Whenever we create a Node.js application there is a need to use external packages for additional features that Node core libraries are not providing. These external packages can be installed using NPM.
NPM stands for Node Package Manager. It is an online repository of thousands of Node.js packages that are free to install, open source and can be used in the creation of different types of applications. For instance, express is a package on NPM that makes writing Node.js easy, mongoose is a package used to connect with the MongoDB database, and so on.
But NPM is not limited to downloading packages from it, we can build our own packages and publish them on NPM so that other developers on the internet can install them and use the features we created.
In this article, we will learn how to create an NPM package or module from scratch and then publish it to the public NPM registry. So let’s get started.
Prerequisites
There are some prerequisites before deep dive into creating the NPM package.
Install Node.js
You should have Node.js installed locally on your system. You can download it directly from here.
To make sure that Node.js is properly installed, open the command line and type the command
node --version
make sure that it returns a version.
Create an NPM account
For publishing the package we will build, we need to create an account in the NPM registry. Just head over to https://www.npmjs.com/ for creating your free NPM public registry account.
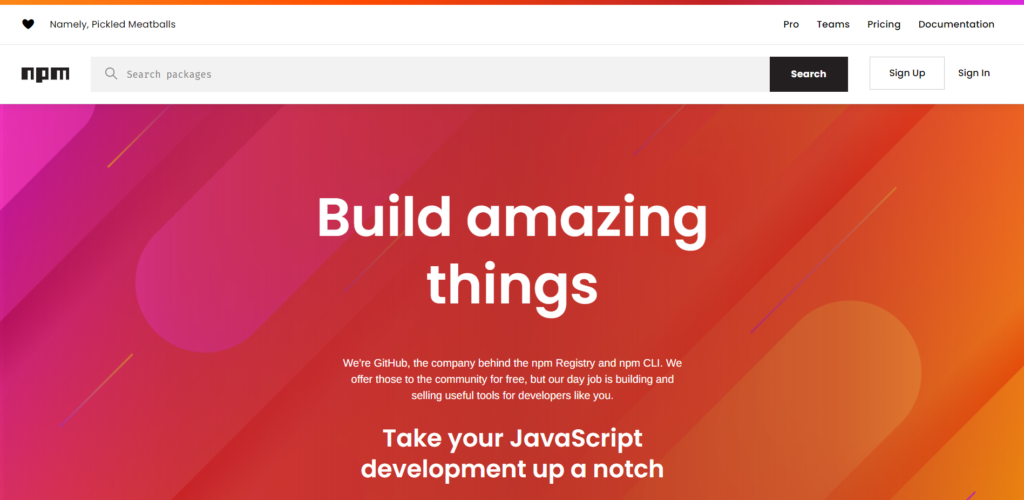
Creating an NPM Package
Once you have the prerequisites met, move on to creating a simple NPM package to demonstrate uploading it. Below are the steps to build an NPM package.
Project Setup
Create a new folder, locate it inside the terminal, and execute the below command to initiate the NPM.
npm init
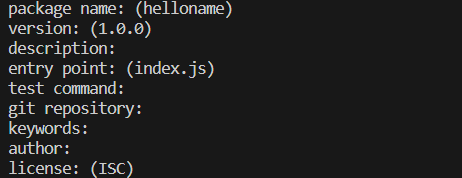
We usually use the “-y” flag with this command as we want to skip entering the details of the project, but since we have to publish it, it is required to enter all the relevant details, such as package name, version (following semantic versioning principles), description, etc. After all this, a package.json file will be created inside the project with the details entered by you.
Writing Code
Now, inside the project folder create a file “index.js” where we will be writing code for creating the functionality of our package. No worries, we will just write a very basic code for demonstration.
index.js
function helloName(name) {
return "Hello " + name;
}
module.exports = helloName;
Here we have used a JavaScript function that takes a name as an argument and return it with a “Hello” word. For instance, if someone uses this package and called the helloName function, and passed “Aditya” as an argument, the function will return “Hello Aditya”.
Testing Your NPM Package
To test a package before going live, you need to follow the below steps. You can skip them if you don’t want to test your package. This section is completely optional.
Step 1: In the terminal, navigate to the project folder and execute the below command to make your package globally available.
npm link
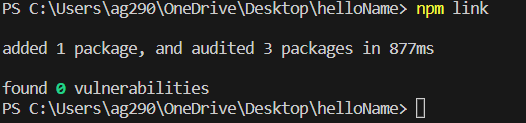
Step 2: Create another folder, let’s name it “test”, navigate it in the terminal and execute the below command.
npm link <name-of-package>
In our case, the name of the package is “helloname”, so we will execute the command npm link helloname.

Now, you can find the package helloname in the node_modules folder just created automatically.
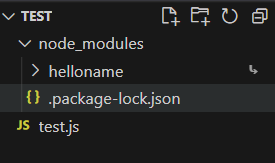
Step 3: Create a test file called “test.js” inside the “test” folder.
Step 4: Write code to use the package by requiring it and then call its function with an argument to test the output.
const helloName = require('helloname')
console.log(helloName("Aditya"))
Step 5: Run the program by executing the following command.
node test.js
Output:

Here we can see that we got the expected output, which means that our package is working properly. Let’s now publish it.
Publishing Your NPM Package
To publish a package to the NPM repository, you need to log in to the NPM registry, for that execute the below command in the root of your main project folder.
npm login

Then enter your NPM credentials.
Now, run the below command to finally publish your package.
npm publish
This way you can publish your first NPM package.
Note: Make sure that your package folder does not contain any confidential information, otherwise it will be uploaded with your source code and your secret will be known to the world.
Conclusion
To publish your NPM package, you just need to have Node.js installed, an NPM account, and a Node.js project. Then you can execute the npm init command and type relevant details about your package, then use the npm login command and enter your NPM username and password, then finally publish it using the npm publish command.
This article is just a demonstration of how you can publish your own npm packages to online NPM repositories. You can also create some advanced dependencies and show them to the world. Hope you enjoy reading the content.
Read More:
Reference
Contributing packages to the registry – npm docs