JSON, which stands for JavaScript Object Notation is a widely used format for storing data in the form of key-value pairs, similar to an object in JavaScript. It is language-independent making it easier to exchange data throughout various programming languages. In this article, we’ll look at working with JSON files in Python, with elaborate steps and examples on how we can read and modify data.
Reading and Modifying JSON Files in Python
Python provides us with simple methods to work with JSON files, including the built-in json library. Utilizing this library and various functions in it, we can efficiently read and modify JSON files.
Before we can start working with JSON files, we must first import the json library using the command:
import json
Now that we have imported json, we must have a JSON file containing some data that we want to read or modify.
Here, our JSON file is called file.json and contains the following data:
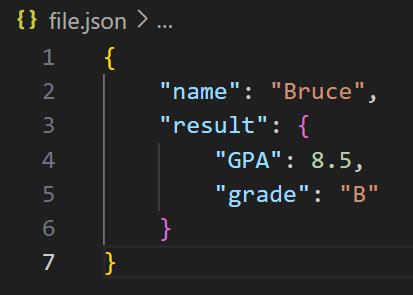
Now we can now work with JSON file.
Reading a JSON File
Before we can manipulate data present in the JSON file, we must first read the file. To read JSON data in Python, the json library provides two methods: json.load() and json.loads().
- json.load() : used to read data from a json file and return it as a Python dictionary.
- json.loads() : used to read data in string format and return it as a Python dictionary.
In this case, we’ll use json.load() as we are reading data from a JSON file and displaying it. Before we can read from the file, we open the file in read mode using open().
Example:
import json
def read_json(file_path):
file = open(file_path, 'r')
data = json.load(file)
return data
file_path = 'file.json'
json_data = read_json(file_path)
print(json_data)
Here, we have a function read_json() that takes in the file path as the argument. We then open our JSON file in read mode (‘r’) and store the data from the file in a variable called file. This data can now be loaded using json.load() and returned by the read_json() function. The data is returned as a Python dictionary and stored in json_data.
Finally, we can print json_data to give the following output.
Output:

Modifying a JSON File
Modifying data in a JSON file includes performing many operations on it such as adding data, removing data, etc.
Some of the operations we’ll discuss are as follows:
- Adding data to a JSON file
- Updating data in a JSON file
- Removing data from a JSON file
In order to modify data, we must first read the JSON file and write it into the JSON file after the modifications are done. Let us discuss each of these operations in detail.
1. Adding Data to a JSON File
Before we can add data to the file, we first load the data using json.load(). We will look at an example of adding a new field rollno to our JSON file which contains the role number.
Example:
import json
file = open('file.json', 'r')
data = json.load(file)
data['rollno'] = 21
file = open('file.json', 'w')
json.dump(data, file)
Here, after loading the JSON file file.json, we add a new field to data called rollno. Now we have to write the updated data into file.json, we can do this using the json.dump() method. This method is used to store data in a JSON file.
Output:
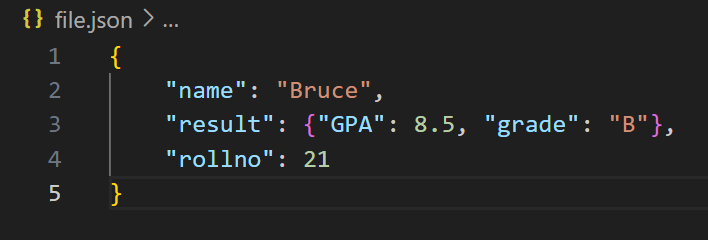
2. Updating Data in a JSON File
We can update data in a JSON file by referencing its key value. Here we’ll update the rollno we’ve added in the previous example and write the updated value to our JSON file.
Example:
import json
file = open('file.json', 'r')
data = json.load(file)
data['rollno'] = 31
file = open('file.json', 'w')
json.dump(data, file)
Here, we have read the data from file.json and updated the rollno field which was previously 21, to 31. To reflect the changes made, we must write to the JSON file using json.dump().
Output:
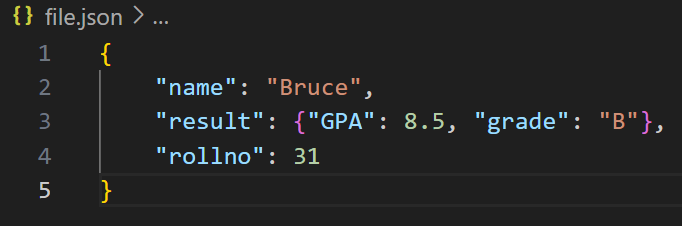
3. Removing Data from a JSON File
We can remove data from a JSON file by referencing the key. We will look at an example where we’ll remove the rollno field from file.json using del. The del keyword is used to delete objects in Python.
Example:
import json
file = open('file.json', 'r')
data = json.load(file)
if 'rollno' in data:
del data['rollno']
file = open('file.json', 'w')
json.dump(data, file)
Here, we remove the rollno field from file.json if a field of that name exists in the file. We delete the field using the del keyword. To reflect these changes we write into the file using json.dump().
Output:
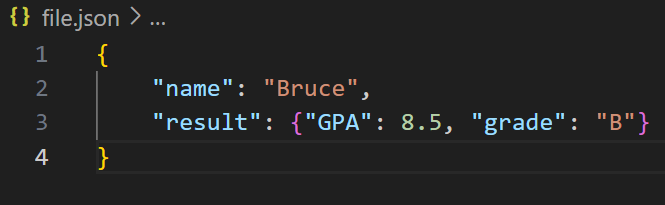
Conclusion
While working with JSON files there will often be instances where we’ll have to modify and manipulate data in the file. In order to modify data we have seen that the file must first be read and loaded using json.load(). For the changes to be reflected in the JSON file we must write back to the JSON file using json.dump(). By knowing how to perform these operations we can work with JSON files more efficiently.
Reference
https://stackoverflow.com/questions/21035762/python-read-json-file-and-modify