Finding the product of array elements in Python is really important for math stuff, programming, looking at data, and doing science things. Our goal in this article is to achieve that. Here, we will discuss some easy ways to calculate the product. We will explore the predefined function in NumPy that helps us to do so, along with parameter details and examples.
Methods to Find Product of Array Elements
To find the product of array elements, you multiply all the numbers in the array together. This task is necessary for programming because it helps with calculating factorial, cumulative product, or doing matrix operations. Let’s explore the various methods to find a product of array elements in Python.
Method 1: By Using Loop
First, we will see how we can use basic logic using a loop and implement the idea to obtain the product of the array elements.
def product(arr):
p = 1
for num in arr:
p *= num
return p
array = [2, 4, 8, 10]
result = product(array)
print("Product of array elements:", result)
In this example, we’re making a function called “product” to find the total when you multiply all the numbers in a list. We start with 1, then go through each number in the list, multiplying them together. Afterwards, we get the result. Then, we use this function with a list of numbers and print out what we get.
Output:

Method 2: By Using NumPy prod() Function
Python’s NumPy library has functions for every calculation to make things easy. It includes the prod() function, which multiplies all elements of an array together.
The syntax of this function is:
numpy.prod(array, axis=None, dtype=None, keepdims=<no value>, initial=<no value>, where=<no value>)
Let’s understand the meaning of all parameters and use them in the given examples.
Example 1:
In this basic example, we simply use the prod() function on the given array.
import numpy as np
array = [1, 2, 3, 4, 5]
result = np.prod(array)
print("Product of array elements:", result)
Output:
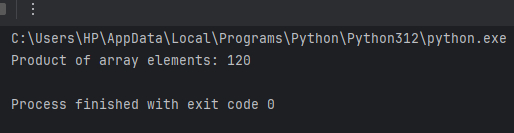
Example 2:
Here, we will specify the data type(dtype) parameter. It will calculate the product of all elements in the array with the specified data type.
import numpy as np
a = np.array([1, 3, 6])
result = np.prod(a, dtype=np.float64)
print("Product with float64 data type:", result)
Output:

Example 3:
Now let’s see how we can keep dimensions along with the results of the product. When keepdims is True, the output keeps the same dimensions as the original array, even if some of them have only one element. So, the output array maintains the shape of the input array.
import numpy as np
a = np.array([[1, 2], [3, 4]])
result = np.prod(a, keepdims=True)
print("Product with dimensions kept:", result)
Output:
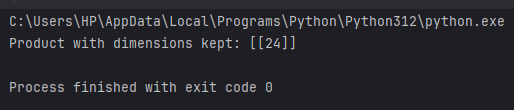
Example 4:
Let’s try specifying the axis for the multiplication. It will calculate the product along different axes.
import numpy as np
a = np.array([[5, 5], [2, 2]])
r0 = np.prod(a, axis=0)
print("Product of elements along axis 0:", r0)
r1 = np.prod(a, axis=1)
print("Product of elements along axis 1:", r1)
When we calculate the product of elements along axis 0 (columns) of the array, it multiplies the elements along each column separately. So, r0 would be [10, 10].
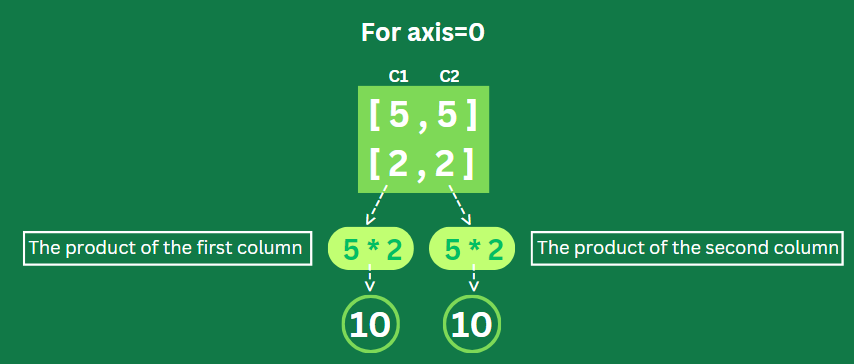
When we calculate the product of elements along axis 1 (rows) of the array, it multiplies the elements along each row separately.
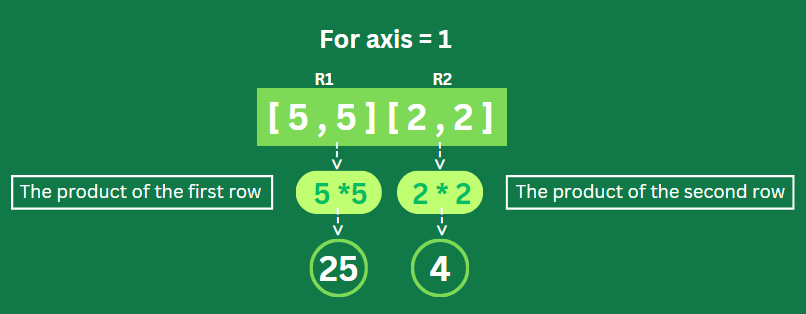
Output:
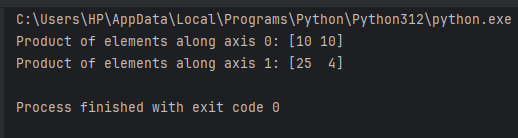
Method 3: By Using NumPy nanprod() Function
The nanprod() function computes the product of array elements while ignoring any NaN (Not a Number) values. It’s useful when we have datasets with missing or invalid values, ensuring correct calculations. The function works similarly to prod(), but skips over NaN values during computation.
Example:
Let’s take an example to illustrate the use of the np.nanprod() function with all parameters.
import numpy as np
a = np.array([[3, np.nan], [9, 9]])
result = np.nanprod(a, axis=0, dtype=np.float64, out=None, keepdims=True)
print("Product on ignoring NaN values:", result)
The product of the array is computed along axis 0, ignoring NaN values.
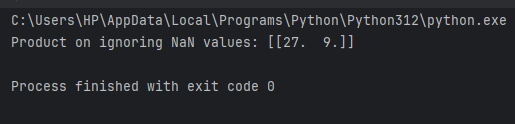
Conclusion
And we’ve reached the end. In this blog, we discussed the most commonly used and easiest ways to calculate the product of arrays. We’ve seen how to use different parameters, such as providing the calculation axis and specifying the data type. I hope this clarifies the concept for you. Now, explore how to calculate differences and find the cumulative sum of data in Python.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.prod.html