Unlike MySQL, where a query can perform various operations like creating tables, inserting records, deleting records, etc, in MongoDB we have separate functions for these such as createCollection(), insertOne(), insertMany(), deleteOne(), and deleteMany(). Queries in MongoDB do not handle such tasks. So what does it do?
Queries in MongoDB are used to filter the result return when selecting the documents from a collection. A query object that contains the query is passed as an argument to the methods find() and findOne() to filter the selection.
For example, if we use the find() method for a student collection, it will select all of them but if we pass a query object { age: “18” } then the find() method returns only those documents when the age field is exactly equal to “18”.
Node.js MongoDB Filter Query
In Node.js and MongoDB, we can pass a filter query object as a first argument to find methods to filter the result.
The query object contains criteria in the form of key-value pairs where the key is the field name and the value is the condition for that field.
Syntax:
database.collection(collectionName).findOne(query, function(err, document) {
if (err) throw err;
console.log(document);
});
Here:
- database: MongoDB database connection object.
- collectionName: Name of the collection for querying documents.
- query: Query object with criteria for finding the document.
- function(err, document): Callback function with “err” that captures any error and “document” that contains the selected document.
Example:
For example, we have some documents in a collection:
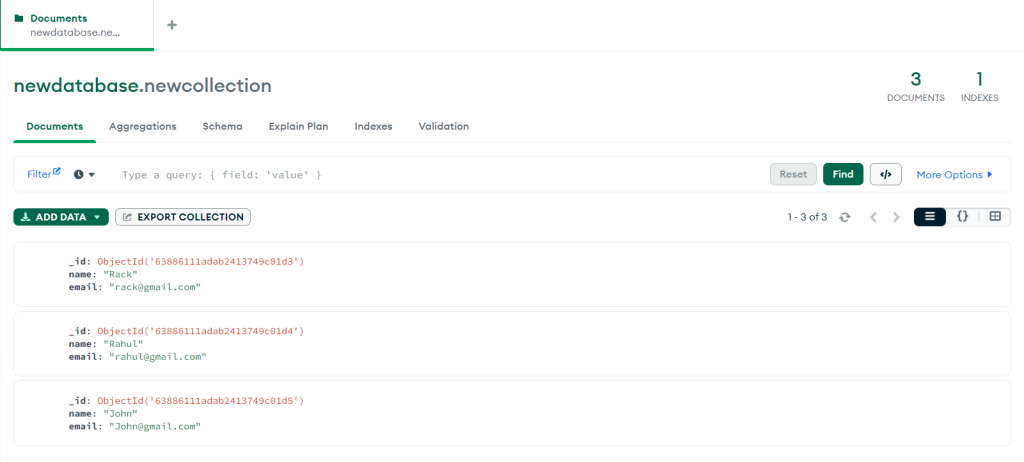
Query to filter the selection:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const query = { name: "Rack" };
database.collection("newcollection").find(query).toArray(function(err, result) {
if (err) throw err;
console.log(result);
});
});
Here the query object { name: “Rack” } is filtering the selection and returns only those documents where the name field is equal to “Rack”.
Output:
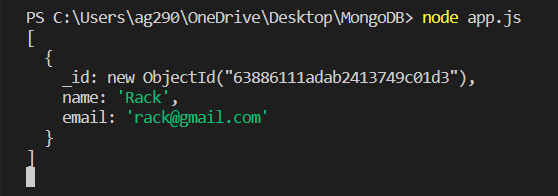
The collection has multiple documents, so the find() method selects all of them but the query filters them to only get the one where the name field is equal to “Rack”.
Node.js MongoDB Filter With Regular Expression
Regular Expression can be used to filter the selection on the basis of some symbols, characters, or groups of characters.
Example:
Below is an example of filtering the documents where the name starts with the letter “R”.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const query = { name: /^R/ };
database.collection("newcollection").find(query).toArray(function(err, result) {
if (err) throw err;
console.log(result);
});
});
Output:
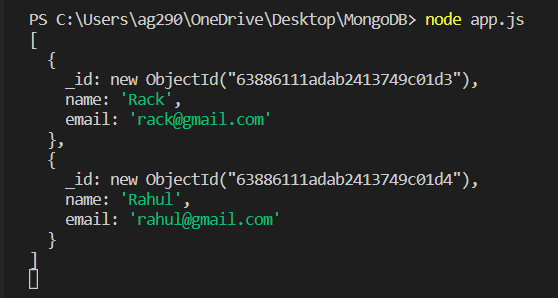
Here we only get those documents where the name field starts with the letter “R”.
Sorting and Projection
That’s not it, we have two cool options that we can use with our query to get the result in the way we want, which are sort and projection. Sort that sorts the result and projection that explicitly excludes or includes fields.
Sorting Documents:
We can sort the documents that the find() method returns in ascending or descending order using the sort option.
We can pass field name to sort by and the order number that is 1 for ascending and -1 for descending.
const options = {
// Sort matched documents by name in ascending order
sort: { name: 1 },
};
It is then passed with the query in the find() method like find(query, options).
Projection of Fields:
By default, MongoDB returns all fields of those documents that match the query passed. So we can use the projection option to filter these out. It excludes unnecessary fields from returned documents and explicitly includes only required ones.
For including a field, we have to pass the field name with 1 and to exclude pass the field name with 0.
const options = {
// Explicitly includes name and age fields, explicitly excludes the id field from returned documents
projection: { _id: 0, name: 1, age: 1 },
};
In the same way, we can pass this along with the query in the find() method to apply.
Summary
Query in MongoDB is used to filter the selected documents. When a query is passed inside a JavaScript object it is called a query object. This query object is passed as an argument to the method find() and findOne() to find the documents from a collection and then filter them according to the condition of the query. We can also use sort and projection options to enhance the search to the next level and that’s it, querying in MongoDB is that easy!
Want to learn about the findById() function? Click here to read our dedicated article on it.
Reference
https://www.mongodb.com/docs/drivers/node/current/usage-examples/find