A set in JavaScript is a group of unique values. Each value can occur only once in a set. It cannot have duplicate or repeated values. The values can be of any type, such as primitive values or objects. A set is a great way of storing distinct elements and is implemented as an object in JavaScript.
Sets in JavaScript
Let’s learn the implementation of sets in JavaScript. In this section, we will cover, how to create sets, get the size of sets and list the elements within a set.
How to Create a Set?
For creating a set, we use the Set constructor in this way:
const setA = new Set();
Here setA is the name of your set, and this behaves as an object.
A set can be empty or contain values such as strings, characters, and integers. But it considers only non-repeated values. Consider the following example:
let setA = new Set();
let setB = new Set(["apple","mango","grapes"]);
let setC = new Set("element");
let setD = new Set([5,10,10,15,15]);
console.log(setA);
console.log(setB);
console.log(setC);
console.log(setD);
In this case,
- The setA is an empty set.
- The setB contains three string elements.
- The setC will consider only ‘e’, ‘l’, ‘m, ‘n’, and ‘t’. The ‘e’ here is repeated three times but considered only once.
- Similarly, setD will only contain 5, 10, and 15.
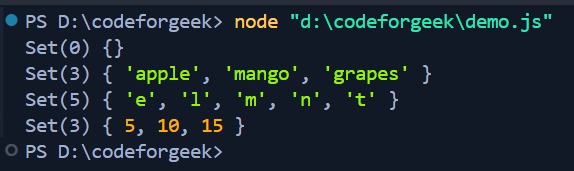
How to Get the Size of a Set?
The number of considered elements in a set is the size of the set. There are two ways in which you can determine the set size:
Method 1: In the console display of the set, look for the integer value inside the brackets. It represents the size of the given set.
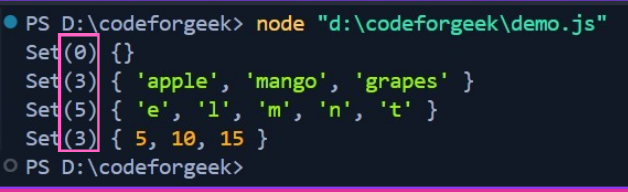
Method 2: To explicitly know the set size, we can use the .size property of a set. It will display the integer value of the set size like this:
let fruits = new Set(["apple","mango","grapes","watermelon"]);
console.log(fruits.size);

How to List the Elements of a Set?
The elements in a set iterate according to the insertion order. We can list all set elements with the help of a loop like this:
let fruits = new Set(["apple", "mango", "grapes", "watermelon"]);
for (let fruit of fruits) {
console.log(fruit);
}
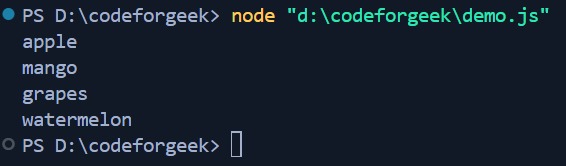
Methods of Set in JavaScript
There are some predefined methods provided by JavaScript to operate on sets, like adding or deleting elements, and many more. Let us go through all of these methods.
1. add() Method
Purpose: It adds a new element with a specific value to the set.
Syntax:
setA.add(value);
Parameter: Here, the value can be any specific value, like an integer, string, or character.
Return: It returns the set containing the added value.
Example:
let setA = new Set(["Blue","Red","Green"]);
console.log("Before Addition :");
console.log(setA);
setA.add("Pink");
setA.add("White");
console.log("After Addition :");
console.log(setA);
Output: New elements get added at the end.
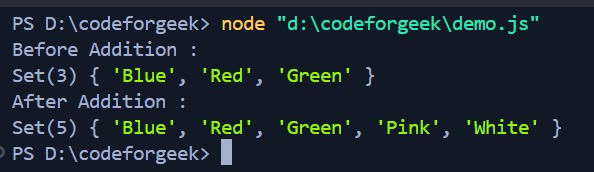
2. delete() Method
Purpose: It deletes the specified element from the set.
Syntax:
setA.delete(value);
Parameter: Here, the value can be any value present in the set.
Return: It returns the set after removing the specified value.
Example:
let setA = new Set(["Blue","Red","Green"]);
console.log("Before Deletion :");
console.log(setA);
setA.delete("Red");
setA.delete("Green");
console.log("After Deletion :");
console.log(setA);
Output: Specified elements get removed from the set.
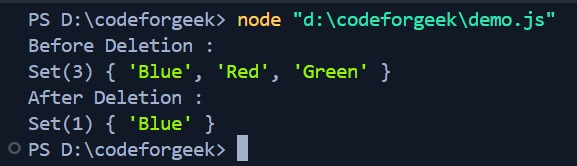
3. clear() Method
Purpose: It removes all the elements from the set and makes it empty.
Syntax:
setA.clear();
Return: It returns an empty set.
Example:
let setA = new Set(["Blue","Red","Green"]);
console.log("Before Clearing :");
console.log(setA);
setA.clear();
console.log("After Clearing :");
console.log(setA);
Output: An empty set is displayed.
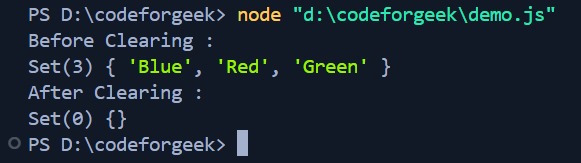
4. entries() Method
Purpose: This method gives you a way to go through each value in the set, returning each value as a pair like [value, value]. But why a pair? This is because the entries() method also works for data structures like maps, which use key-value pairs. Since a set doesn’t have keys, it just shows the value twice.
Syntax:
setA.entries();
Return: It returns an object of the Set iterator that contains an array of [value, value] for each element.
Example:
const mySet = new Set(["apple", "banana", "cherry"]);
const entries = mySet.entries();
for (let entry of entries) {
console.log(entry);
}
Output: The value pairs are displayed on the console.
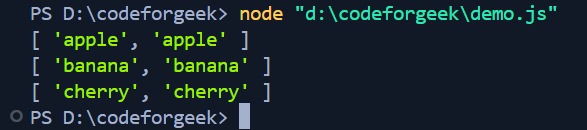
5. has() Method
Purpose: It checks whether the set contains a specific value.
Syntax:
setA.has(value);
Parameter: Here, the value refers to the element whose presence we want to check in the set.
Return: If the specified value is present in the set, this method returns true, if not, then false.
Example:
let setA = new Set(["Blue","Red","Green"]);
console.log("Red present in the set :"+ setA.has("Red"));
console.log("Black present in the set :"+ setA.has("Black"));
Output: Red is present in the setA, hence it returns true. Black is absent from the set, hence, it returns false.
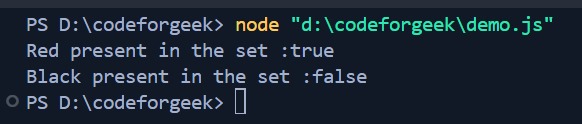
6. values() Method
Purpose: This method gets all the values inside the set and displays them according to their insertion order.
Syntax:
setA.values();
Return: This method returns all the values present inside the set.
Example:
let setA = new Set(["Blue","Red","Green"]);
console.log(setA.values());
let setB = new Set();
setB.add(5);
setB.add(10);
setB.add(15);
console.log(setB.values());
Output: The values inside sets A and B are displayed according to the insertion order, respectively.

7. keys() Method
Purpose: The keys() method works in the same manner as the values() method. It also fetches all the values in the set.
Syntax:
setA.keys();
Return: It returns all the values present inside the set.
Example:
let setA = new Set(["Blue","Red","Green"]);
console.log(setA.keys());
let setB = new Set();
setB.add(5);
setB.add(10);
setB.add(15);
console.log(setB.keys());
Output:

8. forEach() Method
Purpose: This method iterates over the elements of the set and executes the provided function on each element. It does not change the original set.
Syntax:
setA.forEach(callback)
Parameter: Here, callback is a function that will execute on each element of the set.
Return: This method executes a provided function once for each element in a set, but it does not return a new set or any other value.
Example:
let setA = new Set([5, 10, 15]);
console.log("Before division:");
console.log(setA);
function divideByFive(number) {
console.log(number / 5);
}
console.log("After division:");
setA.forEach(divideByFive);
Output: The forEach() method executes the divideByFive function on each element of the set A.
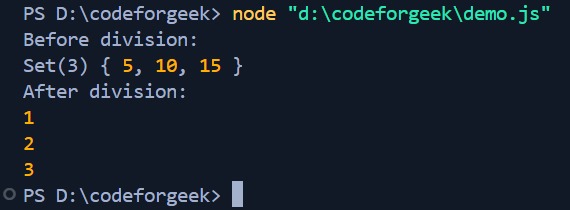
Set Operations in JavaScript
The set operations like subset, union, intersection, and difference are not predefined in JavaScript. We need to implement them manually using JavaScript’s set object. Let’s see how.
1. Subset
Description: If all elements of one set, say set A, are present in another set, say set B, then set A is a subset of set B.
Venn Diagram:
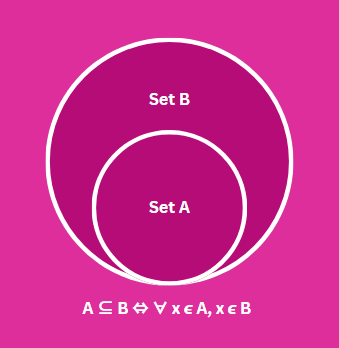
Mathematical Notation: A ⊆ B ⇔ ∀ x∊A, x∊B
Syntax:
function isSubset(setA, setB) {
for (let elem of setA) {
if (!setB.has(elem)) {
return false;
}
}
return true;
}
Parameters: We will input two sets as parameters to our function.
Return: Our function will return a boolean value. If the first set would be the subset of the second subset then it will return true else false.
Example:
function isSubset(setA, setB) {
for (let elem of setA) {
if (!setB.has(elem)) {
return false;
}
}
return true;
}
const A = new Set([2,4,6,8,10]);
const B = new Set([1,2,3,4,5,6,7,8,9,10]);
console.log("A is subset of B :"+isSubset(A,B));
console.log("B is subset of A :"+isSubset(B,A));
Output:
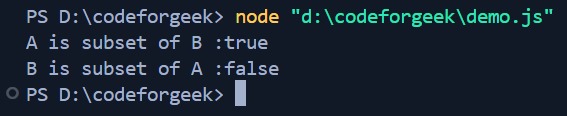
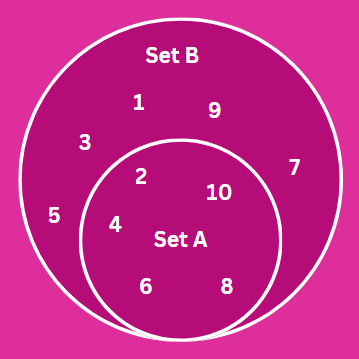
2. Union
Description: A union set of sets A and B is the combination of unique elements from both sets.
Venn Diagram:
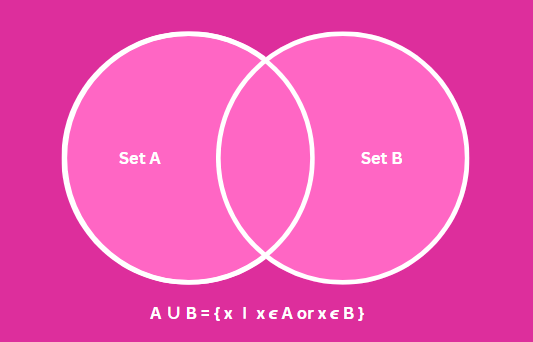
Mathematical Notation: A ∪ B = { x ∣ x∊A or x∊B }
Syntax:
function union(setA, setB) {
return [...new Set([...setA, ...setB])];
}
Parameters: We will input two sets as parameters to our function.
Return: Our function will return an array containing unique elements from both sets.
Example:
function union(setA, setB) {
return [...new Set([...setA, ...setB])];
}
const A = new Set([1, 2, 3, 4]);
const B = new Set([3, 4, 5, 6]);
console.log("The union set of A and B: " + union(A, B));
Output:

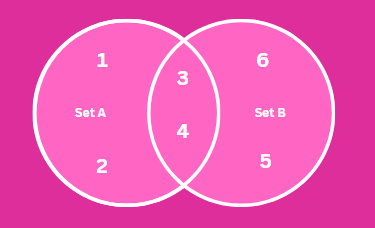
3. Intersection
Description: The intersection set of sets A and B contains the elements that are common to both sets.
Venn Diagram:
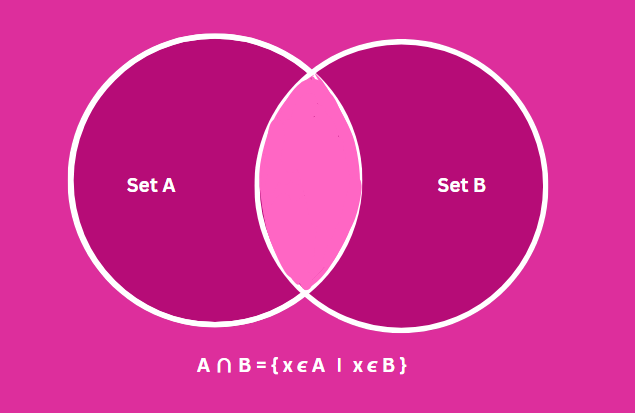
Mathematical Notation: A ∩ B = { x∊A ∣ x∊B }
Syntax:
function intersection(setA, setB) {
return [...new Set([...setA].filter(elem => setB.has(elem)))];
}
Parameters: We will input two sets as parameters to our function.
Return: Our function will return an array containing common elements from both sets.
Example:
function intersection(setA, setB) {
return [...new Set([...setA].filter(elem => setB.has(elem)))];
}
const A = new Set([1, 2, 3, 4]);
const B = new Set([3, 4, 5, 6]);
console.log("The intersection set of A and B: " + intersection(A, B));
Output:

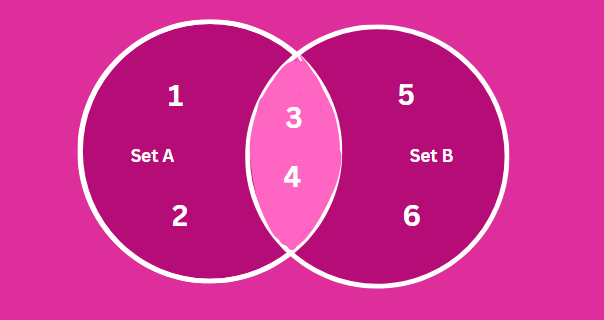
4. Difference
Description: The difference set is a set with elements that are in one set but not in the other.
Venn Diagram:
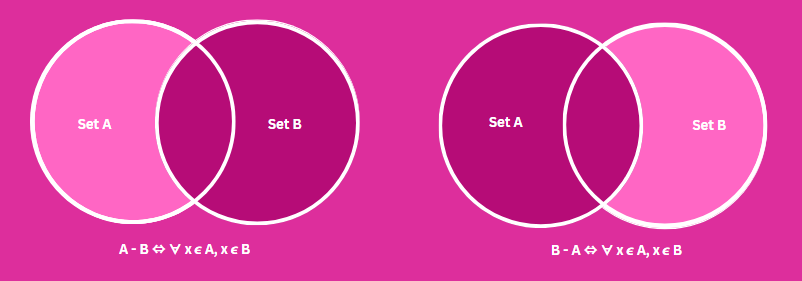
Mathematical Notation: A – B ⇔ ∀ x∊A, x∊B
Syntax:
function difference(setA, setB) {
return [...new Set([...setA].filter(elem => !setB.has(elem)))];
}
Parameters: We will input two sets as parameters to our function.
Return: It will return elements present in the first set that are not present in the second set.
Example:
function difference(setA, setB) {
return [...new Set([...setA].filter(elem => !setB.has(elem)))];
}
const A = new Set([1, 2, 3, 4]);
const B = new Set([3, 4, 5, 6]);
console.log("The subtraction of set A from set B: " + difference(A, B));
console.log("The subtraction of set B from set A: " + difference(B, A));
Output:

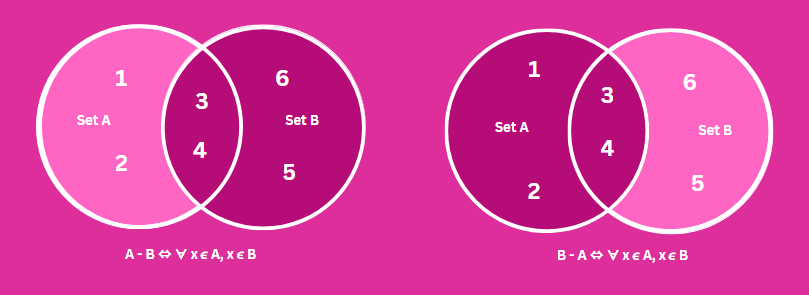
Conclusion
Sets are useful in JavaScript because they only hold unique values with no duplicates. They can be very useful if you want to maintain a collection of items where each item is unique. For example, if you are maintaining a list of users who have logged in, then a set will enable you to treat each user only once, even when the user logs in several times.
Sets, however, are quite efficient for checking whether an item is in the collection, adding new items, or removing items. Maps in JavaScript also work similarly, click here to learn about this.
Are you curious to know about Typescript? Well, then click here to explore!
Reference
https://stackoverflow.com/questions/8363564/ways-to-create-a-set-in-javascript