JavaScript and Node.js are completely different technologies but still related enough to confuse beginners. In this article, we will try to clear your JavaScript and Node.js concepts by creating a very simple random quote generator but we will write the code in two different parts: client side and server side to make it clear when JavaScript is used and when Node.js can be used.
Let’s start with the basics of JavaScript and Node.js and if you are already familiar with them feel free to skip the next section.
JavaScript and Node.js: From Browser to Server
JavaScript is a language developed by Brendan Eich in 1995 to make webpages more interactive. It was created to execute on browsers and hence is widely accessible and with the popularity of HTML and CSS it become an essential part of web development. It started out as a way to import some interacting features into web pages, but with new features and lots of regular updates, it became a powerful language on its own.
JavaScript is mainly used for client-side works like manipulating HTML and CSS, handling events, and interacting with the Document Object Model (DOM), for form validation, dynamic content loading, animations, etc.
Now the question is how can such a powerful language stay limited to just the browser. Well, not after 2009 when Ryan Dahl created Node.js. Node.js is a JavaScript runtime environment that allows us to execute JavaScript outside of web browsers.
Node.js is used to write server-side code for creating web servers, CLI tools, APIs, microservices, etc. It can create dynamic pages, interact with the file system and OS, support all types of databases and much more. Basically, it allows us to create scalable high-performance network applications.
So in sort, JavaScript is a programming language that is used to write client-side code, on the other hand, Node.js is an environment that lets us write JavaScript code for the server-side.
Creating Random Quote Generator Using JavaScript and Node.js
Let’s get started by creating a project folder and opening it inside a code editor.
We will be dividing our application into two parts: first is server-side, for which create a file ‘server.js’ (for Node.js), and the second is client side for which create a file ‘index.html’ (for JavaScript).
Code for Server Side
The server-side code runs on the server or in our case it will run on our system Node.js environment, basically we are creating a local server right now. The code executes on the server and performs the written task, then we will write JavaScript client-side code to make HTTP requests to our local Node.js server to get the desired response.
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
const quotes = [
"Talk to yourself once in a day, otherwise you may miss meeting an intelligent person in this world.",
"You cannot believe in God until you believe in yourself.",
"You have to grow from the inside out. None can teach you, none can make you spiritual. There is no other teacher but your own soul.",
"We are what our thoughts have made us; so take care about what you think. Words are secondary. Thoughts live; they travel far.",
"Truth can be stated in a thousand different ways, yet each one can be true.",
"Arise! Awake! And stop not until the goal is reached."
];
app.get('/random-quote', (req, res) => {
const randomQuote = quotes[Math.floor(Math.random() * quotes.length)];
res.json({ quote: randomQuote });
});
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
The code is very simple, it just imports the ‘express’ module, creates an instance of it to get its methods, and then uses its get() method to send a random quote as a response if the client makes a response to the ‘/random-quote’ route.
One thing to note here is about the ‘cors’ module: it is used when the frontend needs to make a request to the backend server to fetch a quote.
Make sure to install ‘express’ and ‘cors’ modules using ‘npm i express cors’, otherwise, you will end up getting cannot find module error.
Code for Client Side
Now let’s write JavaScript client-side code to make a request to the server we just created and get a random quote as a response.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Random Quote Generator</title>
</head>
<body>
<h1>Random Quote Generator</h1>
<button id="get-quote-btn">Get Random Quote</button>
<p id="quote"></p>
<script>
const getQuoteBtn = document.getElementById('get-quote-btn');
const quoteDisplay = document.getElementById('quote');
getQuoteBtn.addEventListener('click', () => {
fetch('http://localhost:3000/random-quote')
.then(response => response.json())
.then(data => {
quoteDisplay.textContent = data.quote;
})
.catch(error => {
console.error('Error fetching quote:', error);
});
});
</script>
</body>
</html>
Here the JS code first selects the HTML button and then it selects the paragraph having the id ‘quote’, we are adding an event listener on that button which triggers a callback function to fetch the quote and display the response to the selected paragraph element.
See in the callback part, we are using the fetch() method to request to ‘http://localhost:3000/random-quote’ because the server we created is local and running at ‘http://localhost:3000’.
In case you want to read more about fetch() – Getting data from RESTful API in Node
Application Output
Now our work is done. The first thing we need to do is run our local server. Make sure Node.js is properly installed on your system otherwise the code will not work.
Head over to this article if you don’t know how – Resolving ‘node’ is not recognized as an internal or external command Error
Now execute the below command to run our local server:
node server.js
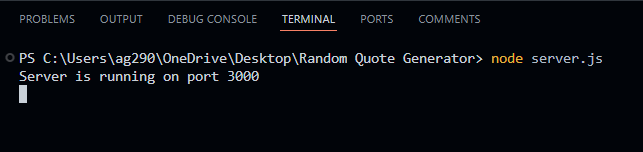
Now double click on ‘index.html’, basically we want to open it in a browser:
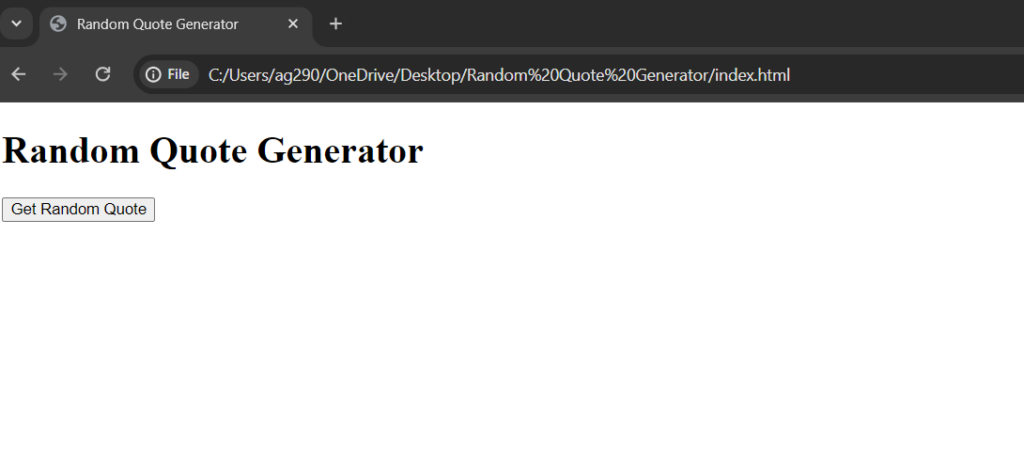
Moment of truth, let’s try to click the button:
GitHub repository of the project, Feel free to contribute!
Summary
So in short, we have learned that JavaScript is used for the front end, which runs on the browser, and Node.js is used for the backend which runs on the server, both can communicate together to make an app work.
That’s all for this article. If you like it, be sure to check out our main page, you will find a lot of interesting JS and Node.js articles that make all the difficult concepts easy.