Next.js comes with many new updates and one of them is the way to store static files. In this article, we will see what static files are, where they should be stored, and how to render and access images, fonts, JSON, and other files for better performance in Next.js. So let’s get started.
Check out our previous tutorial: How to Set Up and Use Tailwind CSS with Next.js
Next.js Static File Serving
Static files are files that are not frequently changed, such as images, fonts, JSON, videos, documents, etc. These files should be stored and used according to the Next.js guide.
To server static files in Next.js, we can create a folder ‘public’ inside the root of the project folder. These files can then be imported directly into the routes in the app directory, without having to specify the full path or worry about indentation, etc.
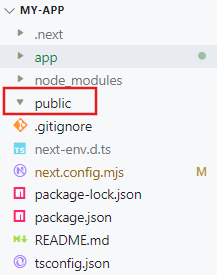
It can be accessed directly without including the public prefix. For example, if we have a file located at ‘public/fileName’, we can access it directly by using the URL ‘/fileName’.
Note: Next.js will only serve these static files if they are in the public directory during the build time, which means, any files added at the request time can’t be accessible.
Storing and Accessing Images in Next.js
Let’s say we have an image called dog.jpg and we want to render it to the home page.
Step 1: Create a folder named ‘public’ in the root of the project folder.
Step 2: Create another folder named ‘images’ in the public folder for better structure.
Step 3: Paste the file dog.jpg inside ‘images’.
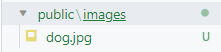
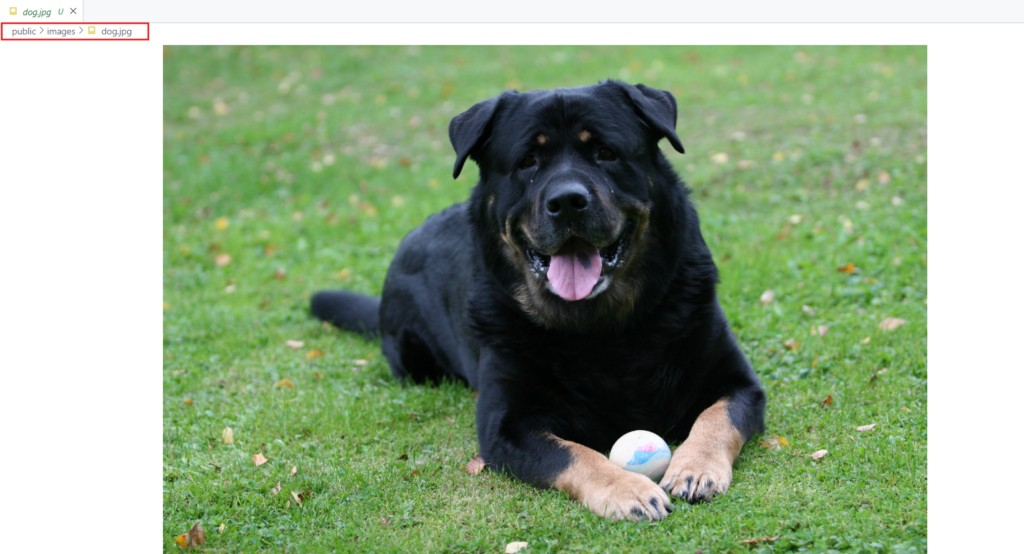
Step 4: Now use the ‘/images/dog.jpg’ path to access that file.
Step 5: Instead of <img> tag, use <Image> component from next to render this image.
import Image from 'next/image';
const HomePage = () => {
return <Image src="/images/dog.jpg" alt="Dog" width={200} height={200} />
};
export default HomePage;
Output:
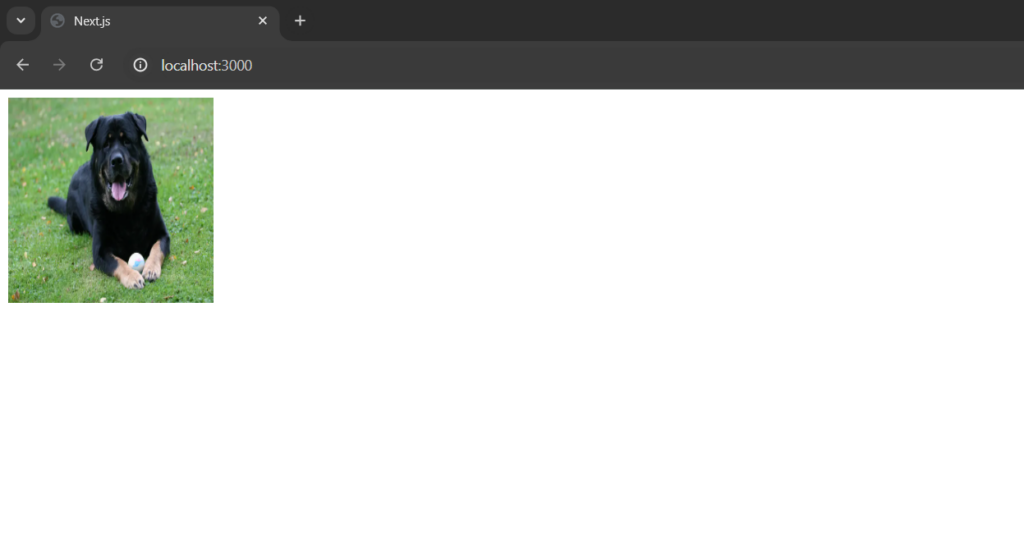
And that’s it.
Storing and Accessing Fonts in Next.js
External fonts can be another common type of static file that we can store in the public/fonts directory and use in CSS directly.
Let’s say you have a font file OpenSans-Regular.ttf.
Step 1: Store the OpenSans-Regular.ttf file in the public/fonts directory.
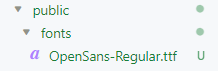
Step 2: Create a file styles/globals.css inside the app directory.
Step 3: Import this file in the root layout.
import './styles/globals.css';
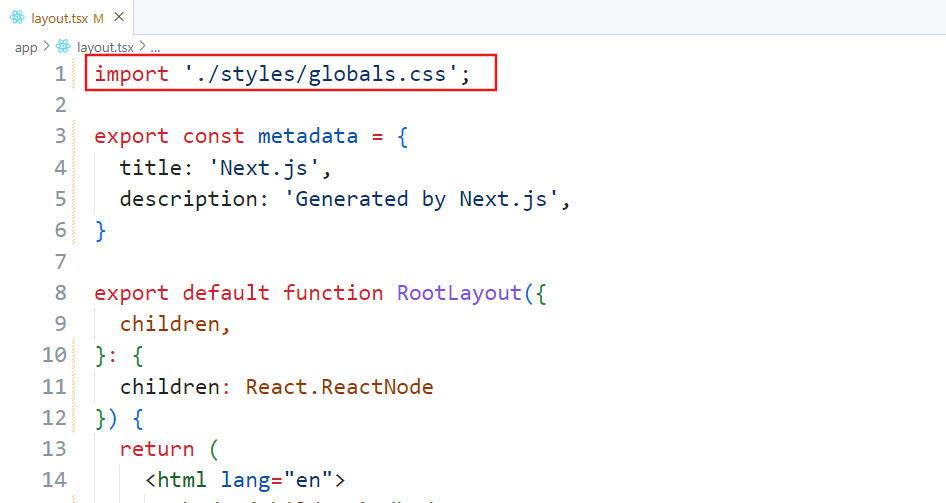
Step 4: Inside the CSS file use the @font-face syntax to import the font.
@font-face { }
Inside the @font-face rule, use font-family and pass a name for the font. This name will be used to call the font anywhere in CSS.
@font-face {
font-family: 'OpenSans';
}
Then use ‘src’ to define the location of the font file. Inside it, we used the ‘url’ function to give the path of the font file and the ‘format’ function to define the font format like truetype, opentype, woff, etc.
@font-face {
font-family: 'OpenSans';
src: url('/fonts/OpenSans-Regular.ttf') format('truetype');
}
Step 5: Now we can use it wherever we want.
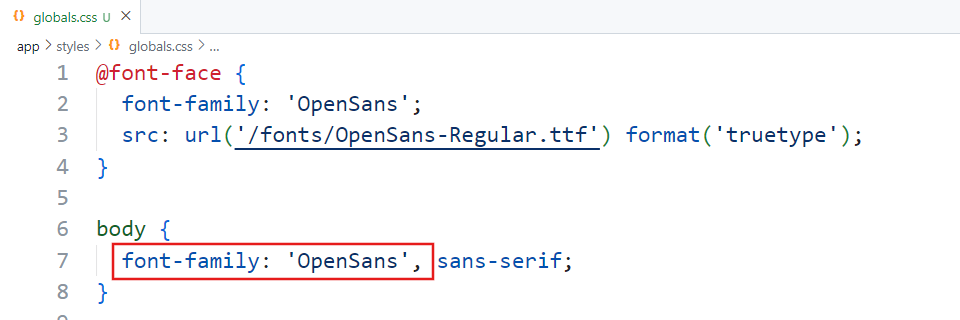
Before:
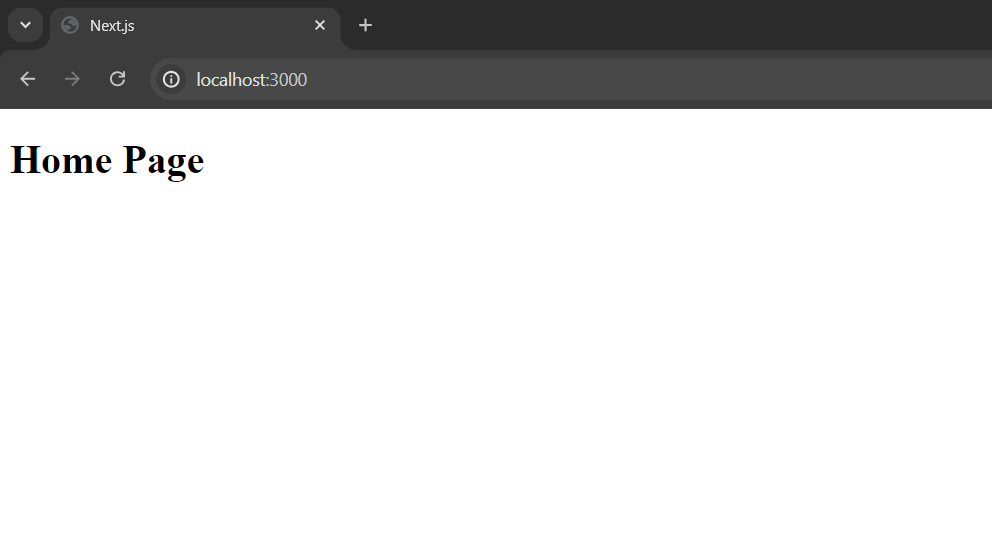
After:
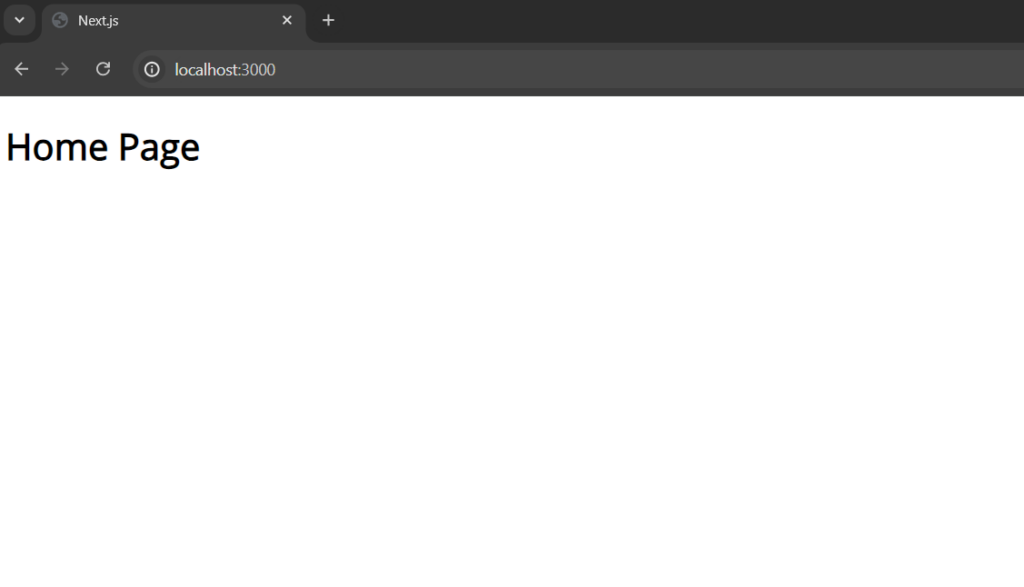
This ensures that the custom fonts are loaded correctly and can be applied to any page, layout or component file of the application.
Storing and Accessing JSON Files in Next.js
Let’s say we have a JSON file data.json.
Step 1: Store the data.json file in the public/data directory.
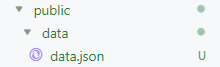
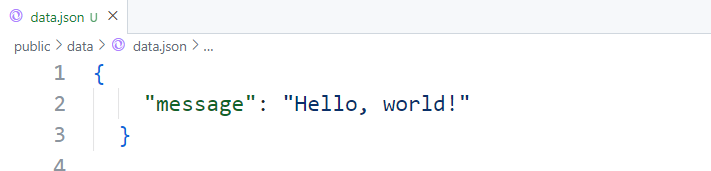
Step 2: Use the Fetch API and pass the base URL with ‘/data/data.json’ to get JSON data and display it in any file in the app directory.
export default async function Home() {
const baseUrl = process.env.NEXT_PUBLIC_BASE_URL || 'http://localhost:3000';
const res = await fetch(`${baseUrl}/data/data.json`);
const data = await res.json();
return (
<div>
<h1>{data.message}</h1>
</div>
);
}
For an in-depth tutorial on data fetching: Data Fetching in Next.js
Output:
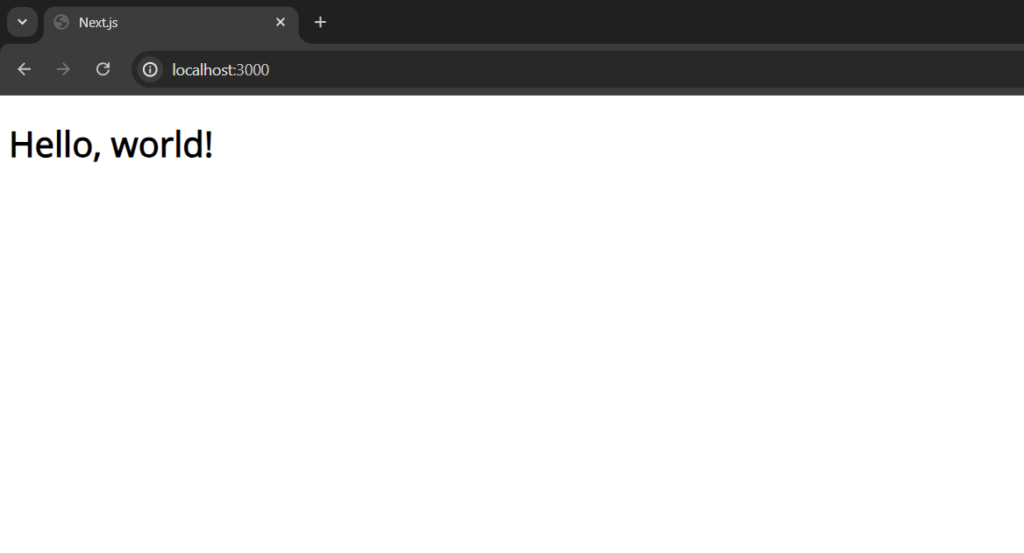
In the same way, we can also server videos using the HTML <video> and <source> tags and documents using the <a> tag.
*What’s Next?
Summary
In short, in Next.js we can store static files in a public directory at the root level of the project so that they can be efficiently accessed using simple URLs. In this way, we can store any static file like images, fonts, JSON, etc to ensure better performance and easy access.
Reference
https://nextjs.org/docs/app/building-your-application/optimizing/static-assets