Now that we have covered all the basic topics of Next.js in our series, it’s time to learn how to style our Next.js application.
Learning how to style a Next.js application is a part of front-end development that cannot be skipped. In Next.js we can do styling in different ways: Global CSS, CSS Modules, Tailwind CSS, CSS-in-JS, and Sass.
In this tutorial, we will cover styling using Global CSS and CSS Modules, not just an overview but with in-depth explanations.
Previous Tutorial: Pre-rendering and Data Fetching in Next.js
Styling Next.js Application Using CSS Modules
In Next.js, we can easily use CSS by writing our code on a file with “.module.css” extension. So just like with HTML, we create a file “styles.css”, here we can create a file “styles.module.css”.
Detailed Step-by-Step Instructions:
Step 1: Create a new Next.js application.
npx create-next-app@latest
Check Yes for both TypeScript and App Router to follow along with us.
If you have any problems using Next.js, see Next.js Installation and Getting Started with Next.js.
Step 2: Open the project inside a code editor -> remove all the files inside the app directory -> create a file page.tsx for the ‘/’ route.
app/page.tsx:
const home = () => {
return <h1>Home Page</h1>;
};
export default home;
Step 3: Create a folder about inside the app directory with a page.tsx file to create a new route ‘/about’.
app/about/page.tsx:
const about = () => {
return <h1>About Page</h1>;
};
export default about;
Now let’s apply different styles to both the home and about pages using CSS modules.
Step 4: Create a file styles.module.css inside the app directory for the home page.
app/styles.module.css:
.homePage {
color: blue;
font-size: 36px;
text-align: center;
margin-top: 50px;
}
Then we can import it and use the “className={styles.name-of-class}” to apply it to the respective elements.
app/page.tsx:
import styles from './styles.module.css';
const Home = () => {
return <h1 className={styles.homePage}>Home Page</h1>;
};
export default Home;
Step 5: Similarly create a file styles.module.css inside the app/about directory.
app/about/styles.module.css:
.aboutPage {
color: green;
font-size: 36px;
text-align: center;
margin-top: 50px;
}
We can then apply this to the about page.
app/about/page.tsx:
import styles from './styles.module.css';
const About = () => {
return <h1 className={styles.aboutPage}>About Page</h1>;
};
export default About;
Step 6: Execute the below command to run our app.
npm run dev
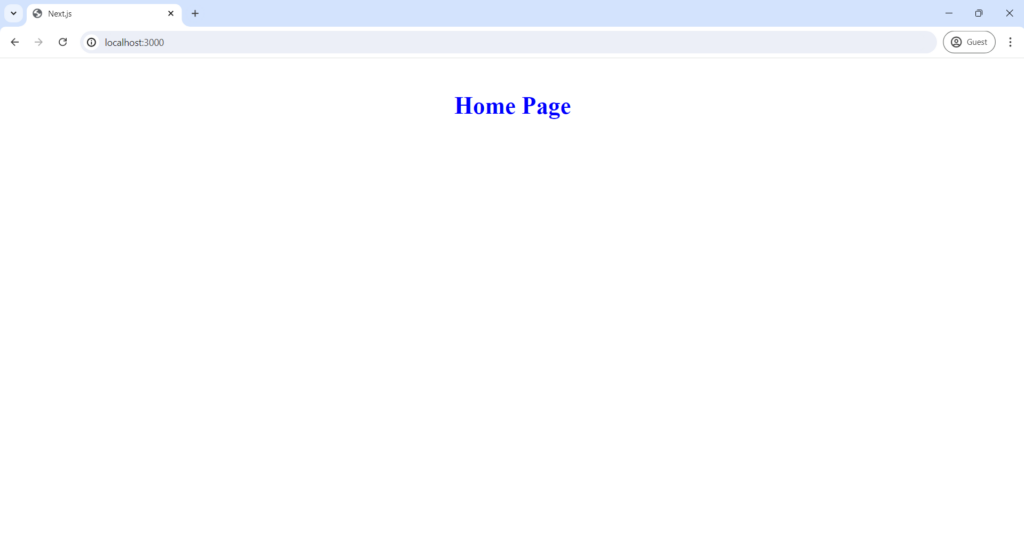
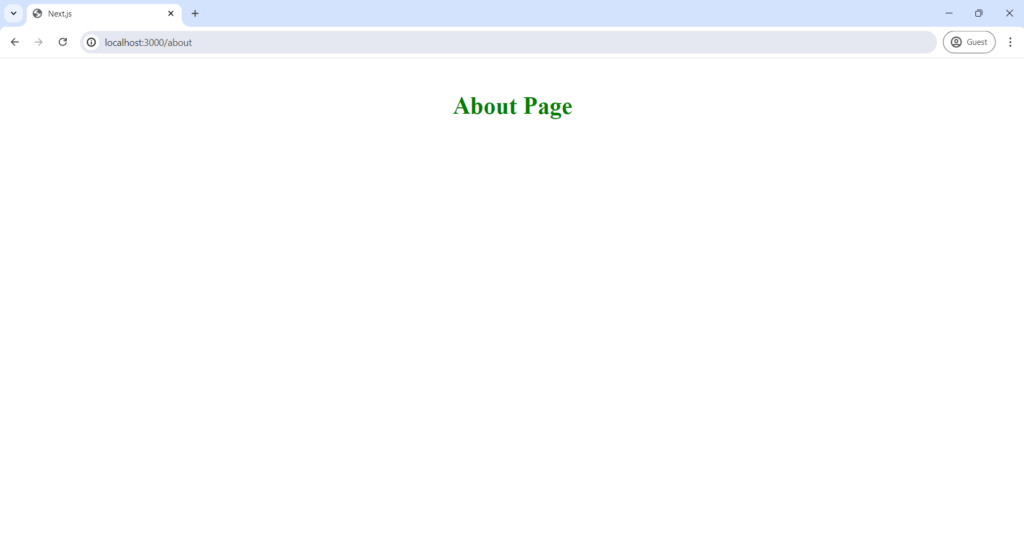
Applying Global CSS in Next.js
We can create different style files for different pages using CSS modules, but if we have to apply some style on every page of the application, then we can create a global.css inside the app directory and import it into the root layout to apply to every route. We don’t even need to import it in each individual file.
app/global.css:
body {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Arial, sans-serif;
}
h1 {
text-decoration: underline;
}
app/layout.tsx:
In ‘app/layout.tsx’, just add an importing statement “import ‘./global.css;’ at the top without changing the default code.
import './global.css';
export default function RootLayout({ children }: { children: React.ReactNode}) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
Now if you check the application again the same style gets applied on every page:
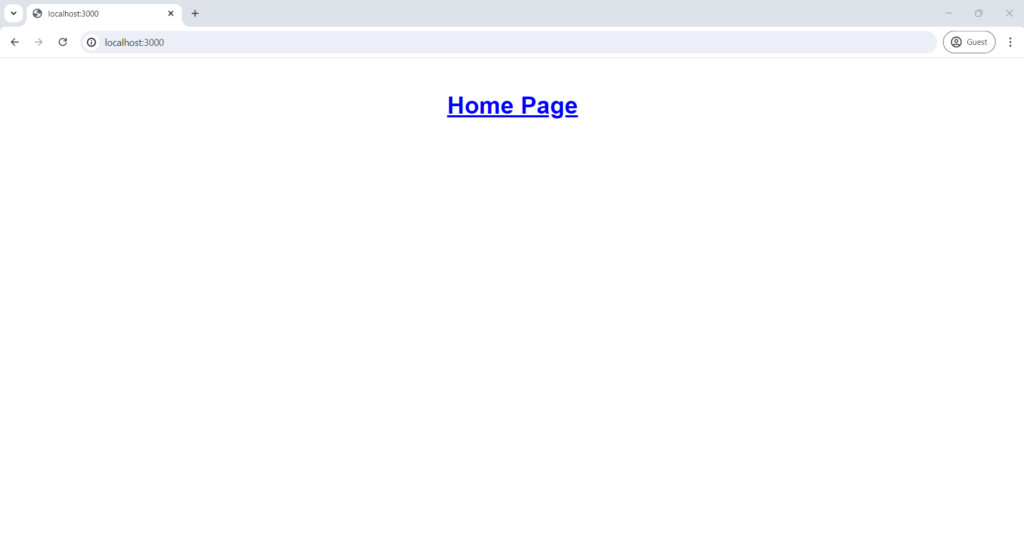
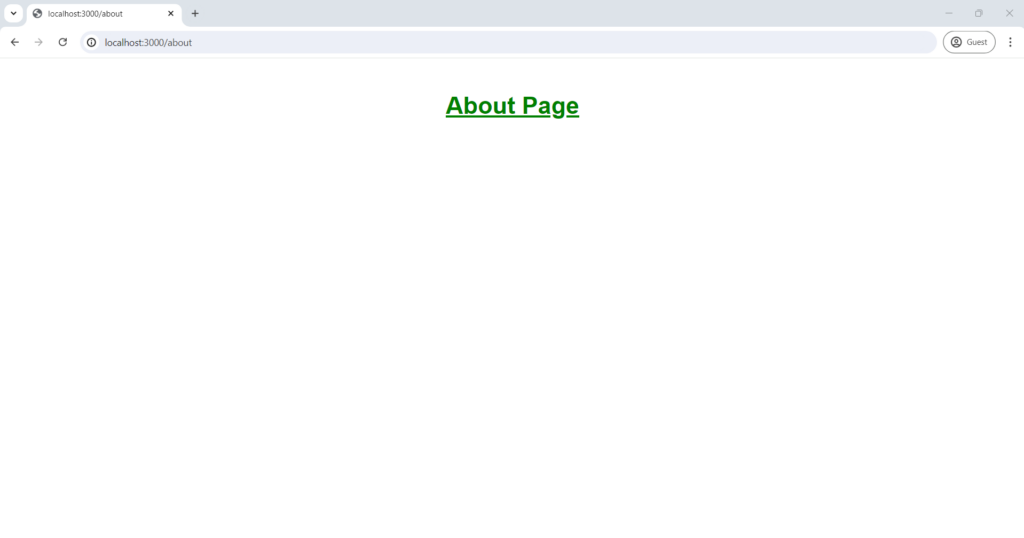
Since we are using the app directory we can import this global CSS in any file, whereas in the case of the pages directory, we can only import global styles inside in _app.js file.
Using External Stylesheets
In the same way, we can also use stylesheets by external packages in Next.js by importing them in any file in the app directory, like in “app/layout.txs” to apply to each page and for individual page in their “page.tsx” file.
For example, let’s install Bootstrap:
npm install bootstrap
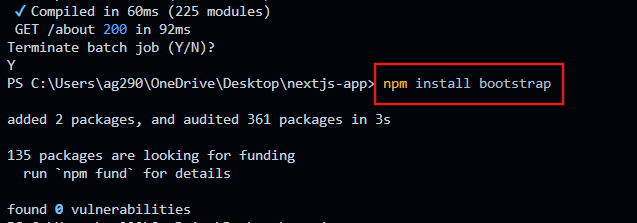
Import it into ‘app/layout.tsx’ to apply it to every page:
import 'bootstrap/dist/css/bootstrap.min.css';
export default function RootLayout({ children }: { children: React.ReactNode}) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
Bootstrap gets applied:
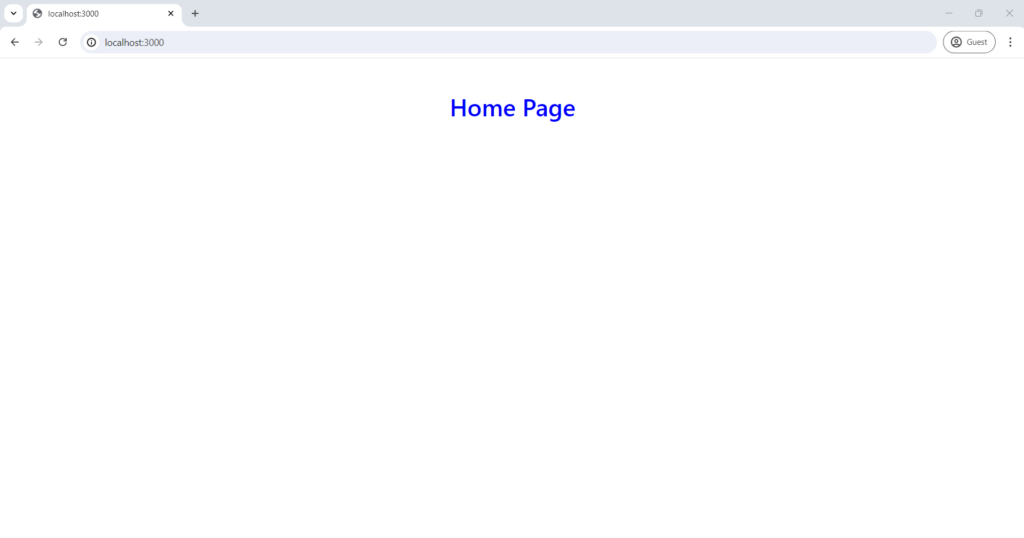
*What’s Next?
Summary
In short, Next.js is compatible with CSS using CSS modules which we can implement by creating a ‘styles.module.css’ file in the relevant route folder and importing it using ‘import styles from ‘./styles.module.css’;’. We can also create global CSS by creating an ‘app/global.css’ file and we can also install and import external stylesheets in the same way. And that’s it, styling Next.js is that easy!
Reference
https://nextjs.org/docs/app/building-your-application/styling/css-modules