ZLIB is a Node.js module that is used to zip and unzip files. It is not required to install this module to use it inside the application, it is a pre-build module so it can be used directly by requiring it inside the application.
Syntax:
const zlib = require('zlib');
Compressing or Zipping a File Using NodeJS Zlib
Compression reduces file sizes and can be done in multiple file formats. Here, let’s try to compress a file as a zip.
The compression can be done by using the createGzip() method of the ZLIB module.
Syntax:
zlib.createGzip()
Let’s create a simple file compression application in Node.js using the ZLIB module.
Implementing File Compression Using NodeJS Zlib
Let’s get right into an example of how you can compress files using NodeJS.
Setting up the environment
- Folder Structure
Create a project folder, “File Compressor”, inside this folder create a file app.js where we will write code and, unzip.text contains a “Hello World!” text.
- Code Editor
Open the project inside the code editor, for this article VS-Code is used, but you can use any editor like the atom, subline, etc.
- Check Node.js
Open the terminal and type the following command to make sure that Node.js is installed on your system.
node -v
If it returns a version then Node.js is installed, if not then install it from Node.js’ official website.
Importing the required modules
To use the ZLIB module, we first have to require it. We will also use the File System module since we have to interact with files.
const zlib = require('zlib');
const fs = require('fs');
Create readable and writable streams, the readable stream is used for reading the file so that we can convert it into a zip file whereas the writable stream is used to create a new file as an output for the converted zip file.
const inputFile = fs.createReadStream('unzip.txt');
const outputFile = fs.createWriteStream('zip.gz');
Then we have to pipe the input stream to read the file and then pipe the output stream to store the converted file.
inputFile.pipe(zlib.createGzip()).pipe(outputFile);
Complete Code to Compress Files Using NodeJS
const zlib = require('zlib');
const fs = require('fs');
const inputFile = fs.createReadStream('unzip.txt');
const outputFile = fs.createWriteStream('zip.gz');
inputFile.pipe(zlib.createGzip()).pipe(outputFile);
Output
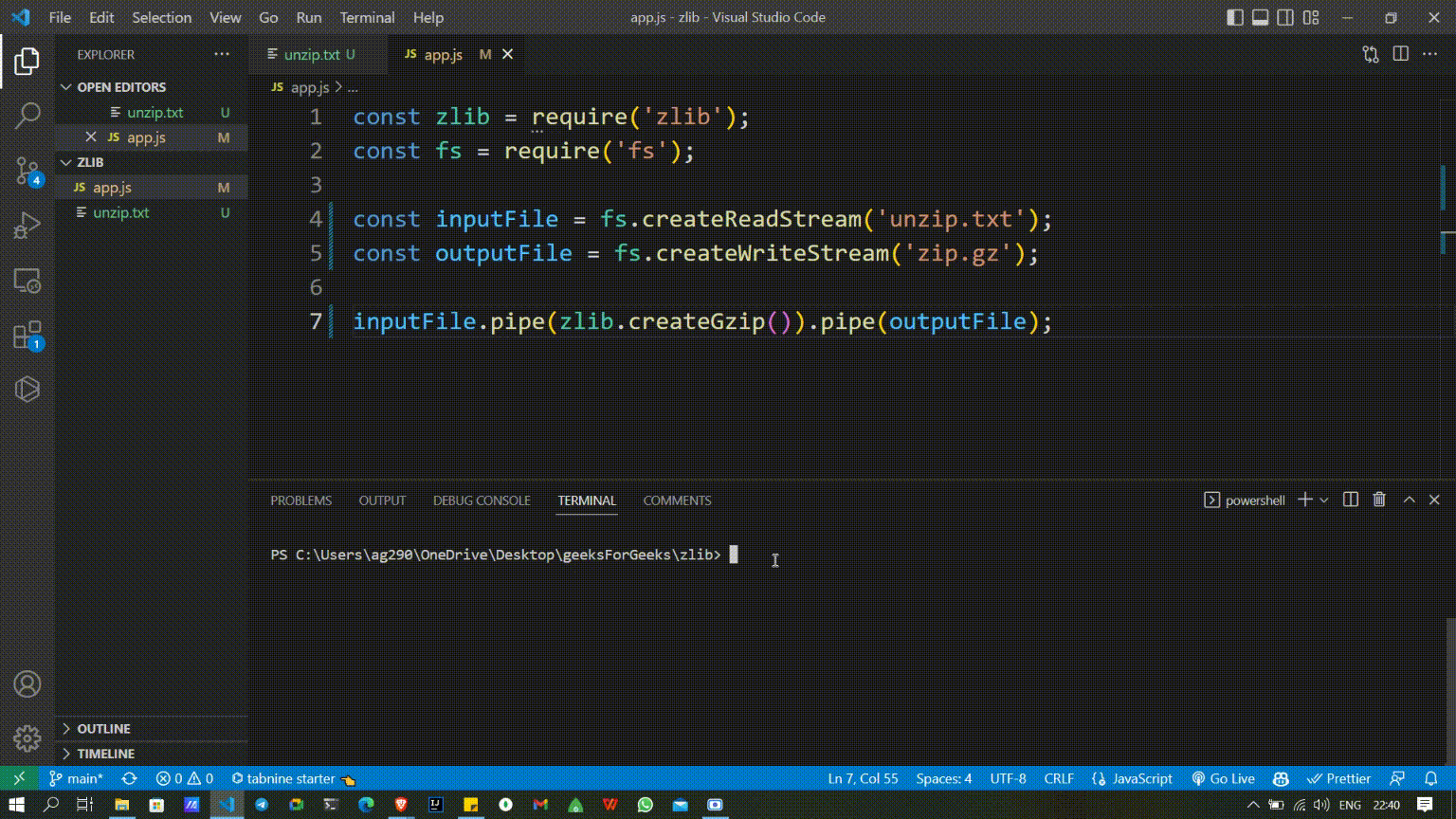
Decompressing a File Using NodeJS Zlib
Decompression is used to decompress/unzip a file and convert it into its original form.
The compression can be done by using the createUnzip() method of the ZLIB module.
Syntax:
zlib.createUnzip()
Let’s create a simple file decompression application in Node.js using the ZLIB module.
Creating a NodeJS App to Decompress Files
The setup and requiring statements are the same as the previous application
const zlib = require('zlib');
const fs = require('fs');
Create readable and writable streams, the readable stream is used for reading the file so that we want to decompress/unzip whereas the writable stream is used to create a new file as an output for the converted file.
const inputFile = fs.createReadStream('zip.gz');
const outputFile = fs.createWriteStream('unzip.txt');
Then we have to pipe the input stream to read the zip file and then pipe the output stream to store the converted unzip file.
inputFile.pipe(zlib.createUnzip()).pipe(outputFile);
Complete Code for Decompressing Files Using Zlib
const zlib = require('zlib');
const fs = require('fs');
const inputFile = fs.createReadStream('zip.gz');
const outputFile = fs.createWriteStream('unzip.txt');
inputFile.pipe(zlib.createUnzip()).pipe(outputFile);
Output
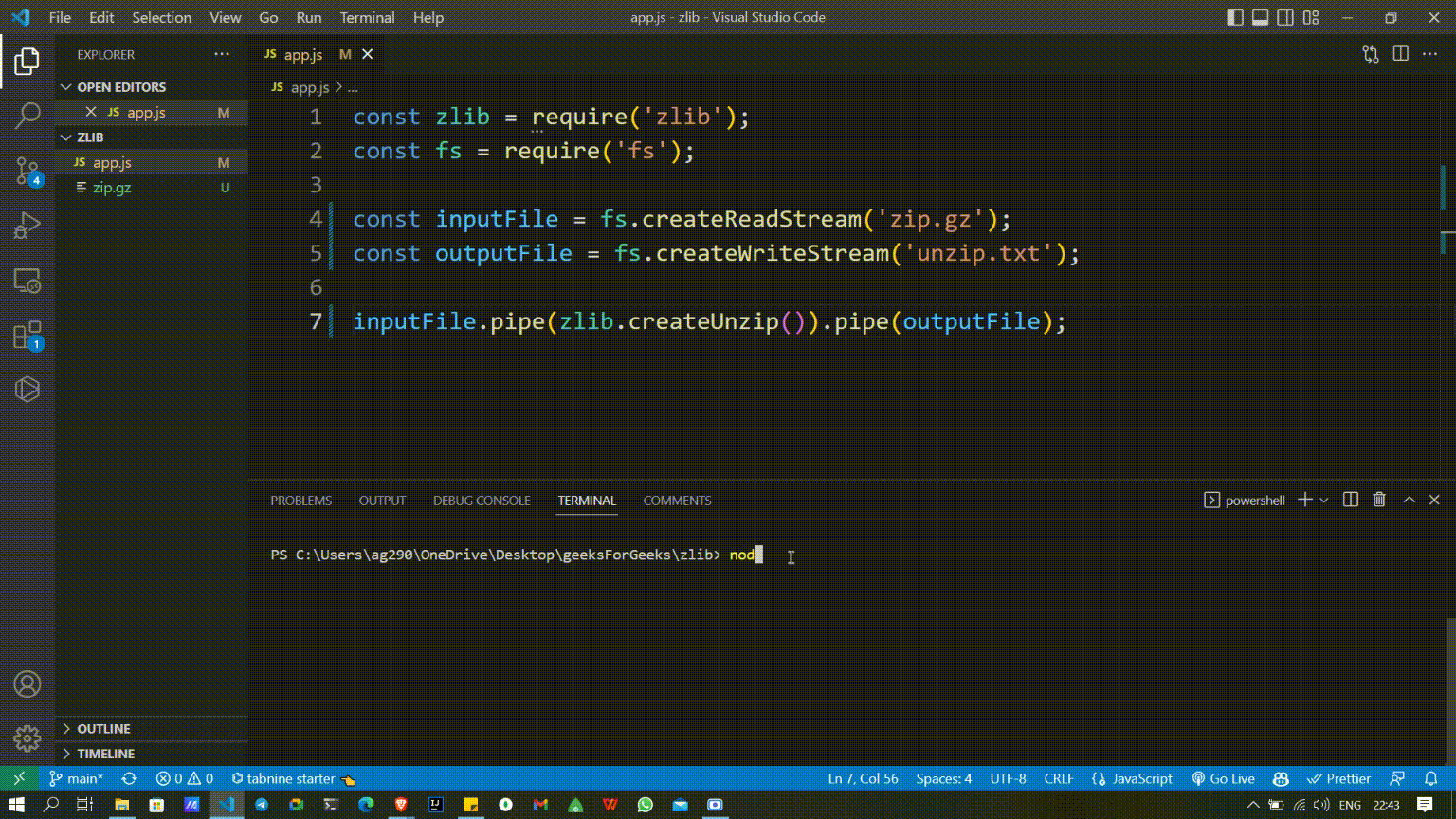
Frequently Asked Questions (FAQs)
How to zip a file using Node.js?
To zip files in Node.js, first import the ‘zlib’ module, then create a readable stream from the file you want to zip and a writable stream using a filename and respective file path for the zip output and finally pipe the readable stream using createGzip() into the writable stream to zip the file.
How to unzip a file in Node.js?
To unzip a file in Node.js, first import the ‘zlib’ module, then create a readable stream from the file you want to unzip and a writable stream using a filename and respective file path for the unzip output and finally pipe the readable stream using createUnzip() into the writable stream to extract the file.
What is the unzip command?
The command for unzipping a file in Node.js is inputFile.pipe(zlib.createUnzip()).pipe(outputFile) where zlib.createUnzip() is a method of the zlib module.
How do I unzip freely?
You can unzip a file freely by using software like 7-Zip, PeaZip, Unzipper, and Unarchiver. If you don’t want to download software, you can use websites like ezyZip, Zippyshare, Zamzar, etc.
Why can’t I unzip a file?
There are many reasons why you cannot unzip a file, such as a file you want to unzip is corrupt, or maybe password projected, or it is too large and you lack storage, or the file format is not supported by your unzipping software.
How to unzip a file using the command line?
You can use the command line to unzip a file directly without using any tools. You just have to run the command unzip [options] file.zip, where options can be used to define the output directory.
Summary
Sometimes we want to create a zip file and sometimes we want to unzip a file. To compress and uncompress files, we have to use a third-party application. But by using the ZLIB, you can create a zip file and also unzip it by writing a few lines of code.
ZLIB is pre-installed in just Node.js so you don’t even have to install it. Just import it inside a javascript file and use its functions to perform different operations. ZLIB provides us with many methods but the widely used method is the createGzip() and createUnzip() method which is used to zip and unzip a file.
The ZLIB module uses various algorithms for this, including the gzip algorithm. If you want to try some other Node.js module to zip and extract zip files, you can use the JSZip module. You can also use the adm-zip module which can be installed using a package manager like NPM. The adm-zip module has methods like addLocalFile, and ExtractAllTo to handle zip files in an asynchronous way. We hope this tutorial helped you understand the ZLIB module in Node.js.
Also Read: Buffers in Node.js – A 3-Minute Guide To Get You Started
Reference
https://nodejs.org/api/zlib.html