In our previous tutorial, we learned how to create different pages in Next.js using file-based routing but to switch between pages we changed the URL manually. Like for the Home page we entered http://localhost:3000/, for the About Us page we entered http://localhost:3000/about but how to navigate between pages directly? Well here comes the Link component in Next.js to link one page to another. Let’s see in detail what it is.
Link Component in Next.js
The Link component is used to navigate between different pages in a Next.js application. This is given by the next/link module and can switch between pages without triggering a full page reload. This makes the overall user experience better by providing seamless and fast page navigation. To link to a page all we have to do is import the <Link> component and define href.
Syntax:
import Link from 'next/link';
<Link href="/path">Page Name</Link>
Props:
- href: In the href, we pass the URL of a page to link it to.
- replace: If we set it to true, then if a user clicks on the back button after navigating, the browser will not take us back to the previous page.
- scroll: By default, Next.js will scroll to the top of the page when navigating to a new route and if false it will not scroll to the top.
- prefetch: By default, Next.js prefetches linked pages to increase navigation speed. If we set it to false, this will not happen.
Linking Between Pages in Next.js
Now we know what <Link> component is, let’s create a new Next.js application and use it to link to different pages.
1. Creating a New Next.js Project
Open a terminal and execute the below command to create a Next.js application:
npx create-next-app@latest
Make sure that Node.js version 18.17 or higher is installed on your system.
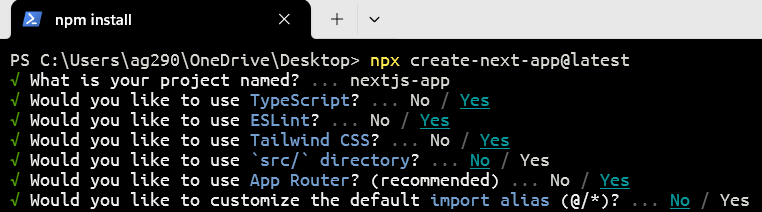
Check Yes for both TypeScript and App Router to follow along with us.
If you want a detailed guide on how to start with Next.js, click here.
2. Creating Different Pages
Once the project is set up, open it inside a code editor and remove all the files inside the app directory and create the following files:
app/page.tsx:
Inside the app directory, create a new page.tsx. This will be used as the home page for our Next.js application.
const HomePage = () => {
return <h1>Home Page</h1>;
};
export default HomePage;
app/about/page.tsx:
To create a new page for our application, create a folder named about with a page.tsx file inside the app directory.
const AboutPage = () => {
return <h1>About Us</h1>;
};
export default AboutPage;
app/contact/page.tsx:
Again create a folder named contact inside the app directory with a page.tsx to create a contact page.
const ContactPage = () => {
return <h1>Contact Us</h1>;
};
export default ContactPage;
This will eventually create three pages: home (“/”), about (“/about”) and contact (“/contact”). This process is called file-based routing which we have covered in our previous tutorial.
3. Linking Between Pages
Now create a file layout.tsx inside the app directory.
app/layout.tsx:
The layout (layout.tsx) is like a wrapper that goes with each page (page.tsx) within their respective folder or subfolder to construct a similar component for all of them.
For example, if we want the same navbar on all of our application pages, we can code it in app/layout.tsx and each page (page.tsx) of that folder or subfolder will have the same navbar.
import Link from 'next/link';
export const metadata = {
title: 'Next.js',
description: 'Generated by Next.js',
}
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en">
<body>
<ul>
<li><Link href="/">Home</Link></li>
<li><Link href="/about">About Us</Link></li>
<li><Link href="/contact">Contact Us</Link></li>
</ul>
{children}
</body>
</html>
)
}
The first line is the import statement, which imports the Link component from “next/link”. Then there is a metadata object that defines the meta title and description. After this, the code exports a component called RootLayout as the default export, using children as props of type React.ReactNode. This component returns JSX, which represents the structure of the HTML document. All the code inside this is just basic HTML containing components and children.
Output
Now execute the below command to run our Next.js application:
npm run dev
Here we are running the development server which listens on http://localhost:3000.
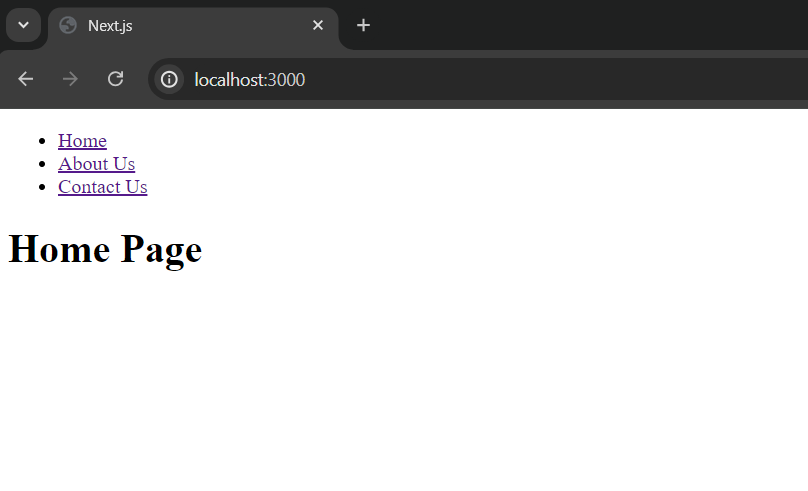
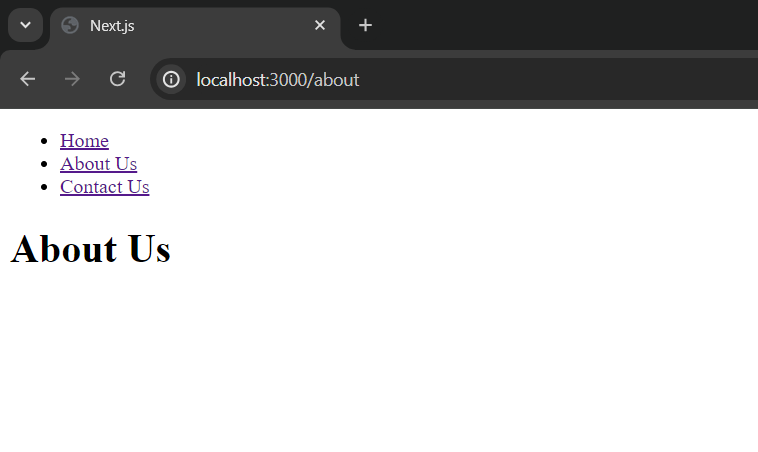
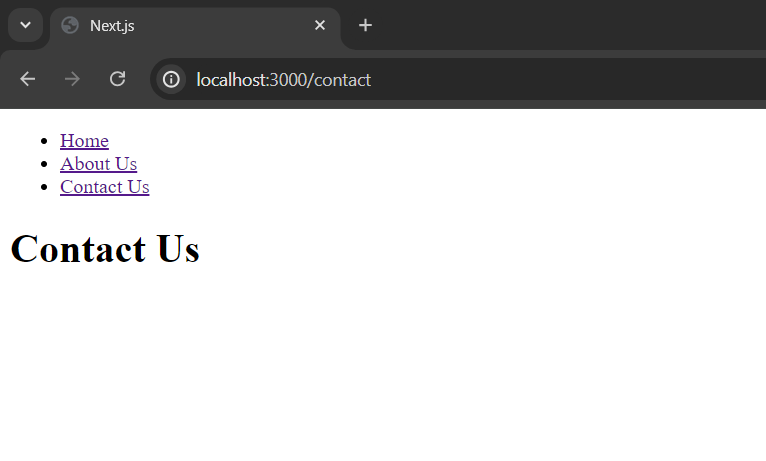
See the links are appear on each page and by clicking on any of them we can go to that page without a full page reload.
Summary
In short, the <Link> component is used to link to different pages in a Next.js application without triggering a full page reload which a typical <a> tag does. To use it, we have to import it from the next/link module and then define a href attribute inside it and pass the URL of a page we want to link it to and that’s it, using the link created we can navigate to that page. If you are creating a navbar, just put it inside app/layout.tsx and it will appear on each page of the application.
Reference
https://nextjs.org/docs/pages/api-reference/components/link