A template engine is used for server-side rendering to inject the data into the HTML template. A template engine can contain multiple variables, these variable is then replaced with the value sent from the server, these values taking the place of variables in the HTML template will then display to the client.
For example, suppose there is a simple web application that required to show the name of the user registered, so a template engine can be used to form an HTML template that contains a variable “userName” when the user is registered and enters their name, the value of the name is captures and send to the template engine, which replaces the variable “userName” with the name entered and this eventually creates the website completely dynamic which change the value of user name depending to the user.
There are many template engines available to use in Node.js such as EJS, Pug, Handlebars, Jade, Mustache, Nunjucks, etc.
In this tutorial, we will learn about Jade Template Engine.
Also Read: Step-by-Step Guide to Using EJS Template Engine in Nodejs
Jade Template Engine
Jade is a template engine used in Node.js to write beautiful, well-organised, and less code to create HTML templates mainly for rendering data.
There are many template engines used with Node.js, one of the popular is EJS, but since Jade requires significantly fewer lines of code in comparison to EJS, it is more recommended.
Installing Jade in Node.js
npm i pug
Note: Jade has been renamed to pug.
Jade vs HTML
A simple Hello World Page can be written in HTML as:
<!DOCTYPE html>
<html>
<head>
<title>Hello from Jade page</title>
</head>
<body>
<h1>Hello World!</h1>
</body>
</html>
The same code can be written using Jade as:
doctype html
html
head
title Hello from Jade page
body
h1 Hello World!
Fundamental of Jade Template Engine
1. Jade Template have no closing tags.
2. It does not use < or > symbols to wrap the HTML tag.
3. It uses indentation to detect which block contains which code, similar to python.
4. Jade treated the first word as a tag, the rest was the value inside that tag.
How to use Attributes in Jade Template Engine?
An attribute in Jade can be used by passing it inside the round bracket() after the tag name.
Example:
doctype html
html
head
title Hello from Jade page
body
h1(class="heading") Hello World!
This code is similar to the below HTML code.
<!DOCTYPE html>
<html>
<head>
<title>Hello from Jade page</title>
</head>
<body>
<h1 class="heading">Hello World!</h1>
</body>
</html>
How to use JavaScript in Jade Template Engine?
JavaScript code can be embedded in Jade using a hyphen symbol(-) at the stating of the JavaScript code.
Example:
doctype html
html
head
title Hello from Jade page
body
h1 Hello World!
ul
- for(var i=0; i<5; i++)
li= i
This code is similar to the below HTML code.
<!DOCTYPE html>
<html>
<head>
<title>Hello from Jade page</title>
</head>
<body>
<h1>Hello World!</h1>
<ul>
<li>0</li>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
</body>
</html>
Hello World Application using Jade Template Engine in Node.js
Let’s create a simple application to revise the concept we have learned yet.
app.js
const express = require('express');
const app = express();
app.set("view engine","pug")
app.get('/', function (req, res) {
res.render('hello', {variable: "Hello World!"});
});
app.listen(3000, function () {
console.log('Server is running on port 3000');
});
Here we have imported Express, then initialise “pug” to use as a template engine, then use the get() method to send the jade file when a user requests to the home(“/”) route, and set the variable “variable” to some given value.
hello.pug
doctype html
html
head
title Hello from Jade page
body
h1 #{variable}
Here #{variable} is considered as a variable that is replaced with the value sent from the server.
Output:
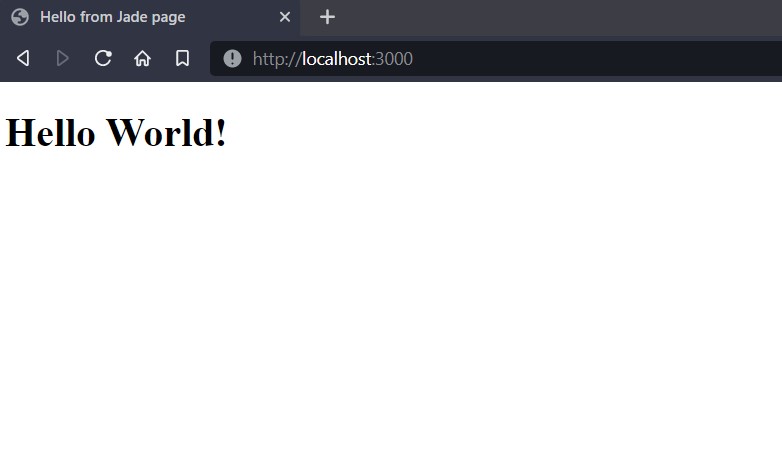
Summary
Jade provides a way to create an HTML template in which data from the server can be injected. It also lets us create the template in only fewer lines of code. Jada template has no closing tags, it does not use < or > just like any other template engine, it used indentation for block detection. Jade is recently named pug, so next time you give it a try, install pug rather than jade. Hope this tutorial helps you to understand the Jade Template Engine.
Reference
https://www.npmjs.com/package/jade
https://www.npmjs.com/package/pug