NumPy is a famous Python library used to perform various types of mathematical operations on elements of multi-dimensional arrays. It is a fundamental package when it comes to scientific computing with Python. In this article, we’ll look at three ways of inverting elements of a boolean array with NumPy along with some examples for better understanding.
Also Read: numpy.cbrt() in Python – Calculating Cube Roots in NumPy
Methods to Invert Elements of a Boolean Array
A boolean array is an array containing only values indicating True or False for every variable in it. Three methods of inverting a boolean array are as follows:
- Using if-else & for loop
- Using the logical_not() function
- Using the invert() function
Now let’s explore these methods in detail with examples.
1. Using if-else & for loop
The simplest and most straightforward way of approaching this is iterating over each element in the array, checking whether they indicate True or False and inverting each element to indicate the opposite.
Example:
arr = [0, 1, 0]
for x in range(len(arr)):
if(arr[x]):
arr[x] = 0
else:
arr[x] = 1
print(arr)
In this example, we have an array arr, containing only values 0 and 1 where 0 indicates False and 1 indicates True. Then we iterate through the elements of the array and check with if-else statements whether the element indicates True or False. If an element indicates True, we invert it to False and if the element indicates False, we invert it to True.
Output:
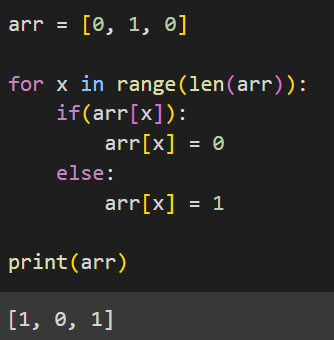
2. Using the logical_not() function
Another method of inverting a boolean array is using NumPy’s logical_not() function. This function computes the logical NOT of each element in the input array. The syntax of this function is given below.
Syntax:
numpy.logical_not(arr, out=None, where = True, casting = ‘same_kind’, order = ‘K’, dtype = None, ufunc ‘logical_not’)
Parameters:
- arr – Parameter used to represent the input array to perform logical NOT on. This is a required parameter.
- out – Represents the name of the output array we want to store the result in.
- where – Used to specify whether logical NOT operation should be performed on all elements of the input array.
- casting – Used to specify whether type casting should be done for elements of the output array. ‘same_kind’ signifies that the output array has the same data type as the input array.
- order – Specifies how memory is laid out for the output array. ‘K‘ indicates the most efficient memory layout.
- dtype – Used to specify the data type of the output array.
- ufunc – Used to specify universal function.
Example:
import numpy as np
arr = np.array([0, 1, 0])
result_arr = np.logical_not(arr)
print(result_arr)
We first initialize an array arr containing only values 0 and 1, using the np.array() function. Then we apply the logical_not() function on this array which performs logical NOT operation on every element in the array. The resultant array is stored in result_arr. It is important to note that logical_not() gives results only in terms of True or False. Even if our input array contains 0 and 1 values, the output returned contains True and False.
Output:
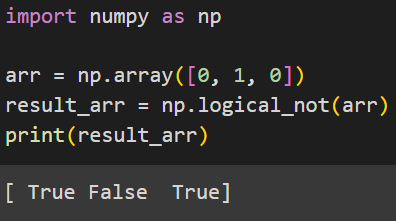
3. Using the invert() function
NumPy provides another method invert() which is similar to logical_not() which can be used for inverting boolean arrays. This function computes the bit-wise NOT of every element in the input array. However, this method only works for arrays containing True and False values and not 0 and 1. The syntax of invert() is given below.
Syntax:
numpy.invert(x, out=None, where = True, casting = ‘same_kind’, order = ‘K’, dtype = None, ufunc ‘invert’)
Parameters:
- x – Represents the input array containing boolean values True and False.
- out – Optional parameter indicating the name of the resultant or output array.
- where – Used to specify whether an inversion operation should be performed on all elements of the input array.
- casting – Used to specify whether type casting should be done for elements of the output array. ‘same_kind’ signifies that the output array has the same data type as the input array.
- order – Specifies how memory is laid out for the output array. ‘K‘ indicates the most efficient memory layout.
- dtype – Used to specify the data type of the output array.
- ufunc – Used to specify universal function. By default, the universal function is ‘invert’.
Example:
import numpy as np
arr = np.array([True, False, True])
result_arr = np.invert(arr)
print(result_arr)
First, we import the NumPy library as np. Then we initialize an input array arr containing True and False values using np.array(). We then apply the invert() function on the input array which performs bit-wise NOT operation on each element of the array. The resultant array is stored as result_arr.
Output:
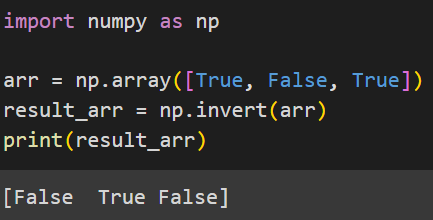
Conclusion
Inverting elements in a NumPy array is a common operation performed in many scientific computation and data manipulation tasks. In this article, we’ve looked at three different methods of inverting elements in a NumPy array from the simplest method using if-else statements, using NumPy’s invert() and logical_not() functions along with supporting examples for better understanding.
Reference
https://stackoverflow.com/questions/13728708/inverting-a-numpy-boolean-array-using