NodeJS is an open-source and cross-platform server environment that allows us to run JavaScript on a server. NodeJS uses asynchronous programming. NodeJS provides built-in modules which can be used without any Installation.
For Example, NodeJS provides http, https, fs, net, path and many other NodeJS modules. These Modules provide us with many functions to create the server environment. It also supports various collections of libraries and tools which are called Frameworks. The Frameworks that NodeJS supports are Express.Js, AdonisJS, MeteorJS, NestJS, SailsJS, Hapi.js, Loopback.io, Feathers.js, MEAN.JS, KoaJS.
What is Express.js Framework
Express is an asynchronous Model-view-controller Framework for NodeJS. It allows us to dynamically render HTML pages, and its robust API makes routing easy. It helps to design various web applications based on passing arguments to templates. It adds helpful utilities to Node.js HTTP objects and facilitates the rendering of dynamic HTTP objects. It allows you to specify a middleware for handling errors.
Simply, Express adds a layer of abstraction, a powerful routing system, middleware support, templating engine integration, error handling mechanisms, an extensive middleware ecosystem, HTTP utility methods, and flexibility to Node.js, making it easier and more efficient to build web applications with Node.js.
How to Install Express.js Framework
For using Express in your Node.js application, it is required to install it.
Syntax:
npm install express
After installing, you can build a simple webpage using Express by Importing the module and creating an instance of it that can be used to call its methods, define a route to produce the output using the get method and define a callback function to send a response on the webpage, and the listen method of the express module to produce the output at a particular port mentioned, You can check the output at http://localhost:8080.
Example:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(8080);
Output:
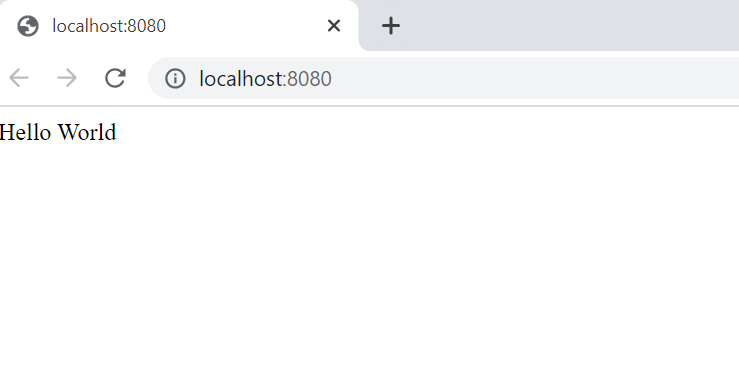
What is Node.js Connect
Connect is a Middleware framework that extends the built-in HTTP server functionality and adds a plug-in framework. Connect offers “higher level” APIs for common HTTP server functionality like session management, authentication, logging and more. Express is built on top of Connect with advanced functionality. Express itself comes with the most common Connect middleware.
Simply, Connect provides Middleware support, Built-in Middleware, Router and mount capabilities, and Advanced request and response handling, It enhances the flexibility, extensibility, and reusability of your Express applications, enabling you to build robust and feature-rich web applications.
How to Install Node.js Connect
To use the features provided by Connect we should install and import the module and create an instance of it, then use its method to handle the request and response objects.
Syntax:
npm install connect
After installing, you can build a simple webpage using the use method of Connect which is software that sits between your application program and some low-level API or that can be in between your request and response. The listen method produces the output at a particular port.
Example:
const connect = require('connect');
const app = connect();
app.use('/',(req, res) => {
res.end("Hello World with Connect");
});
app.listen(3000);
Output:
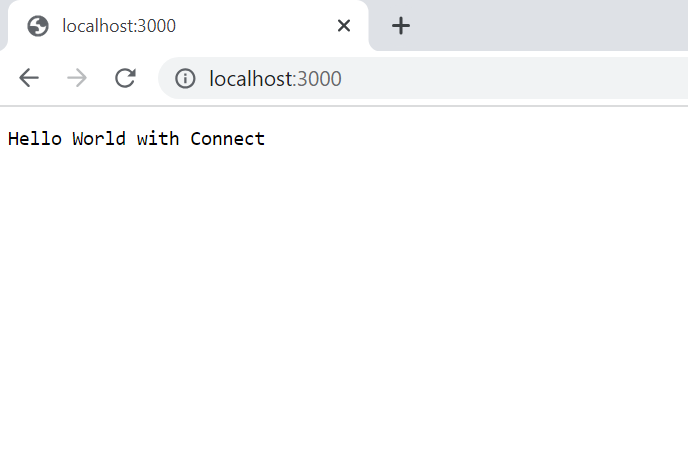
Express no longer uses Connect
Express.js no longer depends on or uses Connect middleware. While Connect is still a functional middleware framework, the trend has shifted towards using Express directly for most of the applications. However, it’s worth noting that Connect can still be used with Express in certain cases where specific Connect middleware components are required or for compatibility with older codebases.
The reasons for this might be the Express Middleware Ecosystem, Express Middleware API, Simplicity and Performance of Express, and Express as a Standalone Framework.
Popular Middleware Libraries for Express.js
The middleware functionality that Connect provides in Express is now mostly handled by the built-in middleware system of Express, as well as by popular middleware libraries specifically designed for Express.
Some of the commonly used middleware libraries that have gained popularity and are replacing Connect in Express are body-parser, cookie-parser, morgan, helmet, compression, and express-session used for session management. It provides a Middleware that provides session creation, storage, management and maintaining user sessions across requests.
Summary
When you’re using Express and need common functionalities, it’s usually more convenient to use Express’s built-in middleware and libraries. However, if you require more customization or have unique requirements, Connect middleware allows for greater flexibility and modularity. Consider the compatibility, specific functionality needed, customization requirements, and community support to make the best choice for your application.
Reference
https://stackoverflow.com/questions/5284340/what-is-node-js-connect-express-and-middleware