A Video Streaming Application is used to play videos. Examples of video streaming applications are Youtube, Netflix, etc, this application have a huge number of videos in their database and has a system to play those videos.
In this tutorial, you will learn to create a video streaming application and understand how to handle video play in NodeJS.
Building a Video Streaming Application in NodeJs
A video streaming application has many videos in its database, we are going to mimic it, but we consider having only one video which makes the development process easy to understand for beginners, then we will create some functionality to play that video.
Below is the step-by-step guide to creating a video streaming application in NodeJS.
Structuring the video streaming application project
Let’s start with creating a project folder having files “app.js” to write the NodeJS code, “index.html” for HTML code, and a video file. The video file can be any video file of any type but we will configure this application for playing MP4 videos which you can easily change during production, we also recommended using small video to increase the loading speed.
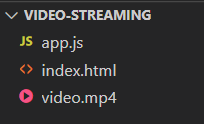
Project Setup
Initiate NPM: It is required to initiate NPM in order to install any NodeJS module. Locate the project folder in the terminal and execute the below command in the terminal to initiate NPM.
npm init -y
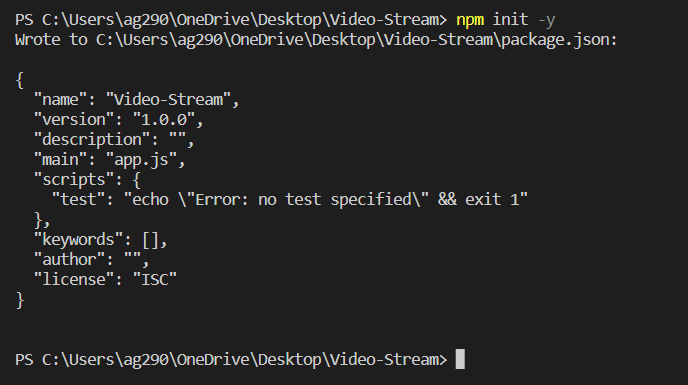
Install Express: To install Express as a module inside the project folder run the below command.
npm i express
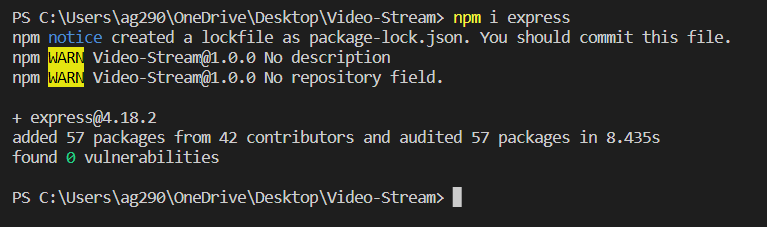
Writing Code to Build a NodeJS Video Streaming App
Import Express and File System Module: Inside the “app.js” file, import the express and file system module.
const express = require('express');
const fs = require('fs');
Create Instance: Create a constant “app” and set it to “express()”, which means creating an instance of an express module, by which any of the express methods can be called.
const app = express();
Setup Home Page: To set up the home page use the get() method to send the “index.html” file to the home(“/”) route.
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
})
Setup Video Player Route: We have to set up another route “/videoplay” which is used to stream the video file.
app.get('/videoplayer', (req, res) => {
})
The video tag in the “index.html” file has an attribute “src=”/videoplay”” to play the video using “/videoplay” route.
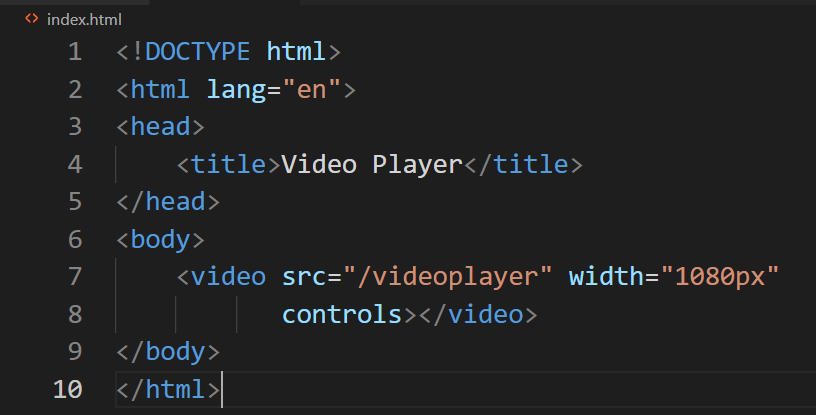
Fetched the range of the video:
const range = req.headers.range;
This value is used to calculate the starting position.
Getting the path of the video which we want to play:
const videoPath = './video.mp4';
Calculating size:
const videoSize = fs.statSync(videoPath).size;
Here we use the File System Module to calculate the video size, which is used to get the end position.
Set chunk size:
const chunkSize = 1 * 1e6;
Calculate the start and end position of the video:
const start = Number(range.replace(/\D/g, ""));
const end = Math.min(start + chunkSize, videoSize - 1);
These values are passed when creating the readable streams for the video.
Calculate the total content-length:
const videoLength = end - start + 1;
The total length will attach to the header.
Set the header with the following properties:
const headers = {
"Content-Range": `bytes ${start}-${end}/${videoSize}`,
"Accept-Ranges": "bytes",
"Content-Length": videoLength,
"Content-Type": "video/mp4"
}
res.writeHead(206, headers)
Create a readable stream and pipe it with the response:
const stream = fs.createReadStream(videoPath, { start, end })
stream.pipe(res)
Complete Code for a Video Streaming Application in NodeJS
const express = require('express');
const fs = require('fs');
const app = express();
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
})
app.get('/videoplayer', (req, res) => {
const range = req.headers.range
const videoPath = './video.mp4';
const videoSize = fs.statSync(videoPath).size
const chunkSize = 1 * 1e6;
const start = Number(range.replace(/\D/g, ""))
const end = Math.min(start + chunkSize, videoSize - 1)
const contentLength = end - start + 1;
const headers = {
"Content-Range": `bytes ${start}-${end}/${videoSize}`,
"Accept-Ranges": "bytes",
"Content-Length": contentLength,
"Content-Type": "video/mp4"
}
res.writeHead(206, headers)
const stream = fs.createReadStream(videoPath, {
start,
end
})
stream.pipe(res)
})
app.listen(3000);
Output
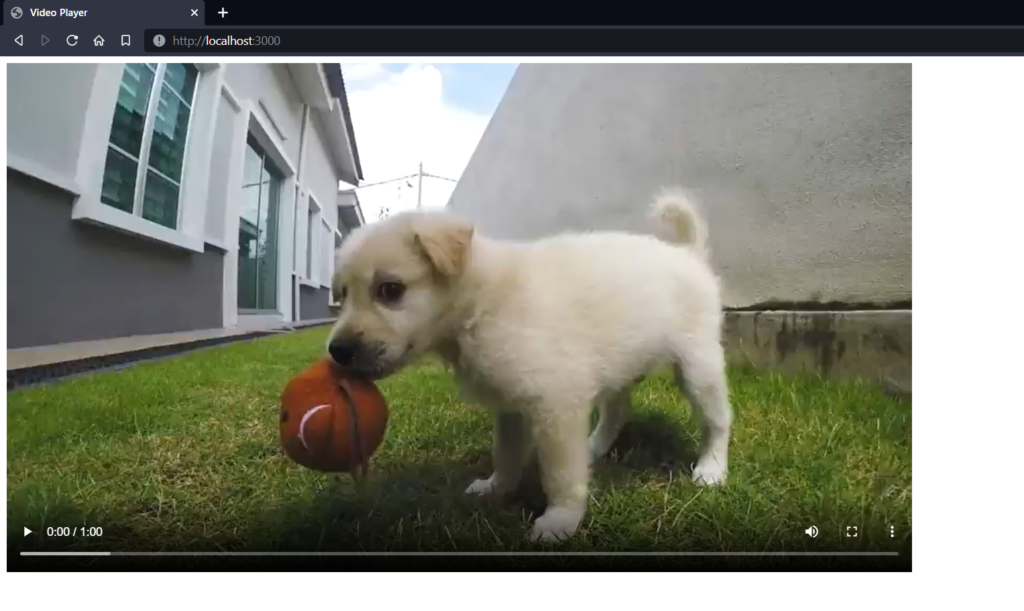
Summary
A Video Streaming Application can easily build using NodeJS to stream different kinds of video, we can use Expres to set up a home route to send an HTML file containing a video tag that contains the video source as another route where we can write functionality to play the available videos. Hope this tutorial teaches you the step-by-step guide to creating a video streaming application in NodeJS.
Reference
https://www.npmjs.com/package/express