Who says that JavaScript does not support object-oriented programming concepts? Classes in JavaScript help us create organized and encapsulated code. They allow us to use objects based on templates. Let’s learn more about JavaScript classes and see how to create and use them.
Introduction to JavaScript Classes
ES6 introduced JavaScript classes, which makes it easy to work with classes. It is important to know that classes are not objects but a blueprint for an object. A class is a template for creating objects. This means that when we create a class and add methods and properties to it, the class acts like a mold, from which we can create objects. These objects have the same properties but can have different values. Using one class, we can make many objects.
How to Create a Class
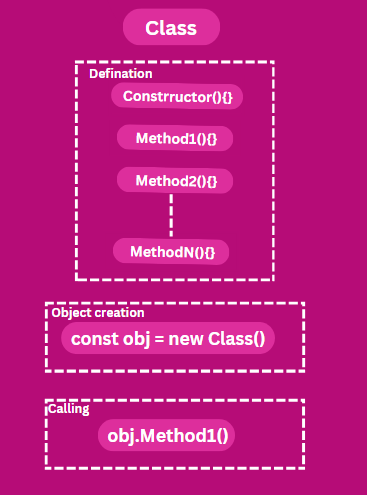
1. Define a Class
We use the keyword class to create a class. A class is defined like this:
class Student {
constructor() {}
}
Here, Student is the name of the class.
2. Add a Constructor
It is mandatory to put a constructor() inside a class. A constructor is a method that helps to add parameters and initialise our objects.
For example, a Student will have some properties, say, name, age, and roll number. So we include these as parameters in the constructor and use this keyword to refer to the properties of the currently created object of the Student class:
class Student {
constructor(name, age, roll_no) {
this.name = name;
this.age = age;
this.roll_no = roll_no;
}
}
If we do not define a constructor method, then JavaScript will add an empty constructor by default. There can be only one constructor in a class, or else the compiler will throw a syntax error.
3. Create an Instance
Now that we have the template (class) ready, we will create an instance (object) of it. This is done using the new keyword, like this:
const sam = new Student("Sam", 12, 109);
Here, sam is the name of the object we want to create from the student class. We place the name of the class after the new keyword, and under the braces, we pass the value of the parameters of our object.
4. Add Class Methods
Now that we have created an object, let’s design some actions our object can perform using methods. We will call these methods later. All the methods, except the constructor, are class methods. These methods are like functions.
For example, if we want to calculate the student’s birth year using the age parameter, we can create a method like this:
class Student {
constructor(name, age, roll_no) {
this.name = name;
this.age = age;
this.roll_no = roll_no;
}
birthYear() {
const currentYear = new Date().getFullYear();
return currentYear - this.age;
}
call() {
console.log(`My name is ${this.name}, I am ${this.age} years old, my serial number is ${this.roll_no}, and my birth year is ${this.birthYear()}.`);
}
}
const sam = new Student("Sam", 12, 109);
sam.call();
In the given code, we have defined two methods: birthyear() and call(). The birthyear() function calculates the birth year of the student using the age of the student. The call() method displays the details of students in a sentence.
You can also see that the birthyear() function is called inside the call() method by using this keyword. Now to display the student’s details, we call the object (sam) along with the call method like sam.call(). This displays the details of the student on the console.

If you are curious to know about the Date() used in this code, then click here!
5. Add a Parameterized Class Method
We can also build a parameterized method that takes a parameter and returns the result according to it.
For example, if we wanted to calculate the result status of the student based on his marks, we can write the code this way:
class Student {
constructor(name, age, roll_no) {
this.name = name;
this.age = age;
this.roll_no = roll_no;
}
birthYear() {
const currentYear = new Date().getFullYear();
return currentYear - this.age;
}
result(marks) {
return marks > 35 ? `${this.name} has passed.` : `${this.name} has failed.`;
}
call() {
console.log(`My name is ${this.name}, I am ${this.age} years old, my serial number is ${this.roll_no}, and my birth year is ${this.birthYear()}.`);
}
}
const sam = new Student("Sam", 12, 109);
sam.call();
console.log(`${sam.result(45)}`);

Inheritance in JavaScript
Inheritance is a way to create a new class based on an existing one. The new class inherits properties and methods from the existing class, allowing it to reuse and extend its functionality.
In inheritance, we have two types of classes:
- Parent class: The superclass from which the properties are being inherited.
- Child class: It is the subclass that inherits the properties from the parent class.
Suppose we have a vehicle class that has a brand name, and we want to create another class car that would inherit the brand name from the vehicle class. Then we can code it this way:
class Vehicle {
constructor(brand) {
this.brand = brand;
}
drive() {
console.log(`${this.brand} is driving.`);
}
}
class Car extends Vehicle {
constructor(brand, doors) {
super(brand);
this.doors = doors;
}
drive() {
console.log(`${this.brand} car with ${this.doors} doors is driving.`);
}
}
const myCar = new Car("BMW", 4);
myCar.drive();
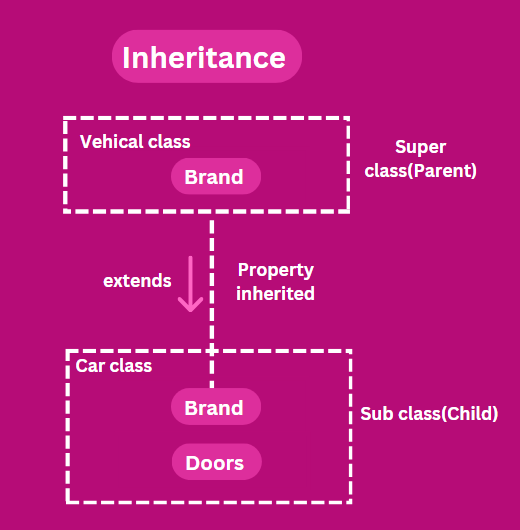
In this code, the extends keyword is used to create a Car class that inherits properties and methods from the Vehicle class. The super keyword will invoke the constructor of the class Vehicle to initialize the property brand in the Car class. Indeed, the drive method of the Car class does override that in the Vehicle class to provide specific behaviour for Car objects.

Conclusion
The class is necessary for grouping data together with the code. This makes it easier to work with objects and adheres to object-oriented programming principles in order to achieve well-structured code. A class defined once can have a lot of instances, called objects. It reduces code length and supports reusability. Besides, classes make use of inheritance, thereby increasing their reusability even more.
Also Read: JavaScript Object
Reference
https://stackoverflow.com/questions/12610394/javascript-classes