We often delete all rows to clear the table, update it with new data, or reset it when needed. There are a few different approaches that we can use to delete all rows from an HTML table using JavaScript. Let’s see the best three of them with code examples.
Understanding Table Structure
An HTML table consists of multiple rows (<tr> elements) inside a table body (<tbody>). Each row contains one or more cells (<td> elements). If we want to delete all the rows in a table, we need to remove all the <tr> elements within the <tbody>.
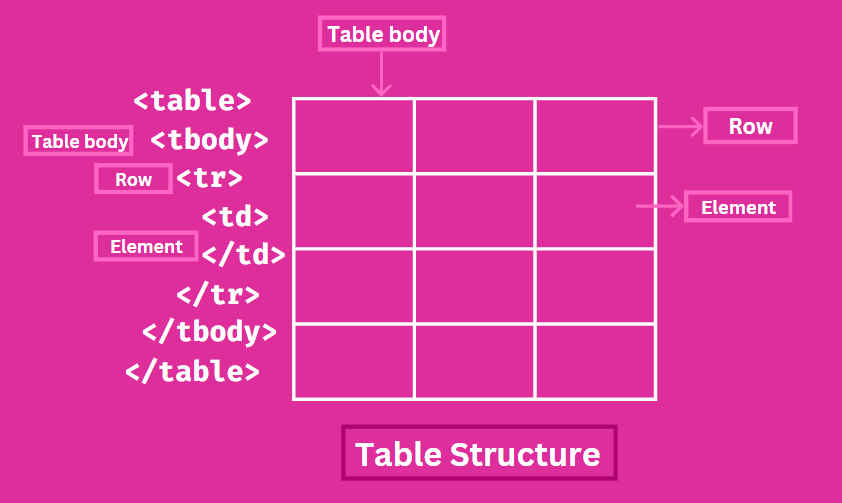
Here is a simple example of a table:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Styled Table</title>
<style>
#myTable {
width: 50%;
border-collapse: collapse;
margin: 20px auto;
outline: 2px solid rgb(255, 39, 201);
font-family: Arial, sans-serif;
}
#myTable th {
background-color: #ec458a;
padding: 12px;
text-align: left;
border-bottom: 1px solid #ddd;
}
#myTable td {
padding: 12px;
border-bottom: 1px solid #ddd;
}
#myTable tr:nth-child(even) {
background-color: #ec458a; /* Light background for even rows */
}
</style>
</head>
<body>
<table id="myTable">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>Snigdha</td>
<td>20</td>
</tr>
<tr>
<td>Hitesh</td>
<td>21</td>
</tr>
<td>Aditya</td>
<td>25</td>
</tr>
</tbody>
</table>
</body>
</html>
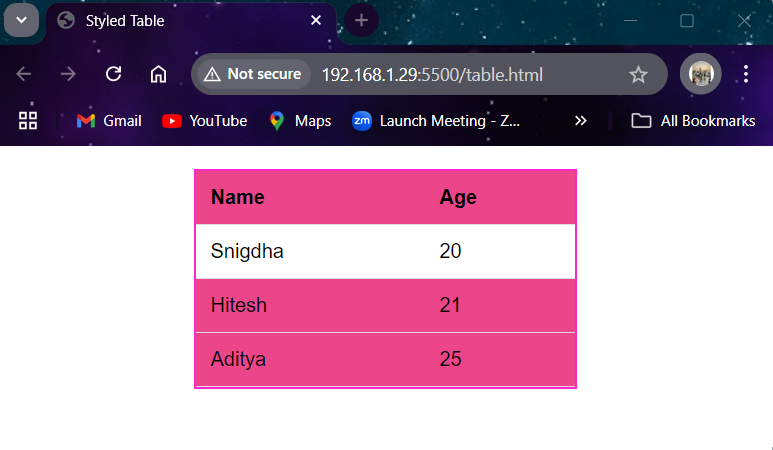
In this table, there are three rows in the <tbody> that we want to delete.
Why Deleting All Rows in a Table
We need to delete all rows from a table when we want to:
- Update Data: If we want to refresh the table with new data, we need to remove the old rows first.
- Clear the Table: When a user clicks the ‘Clear’ button, we might need to empty the table.
- Reset State: After a search or filter operation, we might need to clear the table before showing new results.
Would you like to learn how to convert a JavaScript NodeList to an array? Click here to find out!
Different Methods to Remove All Rows in JavaScript
1. The deleteRow Method
The deleteRow method is used to delete a specific row from a table. We can use this method in a loop to delete all rows.
Example:
We create a ‘Clear’ button on our webpage containing the table (Table 1.1) we previously created. This is the script or logic we add to clear all the rows.
<button id="clearButton">Clear</button>
<script>
function clearTable() {
const tableBody = document.querySelector('#myTable tbody');
const rowCount = tableBody.rows.length; // Get the number of rows
// Loop through rows in reverse and delete each one
for (let i = rowCount - 1; i >= 0; i--) {
tableBody.deleteRow(i);
}
}
document.getElementById('clearButton').addEventListener('click', clearTable);
</script>
Explanation:
- The deleteRow method removes rows based on their index.
- The loop starts from the last row and goes backwards. When we loop in forward, the position (index) of the remaining rows would change after each deletion. So to prevent this issue, we loop in reverse.
Output:
2. The removeChild Method
In this method, we remove each row (<tr>) from the <tbody> using the removeChild method. First, we get the <tbody> element. Then, we loop through the rows and use removeChild to delete each one from the <tbody>. In simple terms, it’s a way to take out each row from the table one by one.
Example:
<script>
function clearTable() {
const tableBody = document.querySelector('#myTable tbody');
// Loop through rows and remove them
while (tableBody.firstChild) {
tableBody.removeChild(tableBody.firstChild);
}
}
document.getElementById('clearButton').addEventListener('click', clearTable);
</script>
Explanation:
- The clearTable function now uses a while loop to remove each row from the <tbody> until no rows are left.
- The condition tableBody.firstChild checks if there are any child nodes left to remove.
- When we click the “Clear” button, all rows in the table will be removed one by one.
Output:
3. The innerHTML Property
To clear the content of an element, we can use the innerHTML property. To delete all rows, we will set the innerHTML of the <tbody> to an empty string (“”). This will clear all the rows.
Example:
<script>
function clearTable() {
const tableBody = document.querySelector('#myTable tbody');
tableBody.innerHTML = '';
}
document.getElementById('clearButton').addEventListener('click', clearTable);
</script>
Explanation:
- The line const tableBody = document.querySelector(‘#myTable tbody’); selects the <tbody> of the table.
- When the “Clear” button is clicked, tableBody.innerHTML = ”; removes all rows by replacing the content with an empty string.
Output:
Conclusion
All the methods we discussed above can help you remove all the rows from an HTML table. You can choose a method based on your needs. The innerHTML method is simple and easy to use, but it won’t keep any events associated with the rows. The removeChild method gives you more control. The deleteRow method is ideal when you want to remove specific rows.
Learn the difference between Java and JavaScript by clicking here!
Reference
https://stackoverflow.com/questions/7271490/delete-all-rows-in-an-html-table