Autofill is a feature that allows a web form to automatically complete input fields based on previously entered or saved data. For example, when we start typing our name in a form, the browser might suggest our full name, email address, or phone number, which we can quickly select without the need to retype them.
Why Do We Need Autofill?
Autofill is important because:
- Saves time: Users don’t have to manually enter the same information again and again.
- Improves user experience: It can be tedious to fill out long forms, if the page is reloaded by mistake, all the details get lost hence in such situations, autofill makes it easier and faster for users to complete forms.
- Reduces errors: Less manual input reduces the chance of making mistakes.
Let’s look at how to autofill forms using JavaScript.
How to Autofill a Form?
Here is the basic approach we will follow to provide an autofill in our code:
- Identify Form Elements: Each form element (e.g., text input, checkbox, etc.) will have an ID, name, or other attribute that we can use to target it.
- Set the Value: Using JavaScript, you can set the value of the input fields by accessing the element and assigning it a value.
- Saving Data in localStorage: When we submit the form, the values of the input fields are saved in the browser’s localStorage, allowing us to remember the previously entered data.
- Suggestions appear on Hover/Focus: A small box pops up when the user moves over or clicks on the input fields. This box shows the saved data and offers to fill in the field automatically.
- Detect Enter Key Press: We will add event listeners to detect the Enter key (using key down) while the input field is focused. If the user presses ‘Enter,’ the field will automatically fill with the saved value from localStorage.
- Hide Suggestion on Blur: When the input field is no longer clicked on, the suggestion box disappears to keep the form tidy.
Want to learn how we can get form data in Node.js? Click here to explore!
Steps to Add Autofill to a Form
Step 1: Start with the Basic HTML Form
We need a form where users can enter their details. Here’s the basic structure for our form:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Autofill on Hover</title>
</head>
<body>
<form id="userForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name" autocomplete="off"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" autocomplete="off"><br><br>
<label for="phone">Phone:</label>
<input type="tel" id="phone" name="phone" autocomplete="off"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
- This code sets up a simple form with three fields: name, email, and phone.
- autocomplete=”off” is used to disable the browser’s default autofill feature, so we can implement our custom version.
Output:
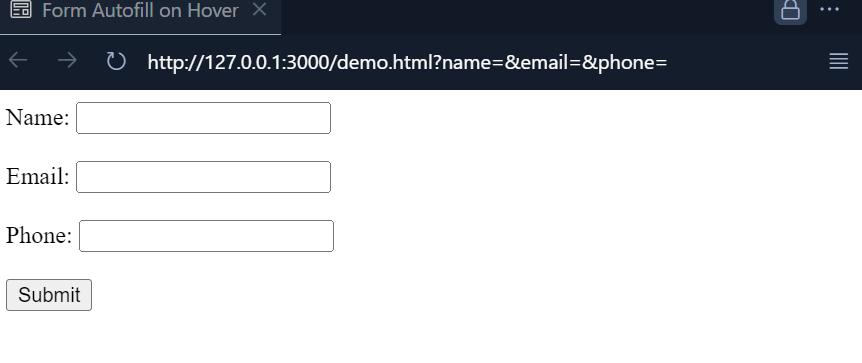
Step 2: Add Suggestion Boxes for Each Field
Next, we create a suggestion box for each input field. This box will show the saved data for autofill if it exists.
<div id="nameSuggestion" class="suggestion-box"></div>
<div id="emailSuggestion" class="suggestion-box"></div>
<div id="phoneSuggestion" class="suggestion-box"></div>
These divs are added right after each input field. Now, let’s style these boxes.
Step 3: Add CSS to Style the Suggestion Boxes
<style>
.suggestion-box {
display: none;
position: absolute;
border: 1px solid #ccc;
background-color: #f9f9f9;
padding: 5px;
z-index: 10;
font-size: 14px;
margin-top: -20px;
}
input {
position: relative;
margin-bottom: 30px;
}
</style>
The .suggestion-box is hidden at first (using display: none) but will show up when we need to offer an autofill suggestion. It will appear just below the input fields, with a simple border and background color to make it easy to see.
Step 4: Implement JavaScript to Save and Autofill Data
Now, let’s add the JavaScript logic to save form data and display suggestions.
<script>
document.getElementById('userForm').addEventListener('submit', function(event) {
event.preventDefault();
localStorage.setItem('name', document.getElementById('name').value);
localStorage.setItem('email', document.getElementById('email').value);
localStorage.setItem('phone', document.getElementById('phone').value);
alert('Form data saved!');
});
</script>
- The submit event listener waits for the form to be submitted. Instead of sending the form, it saves the input values to localStorage.
- localStorage keeps key-value pairs in the browser, so the data can be reused when the user comes back.
Step 5: Show the Autofill Suggestion
Next, we need to display the saved data as suggestions when the user focuses on an input field.
<script>
function showSuggestion(inputId, suggestionId, fieldName) {
let suggestionBox = document.getElementById(suggestionId);
let storedValue = localStorage.getItem(fieldName);
if (storedValue) {
suggestionBox.style.display = 'block';
suggestionBox.textContent = `Press Enter to autofill: ${storedValue}`;
}
}
</script>
- This function shows the suggestion box when the user focuses on an input field.
- It gets the stored value from localStorage and displays it in the suggestion box if the data is available.
Step 6: Autofill the Field When User Presses Enter
Let’s add the functionality to allow users to autofill the input field by pressing the Enter key.
<script>
function autofillOnEnter(inputId, suggestionId, fieldName) {
let inputElement = document.getElementById(inputId);
let storedValue = localStorage.getItem(fieldName);
inputElement.addEventListener('keydown', function(event) {
if (event.key === 'Enter' && storedValue) {
event.preventDefault(); // Prevent form submission
inputElement.value = storedValue; // Autofill the field
document.getElementById(suggestionId).style.display = 'none';
}
});
}
</script>
This function checks for the Enter key while the user is typing in the input field. If the user presses Enter and there’s data saved in localStorage, it automatically fills the field with that data.
Step 7: Attach the Event Listeners
We now need to attach our functions to the focus and keydown events for each input field.
<script>
document.getElementById('name').addEventListener('focus', function() {
showSuggestion('name', 'nameSuggestion', 'name');
});
autofillOnEnter('name', 'nameSuggestion', 'name');
document.getElementById('email').addEventListener('focus', function() {
showSuggestion('email', 'emailSuggestion', 'email');
});
autofillOnEnter('email', 'emailSuggestion', 'email');
document.getElementById('phone').addEventListener('focus', function() {
showSuggestion('phone', 'phoneSuggestion', 'phone');
});
autofillOnEnter('phone', 'phoneSuggestion', 'phone');
</script>
We add a focus event listener to each input field to show suggestions when the user clicks on a field. We also call autofillOnEnter() for each field to let the field fill automatically when the user presses Enter.
Step 8: Hide Suggestion Box When Input Field Loses Focus
To keep things clean, we’ll hide the suggestion box when the user clicks away from an input field.
<script>
function hideSuggestion(suggestionId) {
document.getElementById(suggestionId).style.display = 'none';
}
document.getElementById('name').addEventListener('blur', function() {
hideSuggestion('nameSuggestion');
});
document.getElementById('email').addEventListener('blur', function() {
hideSuggestion('emailSuggestion');
});
document.getElementById('phone').addEventListener('blur', function() {
hideSuggestion('phoneSuggestion');
});
</script>
When the input field is no longer clicked on (the blur event), we hide the suggestion box. This makes it easier for users and keeps the form looking neat.
Complete Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Autofill on Hover</title>
<style>
.suggestion-box {
display: none;
position: absolute;
border: 1px solid #ccc;
background-color: #f9f9f9;
padding: 5px;
z-index: 10;
font-size: 14px;
margin-top: -20px;
}
input {
position: relative;
margin-bottom: 30px;
}
</style>
</head>
<body>
<form id="userForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name" autocomplete="off"><br><br>
<div id="nameSuggestion" class="suggestion-box"></div>
<label for="email">Email:</label>
<input type="email" id="email" name="email" autocomplete="off"><br><br>
<div id="emailSuggestion" class="suggestion-box"></div>
<label for="phone">Phone:</label>
<input type="tel" id="phone" name="phone" autocomplete="off"><br><br>
<div id="phoneSuggestion" class="suggestion-box"></div>
<input type="submit" value="Submit">
</form>
<script>
document.getElementById('userForm').addEventListener('submit', function(event) {
event.preventDefault();
localStorage.setItem('name', document.getElementById('name').value);
localStorage.setItem('email', document.getElementById('email').value);
localStorage.setItem('phone', document.getElementById('phone').value);
alert('Form data saved!');
});
function showSuggestion(inputId, suggestionId, fieldName) {
let suggestionBox = document.getElementById(suggestionId);
let storedValue = localStorage.getItem(fieldName);
if (storedValue) {
suggestionBox.style.display = 'block';
suggestionBox.textContent = `Press Enter to autofill: ${storedValue}`;
}
}
function autofillOnEnter(inputId, suggestionId, fieldName) {
let inputElement = document.getElementById(inputId);
let storedValue = localStorage.getItem(fieldName);
inputElement.addEventListener('keydown', function(event) {
if (event.key === 'Enter' && storedValue) {
event.preventDefault(); // Prevent form submission
inputElement.value = storedValue; // Autofill the field
document.getElementById(suggestionId).style.display = 'none';
}
});
}
document.getElementById('name').addEventListener('focus', function() {
showSuggestion('name', 'nameSuggestion', 'name');
});
autofillOnEnter('name', 'nameSuggestion', 'name');
document.getElementById('email').addEventListener('focus', function() {
showSuggestion('email', 'emailSuggestion', 'email');
});
autofillOnEnter('email', 'emailSuggestion', 'email');
document.getElementById('phone').addEventListener('focus', function() {
showSuggestion('phone', 'phoneSuggestion', 'phone');
});
autofillOnEnter('phone', 'phoneSuggestion', 'phone');
function hideSuggestion(suggestionId) {
document.getElementById(suggestionId).style.display = 'none';
}
document.getElementById('name').addEventListener('blur', function() {
hideSuggestion('nameSuggestion');
});
document.getElementById('email').addEventListener('blur', function() {
hideSuggestion('emailSuggestion');
});
document.getElementById('phone').addEventListener('blur', function() {
hideSuggestion('phoneSuggestion');
});
</script>
</body>
</html>
Output:
Conclusion
Autofilling forms with JavaScript can save users time and effort when they have to fill out the same information repeatedly. Whether we are working on a project that needs to automatically fill in data or just want to make our form easier to use, this method is simple to set up.
How to Read a Local Text File Using JavaScript? Click here to find out!
Reference
https://stackoverflow.com/questions/54615342/use-javascript-to-automatically-fill-in-html-forms