The Nullish coalescing operator, also known as the Javascript double question mark operator (??), is a logical operator that takes two input statements and returns the right-hand side statement if the left-hand side statement is false. Typically, it establishes default values for variables. Let’s discuss it further.
Nullish Coalescing Operator in JavaScript
The Nullish coalescing operator or double question mark is somewhat similar to the logical OR (||) operator but with some differences which we will discuss later. It can function on both single values and statement conditions. The precedence of this operator falls between the || operator and the ternary operator (?:).
Syntax:
leftstatement ?? rightstatement
- leftstatement: It is the left-hand side input to the operator.
- rightstatement: The default value to return if leftstatement is null or undefined.
Both can be any value, expression, or statement.
Returns:
When the left side operand of the expression is traced as ‘null’ or ‘undefined’, then the right-hand side operand of the expression is returned:
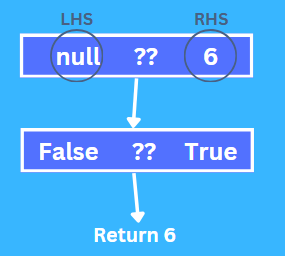
To put it simply, if the left-hand side part of the expression comes out to be a false argument, the operator returns the right-hand side part. Only the null and undefined are considered false arguments.
When the left-hand side operand is not a false argument, the operator returns the left-hand side operand.
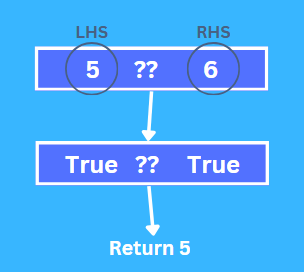
All the arguments other than null and undefined are true arguments.
Learn about JavaScript operators here.
Why Use the ?? Operator?
Now you might think that these types of comparison operations can also be performed by the logical (||) operator. Then what is the need for an operator like the Nullish coalescing?
JavaScript developers often need to use a default value when a given value is null or undefined. Before 2020, they usually used the logical OR (||) operator to do this. However, the OR operator has a problem: it treats any “falsy” value (like 0, an empty string, false, null, undefined, or NaN) as if it were null or undefined. This can cause mistakes because sometimes a false value like 0 or an empty string is actually valid and shouldn’t be replaced by a default value.
In 2020, JavaScript introduced the ?? operator, which only treats null or undefined as reasons to use a default value, avoiding these mistakes.
Nullish Coalescing vs Logical OR Example
Suppose we need to set a default value for the number of apples in a basket.
Then, if we use the || operator for setting the default value:
function getNumberOfApples(applesInBasket) {
return applesInBasket || 10;
}
console.log(getNumberOfApples(0));
console.log(getNumberOfApples(null));
console.log(getNumberOfApples(undefined));
console.log(getNumberOfApples(5));
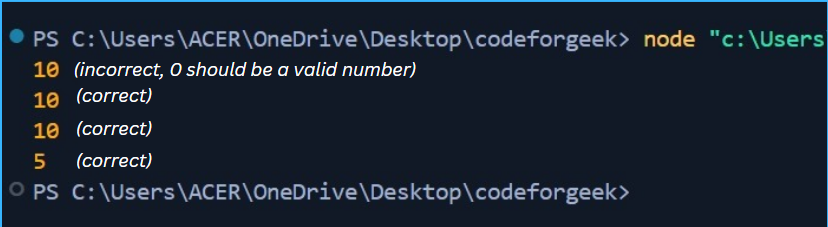
We know zero is a valid entity for the number of apples, but it is not considered a true argument by the OR operator.
So we use the ?? operator to overcome the problem:
function getNumberOfApples(applesInBasket) {
return applesInBasket ?? 10;
}
console.log(getNumberOfApples(0));
console.log(getNumberOfApples(null));
console.log(getNumberOfApples(undefined));
console.log(getNumberOfApples(5));
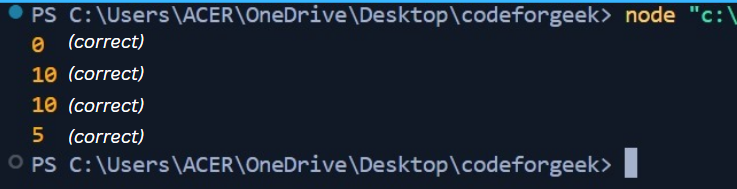
This will consider zero as a true argument and hence sort our issue.
Values that Considered False in Nullish Coalescing Operator
Now let’s see different cases of the Nullish coalescing operator and try to understand the values that are considered false in this operator.
1. Null
Null is a special value in JavaScript that indicates the absence of any value, i.e., no value. It is considered a false argument in Boolean expressions.
let currentValue = null;
let isCorrect = currentValue ?? "The current Value is not True";
console.log(isCorrect);

2. Undefined
When a variable is declared but not assigned any value, it is said to be undefined in Javascript. It is also a false argument for the ?? operator.
let currentValue ;
let isCorrect = currentValue ?? "The current Value is not True";
console.log(isCorrect);
In this code, currentValue is declared as a variable but has not been assigned any value. As a result, it enters the operator as undefined, causing the right-side value to be returned.

3. 0 Value
Zero is an integer value. Many logical operators consider zero as a false argument, but for the ?? operator, the value is a true argument.
function Temperature(celcius) {
console.log("The room Temperature is: ", celcius ?? 25 );
}
Temperature(0);
Temperature(-32);
Temperature(32);
Here, the operator considers every value entered, including zero, as a true argument and hence returns the same.
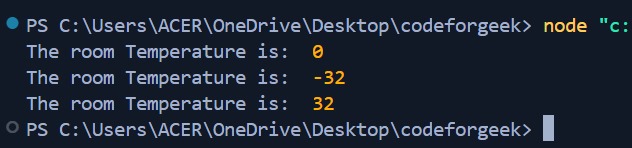
4. NaN Value
NaN stands for “Not-a-Number”. It occurs when an operation cannot produce a valid number. In JavaScript, the ?? operator treats NaN as a valid value. Therefore, when NaN is encountered, this operator will return NaN instead of the expression on the right side.
let value = 10 - "codeforgeek";
let isCorrect = value ?? 10;
console.log(isCorrect);
In this code, 10 – “codeforgeek” turns out to be a NaN value.

5. False
false is a boolean value that shows a negative condition or a lack of truth.
function Raincheck(isRaining) {
let Status = isRaining ?? true;
console.log(Status ? "Take an umbrella." : "No need for an umbrella.");
}
Raincheck(false);
Raincheck(true);
If false is passed as an argument, the ?? operator will return false. Consequently, the next line will print ‘No need for an umbrella,’ since the condition is false.

6. Empty String Value
An empty string means when there is no text or character inside the double quotes. It also stands as a valid argument for this operator. If an empty string is used as the left-side operand, then the empty string itself is returned.
let isCorrect = " " ?? "codeforgeek";
console.log(isCorrect);
If you run this code, you will see a blank screen because the string displayed is empty.

Short-Circuit Behavior of the Nullish Coalescing Operator
The Nullish coalescing operator is short-circuited, which means that if the left-hand value is not false, then the right-hand expression is not evaluated. It only checks on the left-hand side of the expression.
Suppose we have any undefined or null values on the right-hand side. The operator is not bothered by them until the left-hand side value turns false. This saves time and makes the code more efficient.
let currentValue = 90 ;
let isCorrect1 = currentValue ?? null;
let isCorrect2 = currentValue ?? undefined;
let isCorrect3 = null ?? undefined ;
let isCorrect4 = undefined ?? null ;
console.log(isCorrect1);
console.log(isCorrect2);
console.log(isCorrect3);
console.log(isCorrect4);
In the first two cases, the operator is not affected by the right-hand expression. It only checks if the left-hand condition is true and prints the corresponding result.
In the last two cases, since the left-hand condition tends to be false, it prints the right-hand expression regardless of whether the argument (right-side) is true or false.
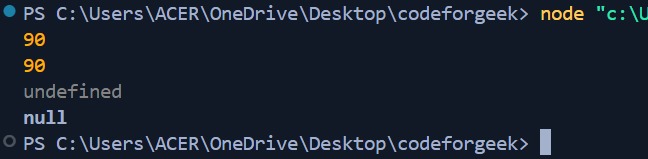
Chaining with Other Operators
We know that we can chain and combine different logical operators, like combining OR (||) with AND (&&), in a single expression like:
let result = true || false && false;
console.log(result);
The given expression will be operated according to the precedence: firstly, false && false results in false, and then true && false results in true.

But in the case of chaining with the ?? operator, we cannot directly chain it with other operators like this:
let result = true || false ?? false;
console.log(result);
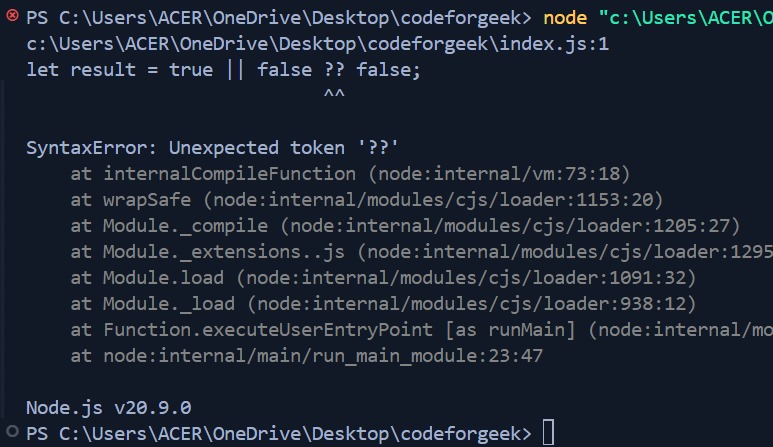
According to our expectations, the result must be true, but an error occurs. This is due to Javascript parsing rules.
We can overcome this problem by setting braces to explicitly specify the operator precedence, like:
let result = true || (false ?? false);
console.log(result);
Now the false ?? false expression will operate first and return false. Then true || false returns true.

Nullish Coalescing Operator Truth Table
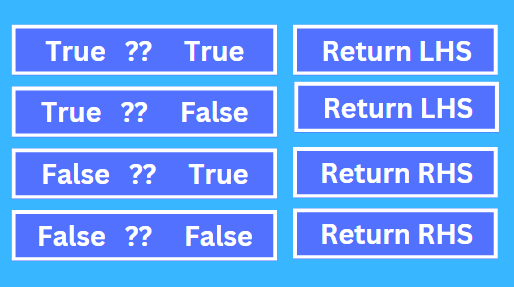
Conclusion
And that’s the end for this one. After reading this article, you are now clear with all the concepts of the ?? operator. This operator ensures defaults are applied only for null or undefined values, preserving other false values. It avoids unintended overwrites compared to the || operator.
Would you like to learn how to convert a JavaScript NodeList to an array? Click here to find out!
Reference
https://stackoverflow.com/questions/71238309/what-is-double-question-mark-equal