A process is a global object which can be used directly inside a Node.js application without importing them.
The majority of Node.js objects and method is needed to import before using them inside an application just like a file system, for using any of its methods it is required to import them but the global object can be used directly. There are different types of global objects which perform different operations.
In Node.js, there are hundreds of processes running simultaneously and it is important to control them, the global process object gives the power to do so. The process object contains many properties and methods which we can use for different operations.
A process object is used to get details about the different processes running, used to manipulate them, stop the processes, etc. Let’s see them one by one.
1. process.version
This property gives the details we get when executing the node –version command.
Example:
let result = process.version;
console.log(result);
Here we are passing the value get from the process.version to a variable and then logging it in the terminal using the console global object.
Output:
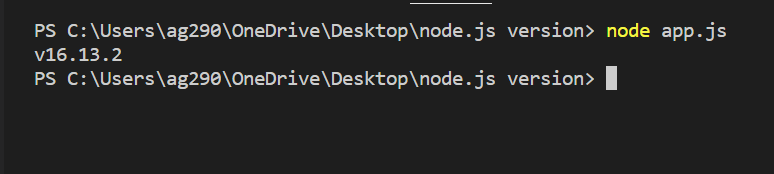
2. process.release
This property gives the details regarding the Node.js version installed on the system.
Example:
let result = process.release;
console.log(result);
Output:
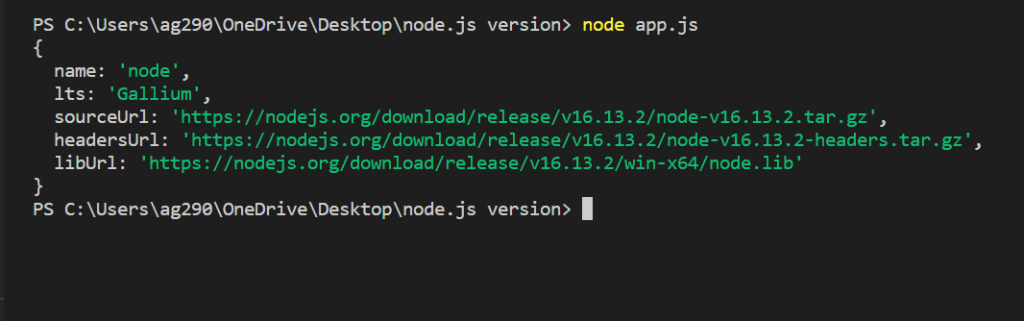
3. process.argv
This property is used to get an array of the arguments typed in the terminal.
By default the first argument is the path of the node itself, the second is the path of a file that is executed using the node and after that, the arguments passed in the terminal.
Example 1:
let arguments = process.argv;
for (argument of arguments) {
console.log(argument);
}
Here we are using the process.argv property to get the argument passed in the terminal then use the for-of loop to loop through it, and print each argument one by one using the console global object.
Learn about the for-of loop in detail – https://codeforgeek.com/nodejs-loops/
Output:

Example 2:
Let’s create a calculation that adds the numbers passed in the terminal. Use the process.argv property to get the argument passed in the terminal, and use the third and fourth arguments since the first two are the node itself and the file which we execute. Before adding them convert them into Integers by default Node treats the arguments as a string.
let arguments = process.argv;
const sum = parseInt(arguments[2]) + parseInt(arguments[3]);
console.log(sum);
Output:
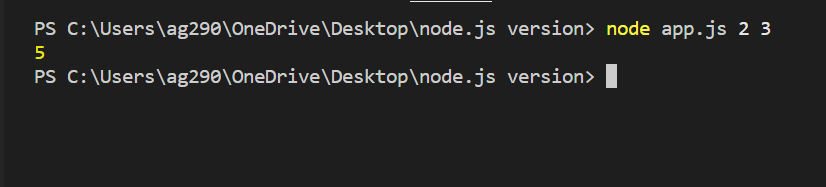
4. process.exit
This method uses to stop the currently running process.
Example:
const hello = false;
if(hello){
console.log('Hello World!');
}else {
process.exit();
}
console.log("I will not execute.");
Here we are defining that if the value of a variable is true then a string is printed in the console otherwise the process gets exited and the code after that doesn’t get executed.
Output:
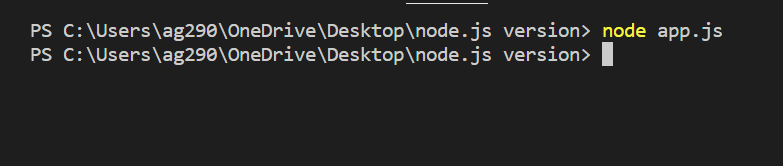
5. process.stdin
This method creates a readable stream which is used to get user inputs from the terminal.
Example:
process.stdin.on('data', data => {
console.log(`You typed ${data.toString()}`);
process.exit();
});
Here we will use the on method which triggers when the user types any value in the terminal and then the on method will log that value in the terminal by changing it into a readable string.
Output:
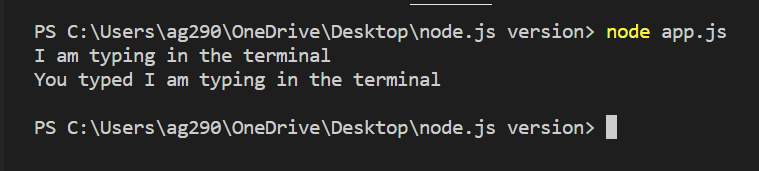
6. process.stdout
This method creates a writeable stream which is used to write values in the terminal.
Example:
process.stdout.write("Hello World!");
Here we will write a string in the terminal.
Output:

7. process.stderr
This method returns a stream connected to stderr.
Example:
console.log(process.stderr.rows);
Output:

Summary
A process is a global object used for different operations regarding the currently running process in Node.js. It is also used to interact with the terminal, output a value, takes input from the users, fetch arguments passes in the terminal, and many more. Hope this article helps you to learn Node.js global process objects.
Reference
https://nodejs.org/api/process.html