NodeJS TLS/SSL is used to make the connection secure through the use of cryptographic protocols, which ensure the security of the transformation of information over the web. It uses public-key cryptography to encrypt messages.
The information transferred from a client to a server can be tempered by a hacker, and the information may contain confidential data, that should be highly secure, to increase security it is required to use TLS/SSL protocol which provides an extra layer of security on the transformation of information over the web.
Node.js TLS module
This module provides TLS/SSL using OpenSSL. For using this module, it is mandatory to import it.
Syntax:
var tls = require('tls');
Methods of TLS module
Let’s get started with the methods that you can use within the NodeJS TLS module.
createServer()
This method is used to create a server object which is used to open a server at a specified port and provide a way so that client can connect to the server at a defined port.
Syntax:
createServer(options, callback);
This method takes different options and a callback function as arguments.
connect()
This method uses to connect to a server whose port is passed as an argument to this method.
Syntax:
connect(port, options, callback)
This method takes a port, different options, and a callback function as arguments.
rootCertificates()
This method is used to get the string containing the root certificate.
Syntax:
rootCertificates()
This method does not take any argument.
getCiphers()
This method is used to return the array of supported TLS ciphers.
Syntax:
getCiphers()
This method does not take any argument.
Complete Example of Using NodeJS TLS module
Let’s look at an example to demonstrate the use of every method we have learned.
We start the code by importing the TLS module and FS module. Here we are using the File System Module to read the private key and public certificate from the file created in the next step.
var tls = require('tls');
var fs = require('fs');
We have created two files, private-key.pem and public-cert.pem containing the key and certificate used to secure the connection between the client and server.
Then we created variable options containing the key and certificate and use the file system module to read the key and certificate from the file private-key.pem and public-cert.pem respectively.
var options = {
key: fs.readFileSync('private-key.pem'),
cert: fs.readFileSync('public-cert.pem'),
rejectUnauthorized: false
};
Then we used the createServer method of the TLS module to create a server object by passing the options as arguments and a callback function that use a socket and use an event that emits when data is received then finally we close the server.
var server = tls.createServer(options, function(socket) {
socket.on('data', function(data) {
console.log('\nReceived:'+ data.toString())
});
server.close(() => {
console.log("Server closed successfully");
});
});
Then we set up a listener to listen at port 5000 using the server object.
server.listen(5000, function() {
console.log("Listening at port" + 5000);
});
In this step, we use the connect method to connect the server on port 5000 and create a variable value and set it to rootCertificate method which returns the string containing the root certificate.
Then we use the write method to write the value of the root certificate and this value will be sent to the server and printed by the event in the console. Then finally we use the end method to close the client.
var client = tls.connect(5000, options, function() {
value = tls.rootCertificates;
client.write(" rootCertificates : " + value[0]);
client.end(()=>{
console.log("Client closed successfully");
});
});
Complete Code
var tls = require('tls');
var fs = require('fs');
var options = {
key: fs.readFileSync('private-key.pem'),
cert: fs.readFileSync('public-cert.pem'),
rejectUnauthorized: false
};
var server = tls.createServer(options, function(socket) {
socket.on('data', function(data) {
console.log('\nReceived:'+ data.toString())
});
server.close(() => {
console.log("Server closed successfully");
});
});
server.listen(5000, function() {
console.log("Listening at port" + 5000);
});
var client = tls.connect(5000, options, function() {
value = tls.rootCertificates;
client.write(" rootCertificates : " + value[0]);
client.end(()=>{
console.log("Client closed successfully");
});
});
Output:
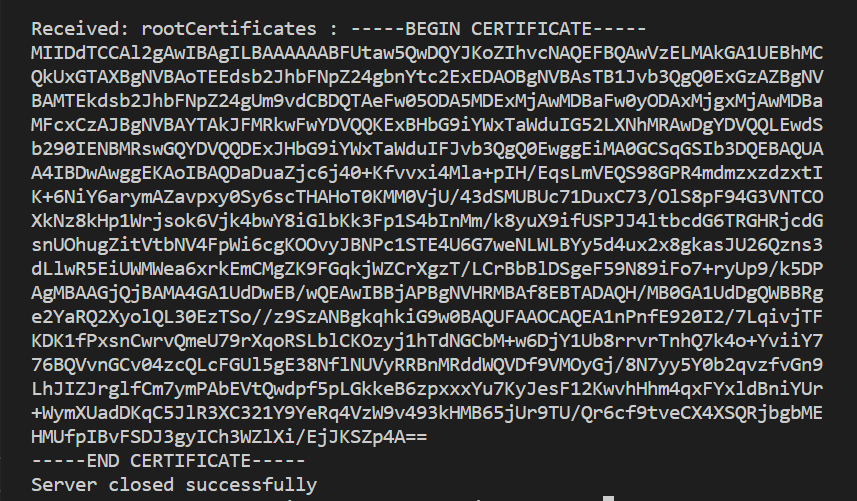
Summary
The TLS/SSL in Node.js is used to provide a secure connection between the client and server. This ensures that the data transferred between the client and server can’t be read by an intruder. Hope this article helps you to understand the concept of TLS/SSL in Node.js.