In Node.js, the File System module provides various methods for interacting with files. We can use these methods for removing files as well. Here are some use cases where we might need to remove files:
- Temporary files cleanup: When our application creates temporary files for processing data or caching purposes, we might want to periodically remove these temporary files to free up disk space and avoid cluttering.
- File uploads management: If our application allows users to upload files, we may need to remove the uploaded files after processing or when they are no longer needed.
- Cache management: When implementing caching mechanisms in our application, we may store cached data in files. Removing expired or unnecessary cache files helps maintain an efficient caching system.
There are two ways to remove files in Node.js: Synchronous and Asynchronous. In this article, we will show you both ways with a step-by-step approach. So let’s get started.
Synchronous Way of Removing a File in Node.js
Synchronous means blocking. For example, if there are LINE 1 and LINE 2, and LINE 1 is synchronous, then LINE 2 will execute only after LINE 1 has finished executing.
Step1: Importing File System Module
For utilizing the methods of the File System Module we first need to import it.
Syntax of importing the fs module:
cosnt fs = require('fs');
Above we have used require() function for requiring or importing the fs module and then stored it into a constant fs by which we can call any of the fs module methods.
Step 2: Writing a File Using the fs.writeFileSync() Function
For writing a file synchronously we have to use the fs.writeFileSync() function.
Syntax of writing a file synchronously:
fs.writeFileSync(path, content);
where:
- path is the file name with the directory where we want to write data,
- content is the data that is to be present inside the file.
Example:
const fs = require('fs');
const dirPath = "./file-repo/";
if (fs.existsSync(dirPath)) {
fs.writeFileSync(dirPath + "/writeFile.txt", "QA BOX");
} else {
console.log(`${dirPath} not found`);
}
Here, we have first imported the fs module in the constant fs. Then created a constant called dirPath which contains the path for the folder file-repo where we want to create a file.
After that, we implemented the fs.existsSync() function which gives a boolean value and is also one of the fs module methods to check whether a directory exists or not.
We have also used the if/else block for control flow and if the dirPath exists then only the fs.writeFileSync() function writes the file. We have passed two parameters inside the fs.writeFileSync() method, first is the path which is dirPath+”/writeFile.txt” and the second parameter is “QA BOX” which is content to be written inside the file writeFile.txt. If the path does not exist then it should go into the else but in this case, it exists so in the file-repo folder the writeFile.txt file will be created with the content QA BOX.
Output:
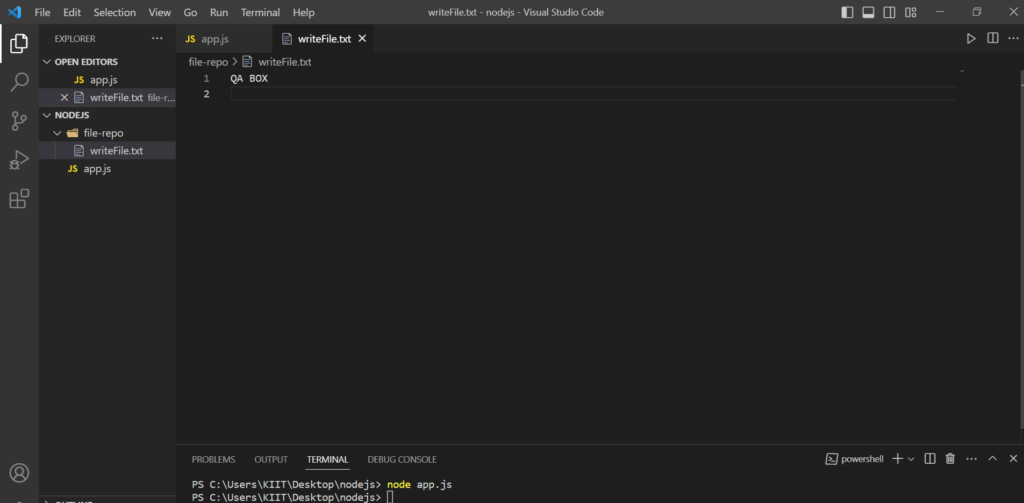
Step 3: Removing a File Using the fs.unlinkSync() Function
To synchronously delete a file, we need to use the fs.unlinkSync() function.
Syntax of removing a file synchronously:
fs.unlinkSync(Path);
where:
- path is the file name with the directory from where we want to remove the file.
Example:
const fs = require('fs');
const dirPath = "./file-repo/";
if (fs.existsSync(dirPath)) {
fs.unlinkSync(dirPath + "/writeFile.txt");
} else {
console.log(`File not found`);
}
Here for deleting the file we have used the fs.unlinkSync() function in which we have passed a path. Also, we have implemented the if/else block and fs.existsSync() function to perform operations based on whether the dirPath exists or not.
Output:
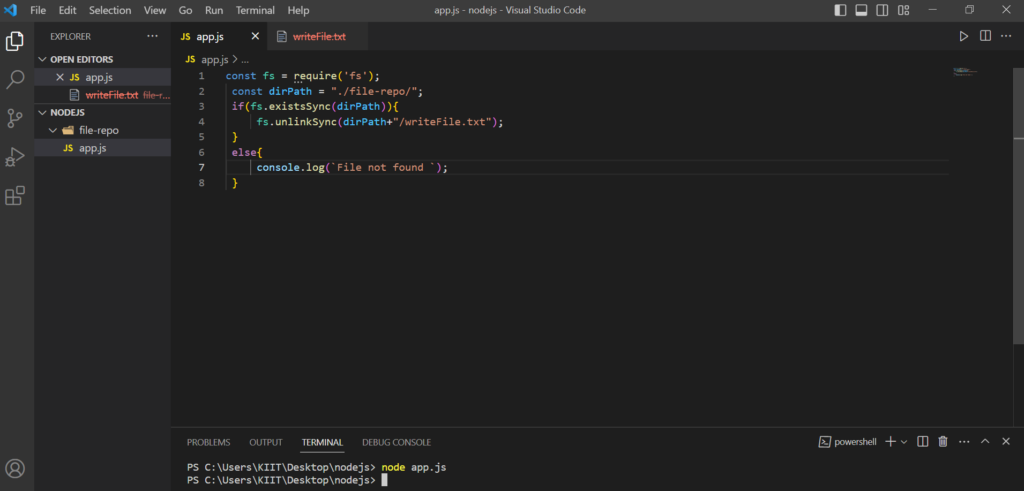
Asynchronous Way of Removing a File in Node.js
Asynchronous means non-blocking. For example, if there are LINE 1 and LINE 2, and LINE 1 is asynchronous then LINE 2 will start executing immediately, which means it will not wait for LINE 1 to complete its execution.
Step1: Importing File System Module
Like the synchronous way, we need to import the fs module here as well.
Syntax of importing the fs module:
cosnt fs = require('fs');
Above we have imported the fs module inside the constant fs in the same way that we have done in the synchronous way.
Step 2: Writing a File Using the fs.writeFile() Function
For writing a file asynchronously we have to use the fs.writeFile() function.
Syntax of writing a file asynchronously:
fs.writeFile(Path,Content,Callback);
where:
- path is the file name with the directory which we want to write,
- content is the data or text which we want to write,
- callback is the function that is called after the file is written or if the error occurs.
Example:
const fs = require('fs');
const dirPath = "./file-repo/";
fs.writeFile(dirPath + "/writeFile.txt", "Test 123", (err) => {
if (err) {
console.log(err.message);
} else {
console.log("Data is Saved");
}
});
Here we have imported the fs module into the constant fs and then we stored the path of folder file-repo into the constant dirPath.
After that, we used the fs.writeFile() function for writing the file asynchronously. In this function, there are three parameters first parameter is a path which is dirPath +”/writeFile.txt”, the second parameter is the content which is “Test 123” and the third parameter is the callback.
Output:
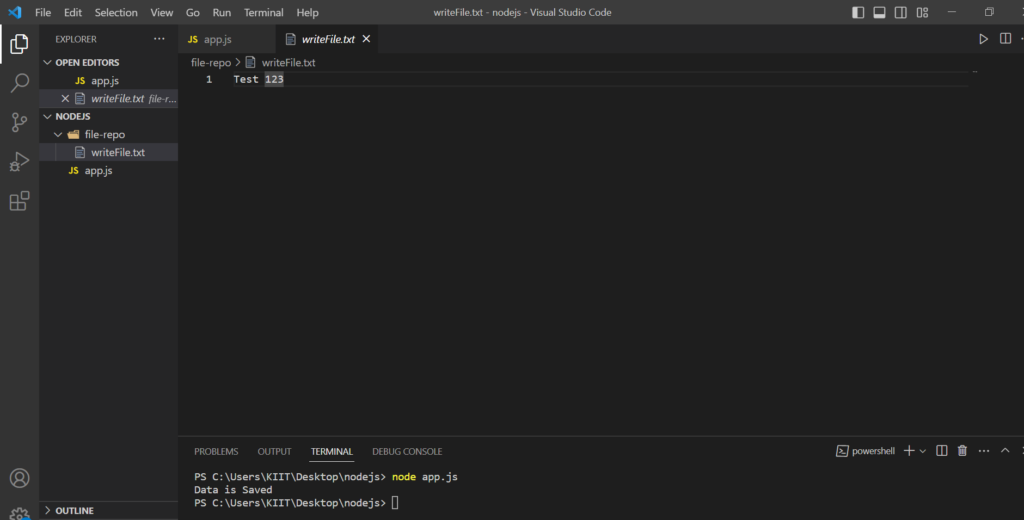
Step 3: Removing a File Using the fs.unlink() Function
For removing a file Asynchronously we have to use the fs.unlink() function.
Syntax of removing a file asynchronously:
fs.unlink(Path,Callback);
where:
- path is the file name with the directory from where we want to remove the file,
- callback is the function that is called after the file is written or if the error occurs.
Example:
const fs = require('fs');
const dirPath = "./file-repo/";
fs.unlink(dirPath + "/writeFile.txt", (err) => {
if (err) {
console.log(err.message);
} else {
console.log("File is Deleted");
}
});
Here we have used the fs.unlink() function and provided a path dirPath+”/writeFile.txt” with a callback to handle the error or print a success message, after executing the above code the file “writeFile. txt” will be deleted.
Output:
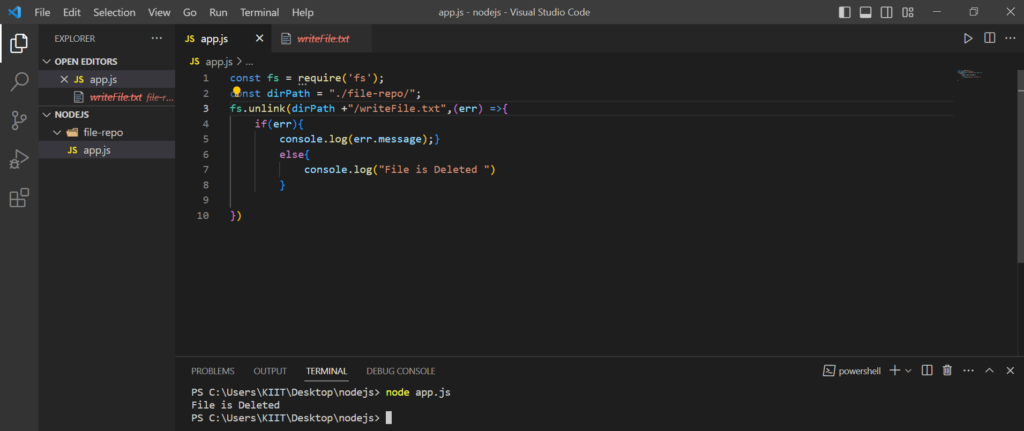
Summary
In this article, we have learned about the synchronous and asynchronous methods of writing and deleting a file from a folder. We first explored how to import the fs module and then how to write a file, and then finally, we looked at how to delete a file. After reading this article, we hope you can easily delete a file from a folder in Node.js.
Reference
https://nodejs.org/api/fs.html#file-system