpts));
Output:
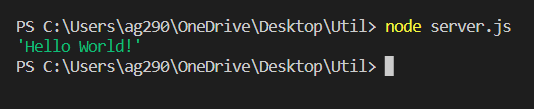
Example of Util.debuglog() Method
This method is used to create a function that writes debug messages based on the NODE_DEBUG environment variables to stderr.
Syntax:
util.debuglog(section[, callback])
where
- section is the part of the code for which the debuglog function is being created,
- callback is an optional argument
Example:
const util = require('util');
const debuglog = util.debuglog('HELLO');
debuglog('Hello [%S]!', 'World');
By default, it doesn’t log anything, for logging the debug message it is required to add NODE_DEBUG=sectionName before the node fileName command.
NODE_DEBUG=HELLO node app.js
Output:
HELLO 3245: Hello World!
Summary
Util is a built-in NodeJS module that is best suited for module development, it provides many useful methods like util.deprecate() to show a deprecation warning, utl.formate() for formatting string, etc. Hope this tutorial helps you to understand the Util Module and its functionalities in NodeJS.
Reference
https://nodejs.org/api/util.html