Suppose you are creating a Next.js application and want the same components like header and footer on all your pages then you can add layout to make this happen. Let’s understand what it is and how to add it to a Next.js application in detail.
Prerequisites
- Next.js Introduction
- Next.js Installation
- Next.js Folder Structure
- Get Started with Next.js
- File-Based Routing in Next.js
Understanding Pages and Layouts
In a Next.js application in the App Router system, a route is created by creating a folder inside the app directory and each folder inside it can have its own layout and page file.
The layout is like a wrapper that goes with each page within their respective folder or subfolder to construct a similar component for all of them. It is used to set the initial state of the application as it is loaded on every page.
So let’s say you want the same navbar on all your application pages, you can store them in app/layout.tsx, and it gets imported into the page of that folder (app/page.tsx) or subfolders (app/../page.tsx) to have the same navbar.
Creating a Layout Component in Next.js
Let’s see this in action and add a layout to a next.js application.
Setup:
Run the below command in the terminal to create a new Next.js application:
npx create-next-app@latest
Check Yes for both TypeScript and App Router to follow along with us:
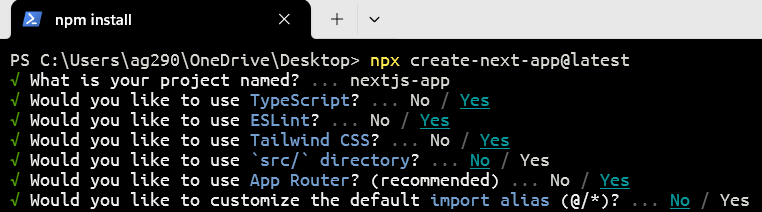
Replacing the Default Next.js Home Page:
Now open the project inside a code editor and remove all the files inside the app directory.
Then only create a file page.tsx for our home page:
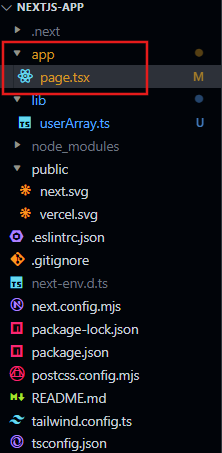
Write the following code inside the app/page.tsx:
const HomePage = () => {
return <h1>Home Page</h1>;
};
export default HomePage;
Creating a New Page:
Now to create a new page like “/new”, create a new folder named new inside the app directory with a page.tsx file:
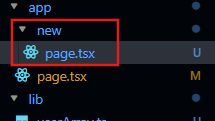
Write the following code inside the app/new/page.tsx:
const NewPage = () => {
return <h1>New Page</h1>;
};
export default NewPage;
Testing the Application:
To run the project, move on to the project directory and execute the below command:
npm run dev
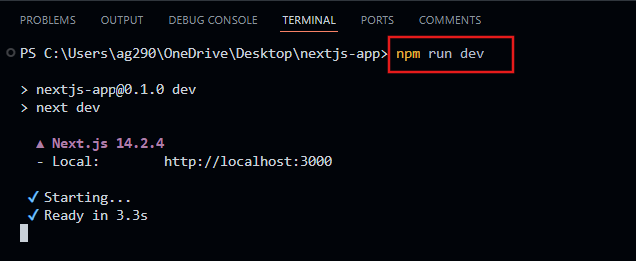
Now open the browser and paste http://localhost:3000 to see our home page:
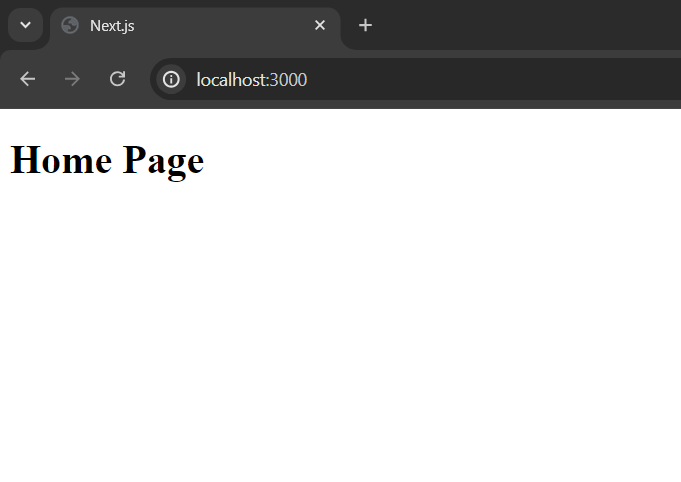
And navigate to http://localhost:3000/new to open our newly created page:
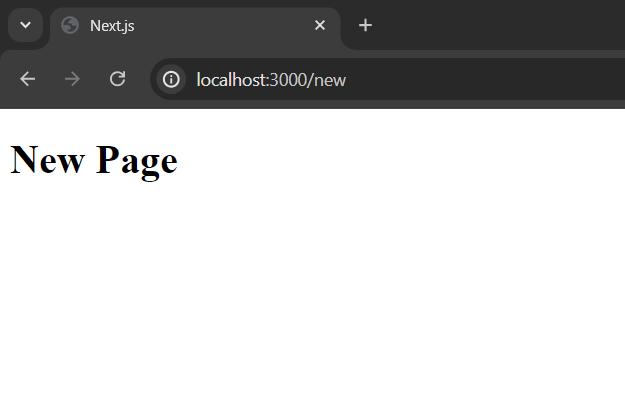
Creating Layout Component:
Now you can see a new layout.tsx file is already created inside the app directory by Next.js:
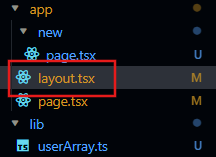
Just remove all the boilerplate code inside it and write the below code:
import Link from 'next/link';
export const metadata = {
title: 'Next.js',
description: 'Generated by Next.js',
}
export default function RootLayout({ children }: { children: React.ReactNode }) {
return (
<html lang="en">
<body>
<nav>
<ul>
<li><Link href="/">Home Page</Link></li>
<li><Link href="/about">New Page</Link></li>
</ul>
</nav>
{children}
</body>
</html>
);
}
Here the first line imports the Link component from “next/link”. Then there is a metadata object that defines the meta title and description. After this, the code exports a component called RootLayout as the default export. This layout component takes a prop called children of React.ReactNode type, holding the contents of each page to be wrapped inside the layout. All the code inside this is just basic HTML containing a navbar and children.
Output:
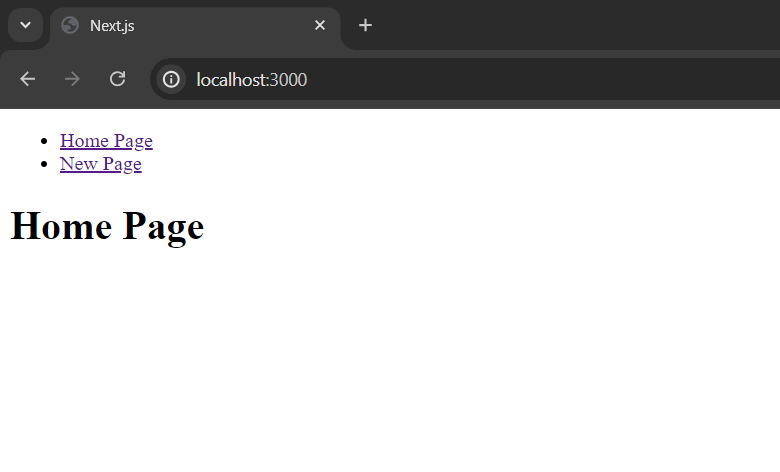
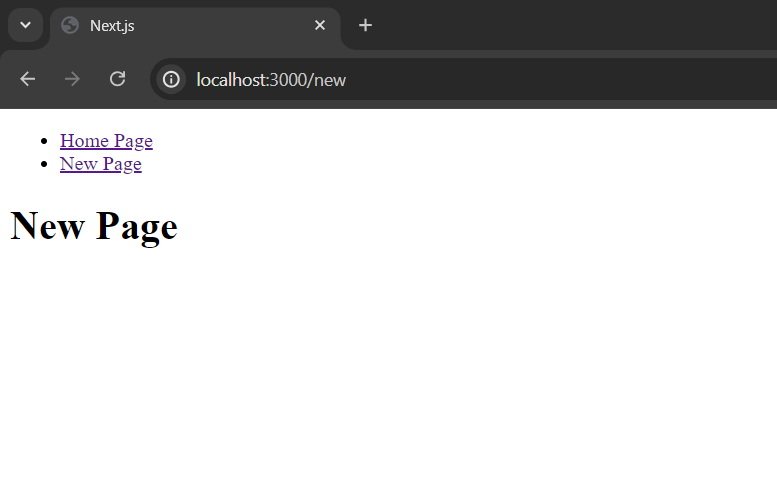
Now if you create any other page you will see the same navbar. Also if you search for a page that doesn’t exist then Next.js shows the default 404 page and it will also contain your layout component:
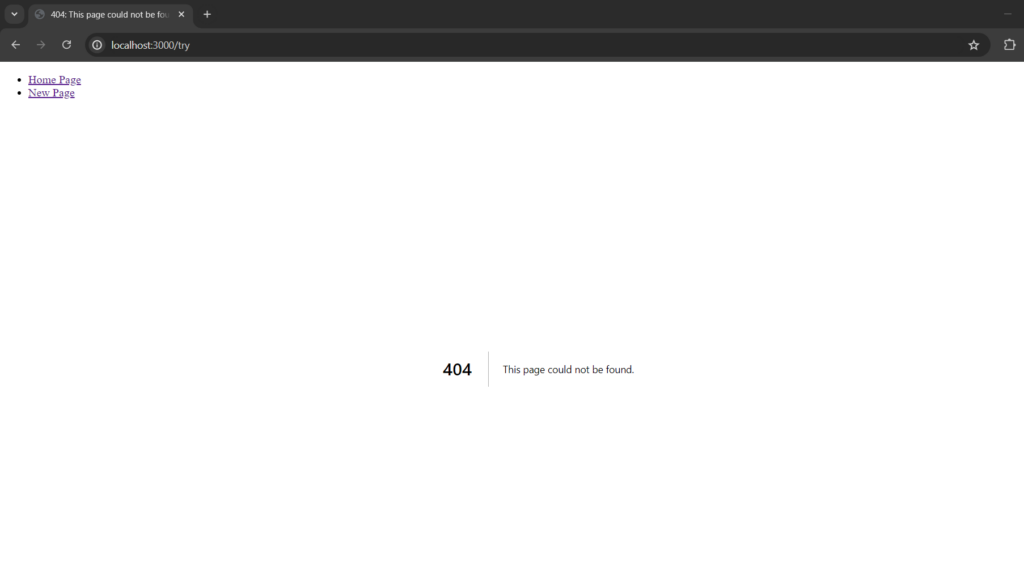
Summary
In short, you can add a layout to a Next.js application by creating a file named layout.tsx (for TypeScript) or layout.js (for JavaScript) inside the app directory. This layout applies to every page.tsx or page.js inside that app directory or subdirectories.
So suppose you want the same component on all your application pages, you can store them in layout.tsx, and it gets automatically imported inside page.tsx of that folder or subfolder to have the same design in all your pages.
Reference
https://nextjs.org/docs/app/building-your-application/routing/pages-and-layouts