In web applications, tables are often used to display data, like student grades, product lists, or user information. A key feature that enhances user experience is the ability to easily add or remove rows. This is important because users may want to update their data, remove mistakes, or simply clean up their tables. In this article, we will explore two easy methods to delete rows from an HTML table: the deleteRow() method and direct DOM manipulation.
We have already discussed how to delete all the rows in a table in our previous article, click here to read.
HTML Table Structure
Before we begin, let’s briefly review how an HTML table is structured. A basic table consists of:
- <table>: The container for the table.
- <tr>: Each row in the table.
- <th>: Header cells, which usually contain column titles.
- <td>: Data cells, which contain the actual data.
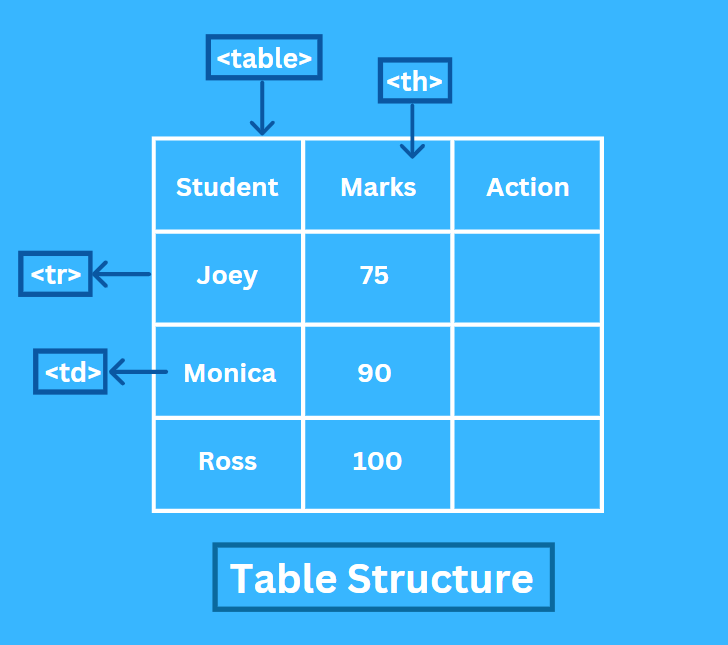
Here is a sample table on which we will perform the deletion. It contains three rows with column headings ‘Student’ and ‘Marks’, along with a remove button in each row to demonstrate single-row deletion.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Styled Table</title>
<style>
#myTable {
width: 50%;
border-collapse: collapse;
margin: 20px auto;
outline: 2px solid rgb(39, 89, 255);
font-family: Arial, sans-serif;
}
#myTable th {
background-color: #45c8ec;
padding: 12px;
text-align: left;
border-bottom: 1px solid #ddd;
}
#myTable td {
padding: 12px;
border-bottom: 1px solid #ddd;
}
#myTable tr:nth-child(even) {
background-color: #45c8ec;
}
</style>
</head>
<body>
<table id="myTable" border="1">
<tr>
<th>Student</th>
<th>Marks</th>
<th>Action</th>
</tr>
<tr>
<td>Joey</td>
<td>75</td>
<td><button onclick="removeRow(this)">Remove</button></td>
</tr>
<tr>
<td>Monica</td>
<td>90</td>
<td><button onclick="removeRow(this)">Remove</button></td>
</tr>
<tr>
<td>Ross</td>
<td>100</td>
<td><button onclick="removeRow(this)">Remove</button></td>
</tr>
</table>
</body>
</html>
Output:
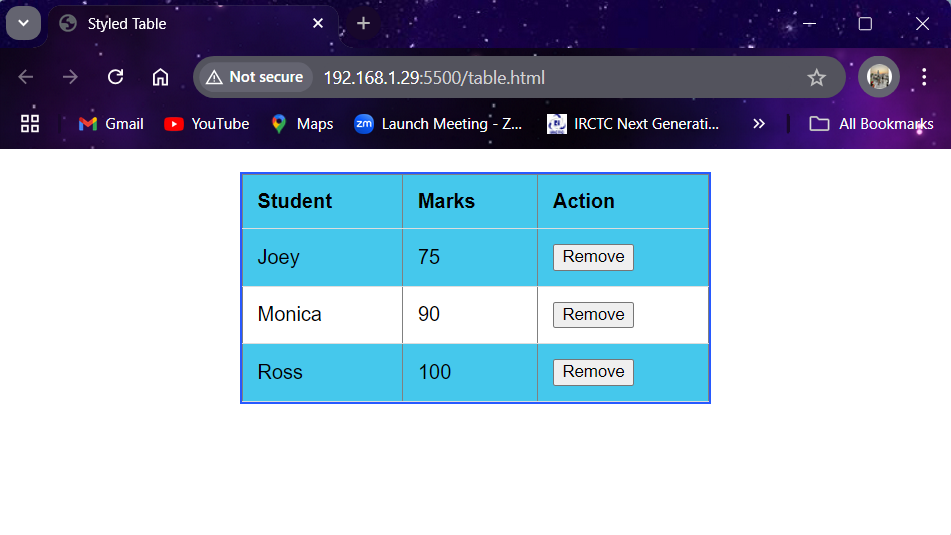
Methods to Remove a Row from a Table
There are two easy ways by which we can perform single-row deletion: the deleteRow() method and DOM manipulation.
The deleteRow() Method
The deleteRow() method is a simple way to remove a row from a table. It’s part of the HTMLTableElement interface and lets you delete a row by specifying its index.
First, we use document.getElementById() to access the table by its ID. Then, we find the row index of the row to be deleted using the button parent elements. Finally, we call the deleteRow() method on the table and pass in the row index to remove that specific row.
Here is the Javascript logic we apply to our sample table for row deletion:
<script>
function removeRow(button) {
var row = button.parentNode.parentNode;
var table = document.getElementById("myTable");
table.deleteRow(row.rowIndex);
}
</script>
Explanation:
- removeRow() function is called when the “Remove” button is clicked.
- It uses the button parameter to get the row (tr) that contains the button by accessing its parentNode.parentNode.
- It then gets the table by its ID (myTable) and deletes the specific row using the deleteRow() method, passing the row’s index (row.rowIndex).
Output:
Advantages:
- It is explicitly designed for manipulating table rows.
- It automatically handles the row indexing.
Disadvantages:
- It requires to know the row index.
DOM Manipulation
In this method, we can remove the row directly by manipulating the DOM. This method is flexible, as you can remove any element without the requirement of its index.
This is the code we use in our sample table for row deletion:
function removeRow(button) {
var row = button.parentNode.parentNode;
row.remove();
}
Explanation:
- removeRow() function is called when the “Remove” button is clicked.
- It identifies the row (tr) containing the clicked button using button.parentNode.parentNode.
- The remove() method is then called directly on the row element, which completely removes it from the DOM.
Output:
Advantages:
- It is more flexible as it works with all HTML elements, not just tables.
- It directly removes the element without requiring its index.
Conclusion
Single-row deletion in JavaScript makes it easy to update tables, enabling the quick removal of errors and improving user experience. It keeps the page interactive without needing a full reload. Both methods effectively allow you to remove a row from a table in JavaScript. The choice between deleteRow() and direct DOM manipulation depends on your specific use case and personal preference.
Do you know what javascript:void(0) means? Click here to find out!