React is a popular framework by Facebook. React is known as an effective and adaptable JavaScript library for creating user interfaces.
In this article, we will use ReactNode and discuss its features and implementation. Our objective is to provide a structured and thorough analysis of ReactNode, moving our spotlight on its specifics and application within the React ecosystem with TypeScript.
Also Read – NodeJS vs ReactJS: What are the similarities and differences?
Introduction to ReactNode
ReactNode is a versatile TypeScript type that provides a union type for many renderable elements inside React. It includes a huge range of types, including JSX elements, null, boolean, string, integer, and undefined. Because of its inclusiveness, it is a great option for creating React components that can handle and display different kinds of material. ReactNode offers a simple method of managing the dynamic content of the React application or section of JSX.
Example of ReactNode:
import React, { ReactNode } from 'react';
function Example(props: { children: ReactNode }) {
return <div>{props.children}</div>;
}
Here, the Example component is a simple and versatile component that may render any material that is supplied to it as one of its children. It supports a wide range of content kinds by utilizing the ReactNode type.
Using ReactNode with TypeScript
Let’s examine a few typical situations and examples to show how ReactNode may be used in real-world situations with TypeScript.
1. Using ReactNode for Dynamic Content Rendering
ReactNode can be very helpful when working with components that need to render dynamic content.
Example:
const Text = "Hello, ReactNode!";
const Component_HTML = <span>This is dynamic content.</span>;
const Example1: React.FC<{ content: React.ReactNode }> = ({ content }) => {
return <div>{content}</div>;
};
<Example1 content={Text} />;
<Example1 content={Component_HTML} />;
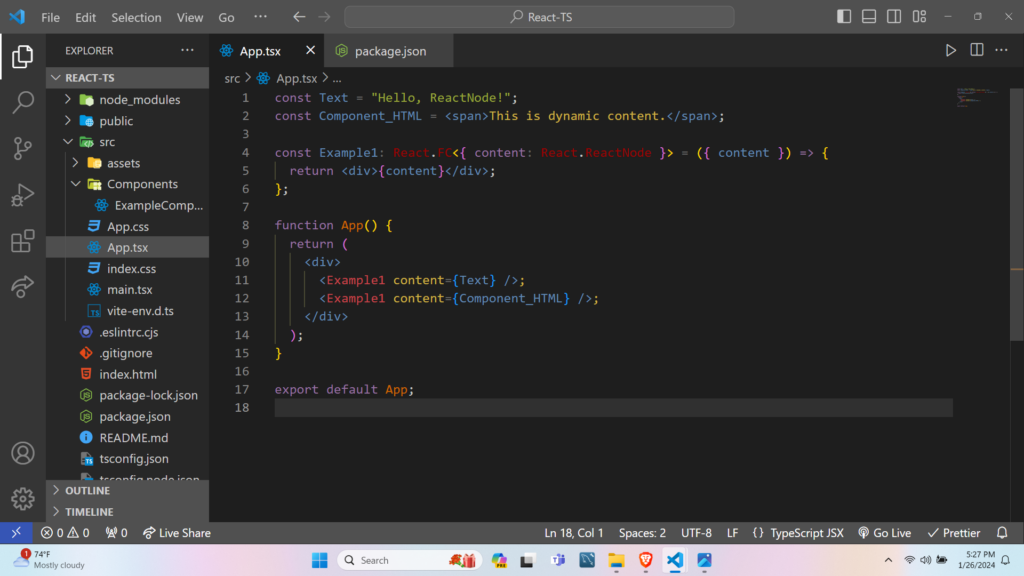
In this example “Example1” is a React functional component designed to accept and render any content of type ReactNode through the “content” prop.
Here we have shown two cases to show dynamic rendering where “Text” is a string, and “Component_HTML” is a JSX element. With these design choices, developers may dynamically supply data to “Example1,” making it able to handle many use cases in a React application.
Output:
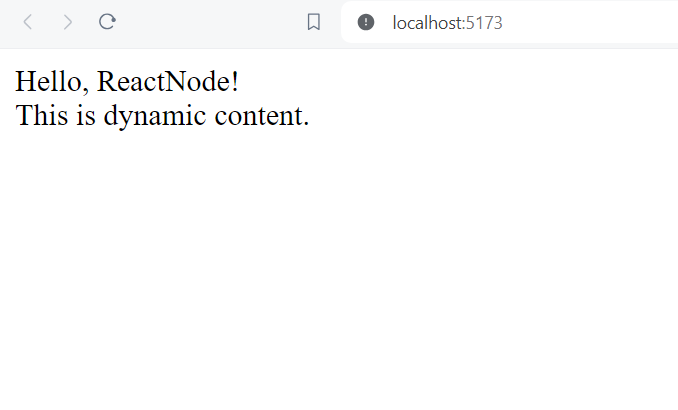
2. Using ReactNode for Conditional Rendering
ReactNode provides a simple solution for components that will render content on the basics of conditions depending on specific criteria. This is helpful in situations when components may display various information in response to user interaction.
Example:
const Example2: React.FC<{ condition: boolean; content: React.ReactNode }> = ({ condition, content }) => {
return condition ? <div>{content}</div> : <div>Condition is false</div>
};
<Example2 condition={true} content="Rendered when condition is true" />;
<Example2 condition={false} content="Not rendered when condition is false" />;
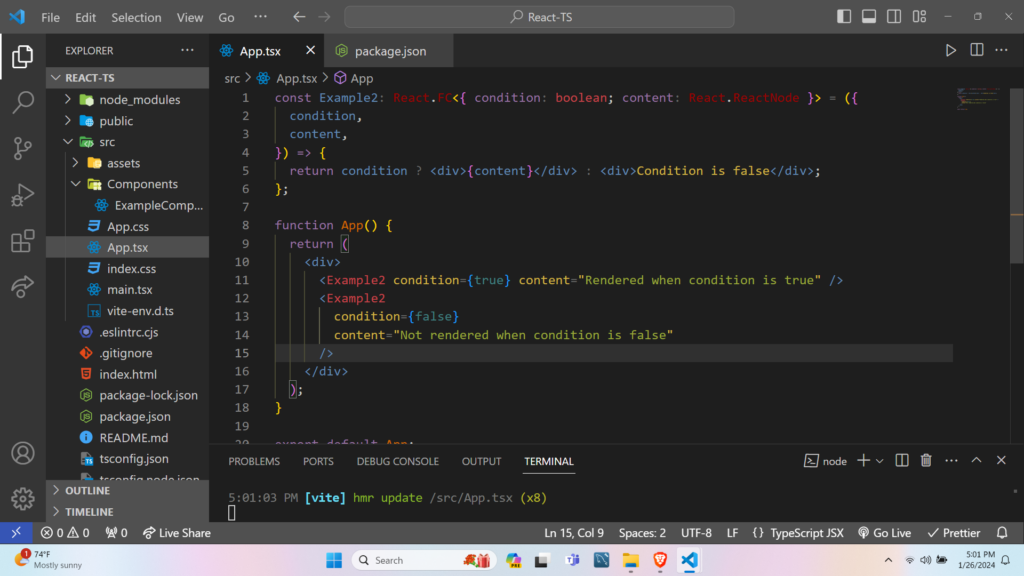
In this example, “Example2” is a React functional component designed to conditionally render content based on a boolean “condition” prop.
We have discussed two cases when the condition is true, the <div> with the specified content will be rendered. When the condition is false, “Condition is false” will render.
Output:
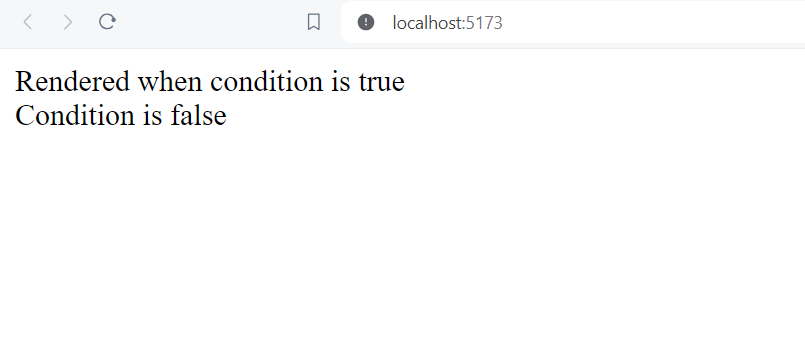
3. Using ReactNode for Combining Different Content Types
We can also use ReactNode for combining different content types.
Example:
const Example3: React.FC<{ header: React.ReactNode; body: React.ReactNode }> = ({ header, body }) => {
return (
<div>
<h2>{header}</h2>
<div>{body}</div>
</div>
);
};
<Example3 header="Welcome!" body={<p>This is the body of the component.</p>} />;
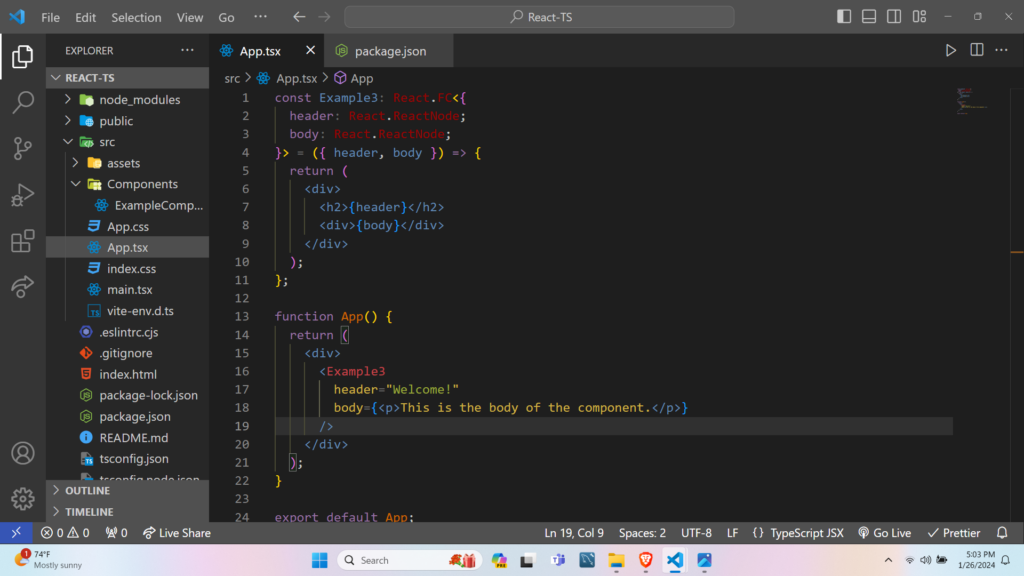
“Example3″ is a functional component that takes props with two properties: header and body, both of type ReactNode. It renders a <div> containing an <h2> element with the specified header and a <div> element with the specified body.
Output:
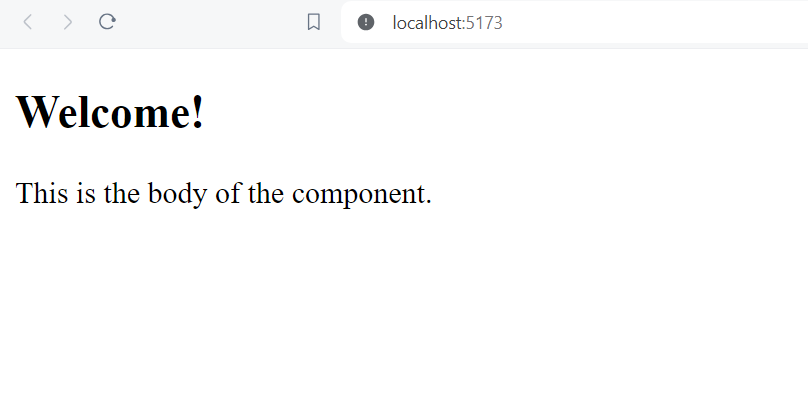
Mistakes to Avoid While Using ReactNode in TypeScript
While working with ReactNode in TypeScript, developers may encounter certain mistakes that if not addressed can lead to issues in the application.
Let’s explore some of these mistakes and provide insights on how to avoid them:
- Proper JSX: Using ReactNode improperly in JSX, such as directly rendering it without wrapping it in a valid JSX element. To prevent these from happening we should wrap it in a fragment (<> … </>).
- Return Type Mismatch: Runtime problems and type mismatches can occur when an incorrect type is assigned to a ReactNode prop. We can avoid this by assigning the expected type of ReactNode.
- Null and Undefined: Passing null or undefined when working with ReactNode can lead to unexpected behaviour and runtime errors. To prevent we should always handle these cases carefully and implement validation.
- Overusing any Type: Using any as type might provide flexibility but can diminish the advantages that TypeScript’s static typing increasing the risk of runtime mistakes and complicating code maintenance.
Read More: Why React Matters
Conclusion
In conclusion, ReactNode is a useful and essential tool for handling dynamic content in React components. By combining TypeScript features like type annotations and interfaces with ReactNode, developers can enhance code readability, spot issues as they arise, and maintain robust typing.
Reference
https://stackoverflow.com/questions/58123398/when-to-use-jsx-element-vs-reactnode-vs-reactelement